How to Get Path of the Current File in Python
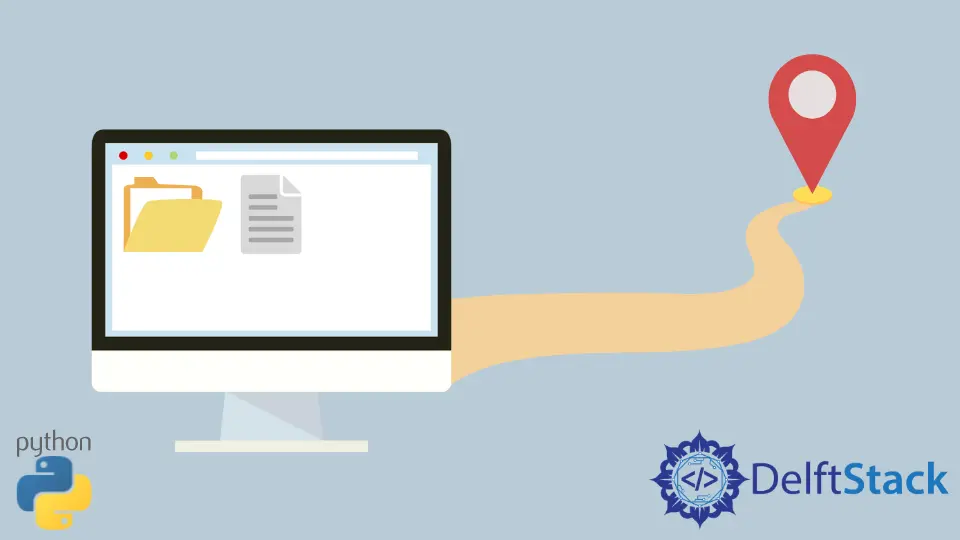
Understanding how to retrieve the path of the current file in Python is essential for many programming tasks. Whether you’re developing a script that needs to access other files in the same directory or you want to log the file path for debugging purposes, knowing how to get this information can save you time and effort.
In this tutorial, we will explore various methods to obtain the current file path in Python. We will cover the use of built-in libraries and provide clear code examples to help you grasp these concepts easily. Let’s dive in!
Using __file__
Attribute
One of the simplest ways to get the path of the current file in Python is by using the __file__
attribute. This special attribute holds the path of the script that is currently being executed. Here’s how you can use it:
import os
current_file_path = os.path.abspath(__file__)
print(current_file_path)
Output:
/path/to/your/current_file.py
In this example, we first import the os
module, which provides a way of using operating system-dependent functionality. The os.path.abspath()
function is then called with __file__
as an argument. This returns the absolute path of the current file, regardless of where the script is run from. This method is particularly useful because it works seamlessly in both standalone scripts and modules, making it a versatile choice.
Using os.getcwd()
Another method to obtain the current file path is by using the os.getcwd()
function. This function returns the current working directory, which is the directory from which your script is run. Here’s how you can implement it:
import os
current_working_directory = os.getcwd()
print(current_working_directory)
Output:
/path/to/your/current/working/directory
In this example, we again import the os
module and call os.getcwd()
. This returns the path of the directory from which the script was executed. While this method does not give you the path of the current file directly, it can be useful when you need to know the context in which your script is running. You can then combine this with the file name to create the full path if needed.
Using pathlib
Module
Python’s pathlib
module offers an object-oriented approach to handling filesystem paths. It provides a more intuitive way to work with paths compared to traditional methods. Here’s how to get the current file path using pathlib
:
from pathlib import Path
current_file_path = Path(__file__).resolve()
print(current_file_path)
Output:
/path/to/your/current_file.py
In this example, we import Path
from the pathlib
module. By creating a Path
object with __file__
, we can then call the resolve()
method to get the absolute path of the current file. This method is particularly powerful because it automatically handles symbolic links and provides a cleaner syntax. Using pathlib
is recommended for new projects as it improves code readability and maintainability.
Conclusion
In this tutorial, we’ve explored several effective methods to get the path of the current file in Python. We discussed using the __file__
attribute, the os.getcwd()
function, and the pathlib
module. Each method has its unique advantages, and depending on your specific needs, you can choose the one that best fits your project. Understanding how to manipulate file paths is crucial for any Python developer, and mastering these techniques will enhance your coding skills. Happy coding!
FAQ
-
How do I get the path of a file in a different directory?
You can specify the relative or absolute path to the desired file using theos.path
orpathlib
methods. -
Can I use these methods in Jupyter notebooks?
Yes, you can use the__file__
attribute in Python scripts, but in Jupyter notebooks, it will not return the notebook’s path. Instead, useos.getcwd()
to get the current working directory. -
Is
pathlib
available in all Python versions?
pathlib
was introduced in Python 3.4, so make sure you are using this version or later. -
What should I do if
__file__
is not defined?
If you run code in an interactive environment or a Jupyter notebook,__file__
may not be defined. In such cases, consider usingos.getcwd()
orpathlib
. -
Can I get the path of the current file in a Git repository?
Yes, you can use the methods described above to get the path of the current file, regardless of whether it’s in a Git repository or not.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn