How to Python Get Filename Without Extension From Path
-
Get Filename Without Extension From Path Using
pathlib.path().stem
Method in Python -
Get Filename Without Extension From Path Using
os.path.splitext()
andstring.split()
Methods in Python -
Get Filename From Path Using
os.path.basename()
andos.path.splitext()
Methods in Python
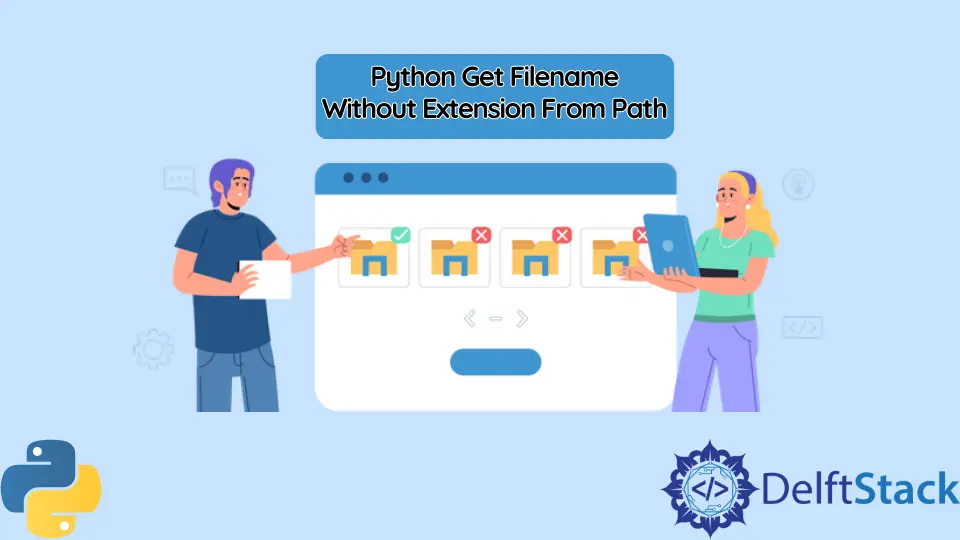
This tutorial will demonstrate various methods to get filename without extension from the file path in Python. Suppose the goal is to get the name of files from the list of file paths available in the form of a string, like from the path Desktop/folder/myfile.txt
, we get only the filename myfile
without .txt
extension.
Get Filename Without Extension From Path Using pathlib.path().stem
Method in Python
The path().stem
method takes the file path as input and returns the file name by extracting it from the file path. For example, from the path Desktop/folder/myfile.txt
, it will return myfile
without the .txt
extension.
The code example below demonstrates how to use the path().stem
to get file name without the file extension from the file path:
from pathlib import Path
file_path = "Desktop/folder/myfile.txt"
file_name = Path(file_path).stem
print(file_name)
Output:
myfile
Get Filename Without Extension From Path Using os.path.splitext()
and string.split()
Methods in Python
The path.splitext()
method of the os
module takes the file path as string input and returns the file path and file extension as output.
As we want to get the file name from the file path, we can first remove the file extension from the file path using the os.path.splitext()
method. The first element of the splitting result is the file path without extension. This result is further split it using /
as the separator. The last element will be the filename without extension. The code example below demonstrates how to get filename without extension from the file path using path.splitext()
and string.split()
methods.
import os
file_path = "Desktop/folder/myfile.txt"
file_path = os.path.splitext(file_path)[0]
file_name = file_path.split("/")[-1]
print(file_name)
Output:
test
Get Filename From Path Using os.path.basename()
and os.path.splitext()
Methods in Python
In Python the path.basename()
method of the os
module takes the file path as input and returns the basename extracted from the file path. For example, the basename of Desktop/folder/myfile.txt
is myfile.txt
.
As we want to get filename from the file path, the basename can be extracted using the path.basename()
method and filename using path.splitext()
. The code example below demonstrates to get filename from the file path using path.basename()
and path.splitext()
methods.
import os
file_path = "Desktop/folder/myfile.txt"
basename = os.path.basename(file_path)
file_name = os.path.splitext(basename)[0]
print(file_name)
Output:
myfile
myfile.tar.gz
, all the methods explained above will return myfile.tar
as the filename.Suppose we need to get filename without the part after .
like myfile
instead of myfile.tar
from the path Desktop/folder/myfile.tar.gz
, the string.index()
method can be used to extract only myfile
from myfile.tar
. But the drawback of this method is if the .
is part of the file name like my.file.tar.gz
, it will return my
as the file name.
The code example below how we can use string.index()
to remove .tar
from the output myfile.tar
of methods explained above:
file_name = "myfile.tar"
index = file_name.index(".")
file_name = file_name[:index]
print(file_name)
file_name = "my.file.tar"
index = file_name.index(".")
file_name = file_name[:index]
print(file_name)
Output:
myfile
my