How to Use getitem in Python
-
Basic Understanding of
__getitem__()
-
Implementing
__getitem__()
in Custom Classes: - Understanding Key Types:
-
Support Slicing in
__getitem()__
-
Error Handling When Using
__getitem__()
For Custom Objects
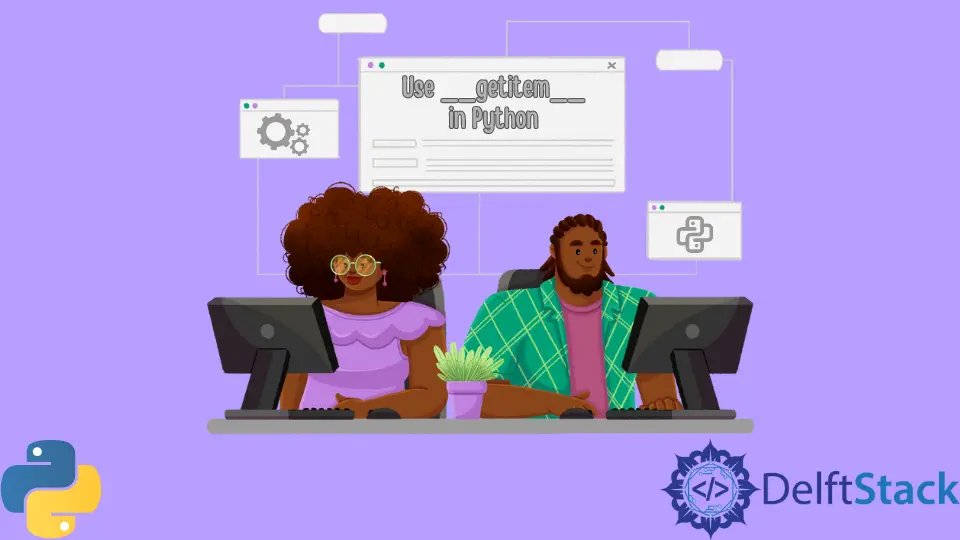
The __getitem__()
method in Python is a special method, often referred to as a magic
or dunder
method, that allows an object to support indexing. This method is a part of Python’s data model and is invoked when the []
indexing syntax is used on an object. By implementing the __getitem__()
method in your classes, you can allow instances of your classes to be indexed just like lists or dictionaries.
The importance of the __getitem__()
method cannot be overstated as it forms the foundation for accessing elements in various built-in data types, and can be leveraged to create custom data structures that support indexing. Common use cases of __getitem__()
include:
- Creating array-like structures that support element access via indexing.
- Building dictionary-like objects that allow key-value retrieval.
- Enhancing classes to support slicing operations.
- Designing proxy objects that delegate indexing to other objects.
This method provides a way to build intuitive and clean interfaces for custom data structures, thereby enhancing code readability and ease of use.
Basic Understanding of __getitem__()
The __getitem__()
method is at the heart of how built-in types like lists and dictionaries allow element access. Let’s delve into simple examples to understand its basic usage:
Lists:
my_list = [10, 20, 30, 40, 50]
# Accessing the third item (index 2) in the list
item = my_list[2]
print(item)
Output:
30
In this example, when we use the indexing syntax my_list[2]
, Python internally calls the __getitem__()
method of the list object to retrieve the element at index 2.
my_list = [10, 20, 30, 40, 50]
print(my_list.__getitem__(2))
Dictionaries:
my_dict = {"Python": "A programming language", "Pi": 3.14}
# Accessing the value associated with the key "Python"
value = my_dict["Python"]
print(value)
Output:
A programming language
Similar to lists, when we access a value in a dictionary using a key, the __getitem__()
method is invoked to fetch the associated value.
my_dict = {"Python": "A programming language", "Pi": 3.14}
print(my_dict.__getitem__("Python"))
Implementing __getitem__()
in Custom Classes:
Implementing the __getitem__()
method in your custom classes is straightforward, yet immensely powerful. It allows your objects to support indexing operations. Here’s an example to illustrate this:
class CustomList:
def __init__(self, elements):
self.elements = elements
def __getitem__(self, index):
return self.elements[index]
# Usage:
my_list = CustomList([10, 20, 30, 40, 50])
element = my_list[2]
print(element)
In this CustomList
class, we’ve defined a __getitem__()
method that takes an index as an argument and returns the element at that index from the elements
list. This enables instances of CustomList
to be indexed using the square bracket ([]
) notation, just like built-in lists.
Understanding Key Types:
The __getitem__()
method is not limited to handling integer indices; it can also work with slices, strings, or even custom objects. This flexibility allows for a rich variety of behaviors.
Integer Indices and Slices:
class CustomSequence:
def __getitem__(self, key):
if isinstance(key, int):
return f"Element at index {key}"
elif isinstance(key, slice):
return f"Slice from {key.start} to {key.stop} with step {key.step}"
# Usage:
seq = CustomSequence()
print(seq[5])
print(seq[1:10:2])
Output:
Element at index 5
Slice from 1 to 10 with step 2
Custom Objects as Keys:
class CustomKey:
def __init__(self, key):
self.key = key
class CustomDictionary:
def __getitem__(self, custom_key):
return f"Value associated with key {custom_key.key}"
# Usage:
custom_dict = CustomDictionary()
key = CustomKey("hello")
value = custom_dict[key]
print(value)
Output:
Value associated with key hello
These examples demonstrate the flexibility of __getitem__()
, showing how it can be adapted to handle a variety of key types to cater to different use cases.
Support Slicing in __getitem()__
Slicing is a powerful feature in Python that allows for extracting portions of a sequence like a list or string. The __getitem__()
method can be tailored to support slicing operations within custom objects, enabling a more versatile and Pythonic interface. This session delves into how slicing can be implemented and interacted with through the __getitem__()
method.
Implementing Slicing Support in __getitem__()
:
To support slicing, the __getitem__()
method needs to recognize when it receives a slice object rather than a simple integer index. A slice object contains start, stop, and step attributes, which represent the beginning, end, and step values of the slice, respectively. Here’s an example of how to implement slicing support in a custom sequence object:
class CustomSequence:
def __init__(self, data):
self.data = data
def __getitem__(self, key):
if isinstance(key, slice):
start, stop, step = key.indices(len(self.data))
return [self.data[i] for i in range(start, stop, step)]
elif isinstance(key, int):
return self.data[key]
else:
raise TypeError(f"Invalid argument type: {type(key)}")
# Usage:
seq = CustomSequence([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
print(seq[2:7:2])
Output:
[2, 4, 6]
In this CustomSequence
class, the __getitem__()
method checks if the key is a slice object using isinstance()
. If it’s a slice, it calculates the actual indices using the indices()
method of the slice object and then constructs and returns a new list containing the sliced elements.
Explaining How Slicing Interacts with __getitem__()
:
The interaction between slicing and the __getitem__()
method is facilitated by the slice object. When a slice operation is performed on an object, a slice object is created and passed to the __getitem__()
method of the object. This slice object encapsulates the slicing parameters (start, stop, and step), allowing __getitem__()
to understand and perform the requested slice operation.
In the example above, when seq[2:7:2]
is executed, a slice object with start = 2, stop = 7, and step = 2 is created and passed to __getitem__()
. The __getitem__()
method then uses this information to extract and return the required elements from the data
list.
This mechanism enables seamless integration of slicing operations in custom objects, making them behave in a manner similar to built-in sequence types like lists and strings. By handling slice objects appropriately within the __getitem__()
method, you can provide a rich, intuitive, and Pythonic interface for interacting with your custom objects.
Supporting slicing in your custom objects not only enhances their functionality but also provides a familiar and intuitive interface for other developers, making your classes more usable and idiomatic in the broader context of the Python programming environment.
Error Handling When Using __getitem__()
For Custom Objects
Implementing error handling in your __getitem__()
method is crucial to provide clear and informative error messages to the users of your class.
class SafeList:
def __init__(self, elements):
self.elements = elements
def __getitem__(self, index):
try:
return self.elements[index]
except IndexError:
return f"Index {index} out of range!"
except TypeError:
return "Invalid index type!"
# Usage:
safe_list = SafeList([10, 20, 30, 40, 50])
print(safe_list[60])
print(safe_list["hello"])
In this SafeList
class, we’ve added error handling to the __getitem__()
method to catch IndexError
and TypeError
exceptions, providing clear error messages that inform the user of the issue. This makes your class more robust and user-friendly, especially when used in larger or more complex codebases.
Output:
[2, 4, 6]
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn