How to Use Lambda Functions With the for Loop in Python
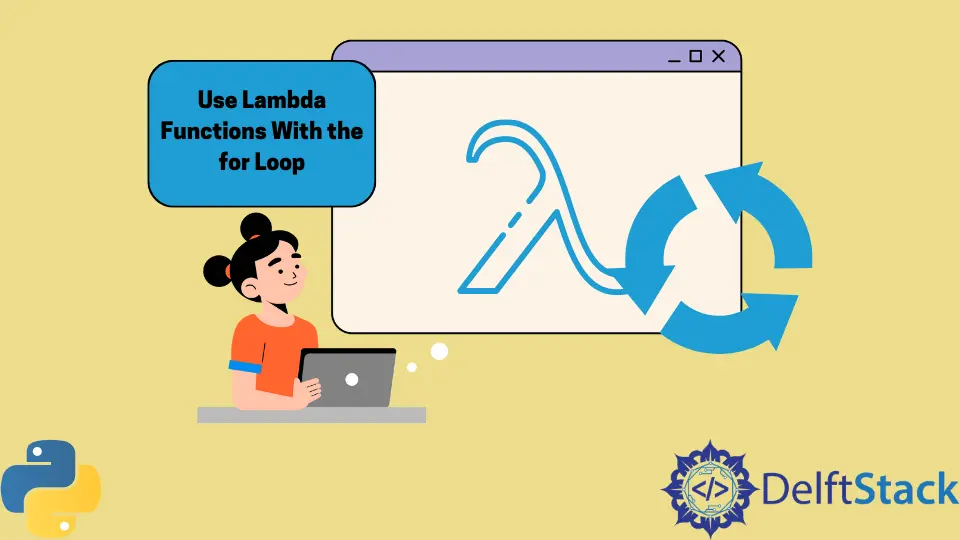
Lambda functions or anonymous functions are Python functions without a name. These functions are created through the use of the lambda keyword as opposed to the def keyword that is traditionally used to define normal functions in Python.
Lambda functions are not only smaller as compared to normal functions, but they also have several differences. Most significant is the absence of an explicitly defined return statement and the restriction to only one expression.
Nevertheless, lambda functions accept multiple arguments just like the normal Python functions.
In Python, Lambda functions can be implemented as shown below.
lambda arguments: expressions:
Lambda functions are used when there is a need to create a function object used as an argument in another function.
The power of Lambda is particularly evident when used alongside higher-order functions such as map()
. These functions accept other function objects as arguments.
In addition, lambda functions can also be used to perform operations that require anonymous functions only for a short time. A good example of this would be using lambda functions alongside [a for
loop]({{relref “/HowTo/Python/one line for loop python.en.md”}}) to perform a certain operation on all the elements of an iterable.
The program below illustrates how we can use a for loop to iterate over a list of numbers, and a lambda function is used as an incrementer in this case.
nums1 = [45, 46, 47, 48, 50]
nums2 = []
for i in nums1:
def x(i):
return i + 1
nums2.append(x(i))
print(nums2)
Output:
[46, 47, 48, 49, 51]
For loops can be used to iterate over other sequences such as tuples, strings, dictionaries, and sets. Although lambda functions can only have one expression, there are no restrictions on the data types that can be used.
The example below illustrates how we can iterate over a list of strings using a for loop and a lambda function.
fruits = ["mango", "apple", "melon", "pineapple"]
fruits_upper = []
for fruit in fruits:
def x(fruit):
return fruit.upper()
fruits_upper.append(x(fruit))
print(fruits_upper)
Output:
['MANGO', 'APPLE', 'MELON', 'PINEAPPLE']
The Lambda function can be used together with a for loop to create a list of lambda objects. Using these objects, we can perform actions on elements of an iterable using a for loop.
This is beneficial, especially when the intention is to iterate over an iterable object while making changes simultaneously without getting a Runtime error.
list = [1, 2, 3, 4, 5]
def add_two(x):
return lambda: x + 2
list = [add_two(i) for i in list]
for element in list:
print(element())
Output:
3
4
5
6
7
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn