How to Ignore an Exception in Python
-
Use the
pass
Statement in theexcept
Block in Python -
Use the
sys.exc_clear()
Statement in theexcept
Block in Python - Conclusion
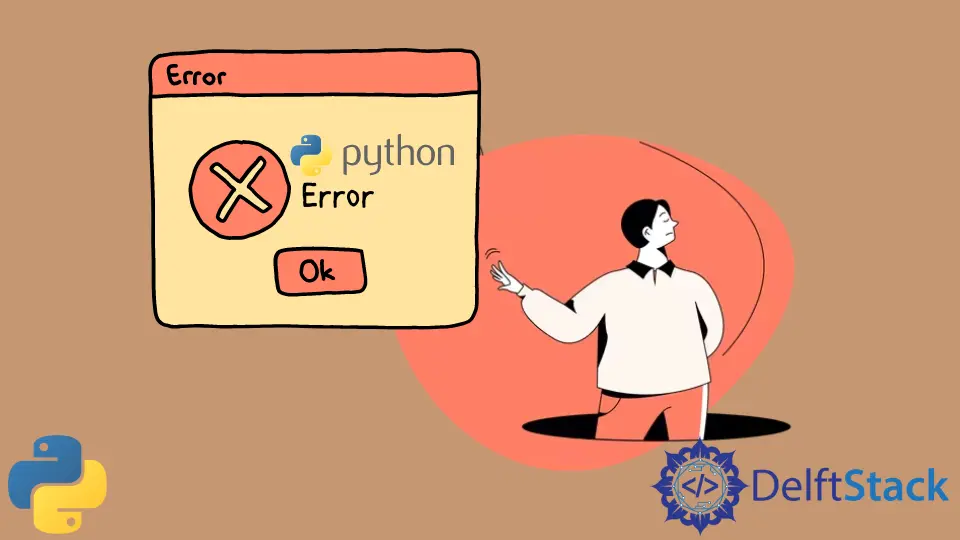
An exception is an event that, when raised, alters the flow of the program.
Exceptions result from the program being syntactically correct but still giving an error on the execution of the code. This error does not alter the program’s execution but rather changes the default flow of the program.
In Python, we handle exceptions using the try...except
block. This tutorial will discuss several methods to ignore an exception and proceed with the code in Python.
Use the pass
Statement in the except
Block in Python
The pass
statement can be considered as a placeholder in Python programming. It returns a NULL
statement and, therefore, produces no value. However, the Python interpreter does not ignore the pass statement, and we prevent getting errors for empty code where the statement is used.
When the pass
statement is used in the try...except
statements, it simply passes any errors and does not alter the flow of the Python program.
The following code uses the pass
statement in the except
block to ignore an exception and proceed with the code in Python.
try:
print(hey)
except Exception:
pass
print("ignored the exception")
As we navigate through the code, let’s comprehend the flow of the try-except
block with the pass
method.
Inside the try
block, we attempt to print the value of the variable hey
, which is not defined. This results in a NameError
.
This block catches any exception of type Exception
, which is a broad category covering most standard exceptions.
Instead of specifying explicit actions to take in response to the exception, we use the pass
statement. This signals that we acknowledge the exception without executing any specific code in response.
Following the try-except
block, we have a print
statement that will be executed regardless of whether an exception occurred or not.
Output:
ignored the exception
Use the sys.exc_clear()
Statement in the except
Block in Python
In Python 2, the last thrown exception gets remembered by the interpreter, while it does not happen in the newer versions of Python. Therefore, the sys.exc_clear()
statement is not needed in the versions released after Python 3.
The sys.exc_clear()
statement can be utilized to clear the last thrown exception of the Python interpreter.
The following code uses the sys.exc_clear()
statement in the except
block to ignore an exception and proceed with the code in Python.
import sys
try:
print(hey)
except Exception:
sys.exc_clear()
print("ignored the exception")
As we delve into the code, let’s navigate through the logical flow of the try-except
block with the sys.exc_clear()
method.
Inside the try
block, we attempt to print the value of the variable hey
, which is not defined. This results in a NameError
.
This block catches any exception of type Exception
, which is a broad category covering most standard exceptions.
Instead of using the pass
statement, as seen in other examples, we employ the sys.exc_clear()
method to clear the exception information.
Following the try-except
block, we have a print
statement that will be executed regardless of whether an exception occurred or not.
Output:
ignored the exception
Conclusion
The pass
statement emerges as a concise and modern approach to ignore exceptions in Python. It acts as a placeholder, allowing the program to gracefully handle errors without disrupting the code’s readability.
When used in the except
block, it clearly signifies the intention to acknowledge the exception without executing any specific actions.
On the other hand, the deprecated sys.exc_clear()
method, designed for Python 2, is no longer relevant in newer Python versions.
In modern Python programming, the pass
statement stands out as a recommended and clear choice for ignoring exceptions.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn