How to Get Yesterday's Date in Python
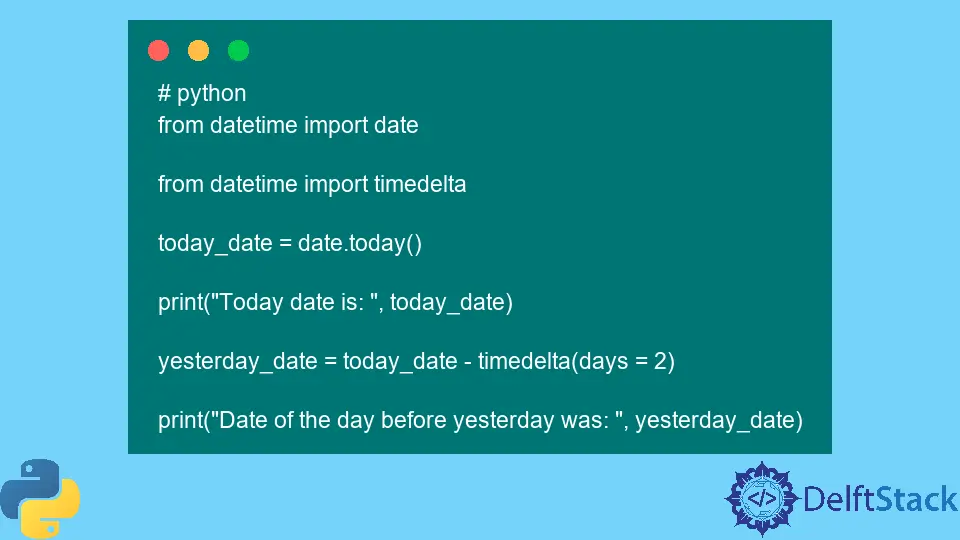
We will introduce how we can get yesterday’s date using Python with multiple examples.
Get Yesterday’s Date in Python
Python is a high-level language that can be used in Data Science and Machine learning to train the AI using Python’s data structures. It is used to program various applications, from web and desktop to operating system-level programs.
It is a built-in simple and easy-to-use syntax that makes it easy to understand and learn for new programmers. This article will study how to get yesterday’s date in Python.
When we are working on large-scale applications, we may need to get the data from the previous date, and it can be a lengthy process; we may have to access the database or make some additional features to get it.
But we will automate it with a function that will take in the value of the date and return the date one day earlier.
Let’s discuss some easy methods to find yesterday’s date, which is easily understandable. The Python modules datetime
and timedelta
work with date and time.
Date Module in Python
First, we need to install the module using the following command.
# python
pip install datetime
Once we have installed the datetime
module, we can get today’s date using the simple method .today()
. We need to import the date
from our module and use the .today()
method.
# python
from datetime import date
today = date.today()
Now we will install the timedelta
module using the following command.
pip install timedelta
Once our module is installed now, we will import timedelta
. We can use this module to represent an interval of time that can be positive or negative.
In simple words, we can use this module to find the difference between seconds, minutes, hours, or days after a certain date. We normally use it for date manipulation.
Now we will discuss some of the examples to find the yesterday date by using programs and see their implementations in programming.
Examples to Get Yesterday’s Date in Python
Our first example is a simple program that uses the date and time module to display the current date and the date and time of the previous day, as shown below.
# python
from datetime import date
from datetime import timedelta
today_date = date.today()
print("Today date is: ", today_date)
yesterday_date = today_date - timedelta(days=1)
print("Yesterday date was: ", yesterday_date)
Output:
Now let’s take another example: a simple program that uses the date and time module to display the date and time of the current date and the date of the one day before the previous day.
# python
from datetime import date
from datetime import timedelta
today_date = date.today()
print("Today date is: ", today_date)
yesterday_date = today_date - timedelta(days=2)
print("Date of the day before yesterday was: ", yesterday_date)
Output:
The above example shows that using the date
. We can get yesterday’s date or the date before yesterday using the simple module timedelta
.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn