Python 从路径获取不带扩展名的文件名
-
在 Python 中使用
pathlib.path().stem
方法从路径中获取没有扩展名的文件名 -
使用 Python 中的
os.path.splitext()
和string.split()
方法从路径中获取不带扩展名的文件名 -
在 Python 中使用
os.path.basename()
和os.path.splitext()
方法从路径中获取文件名
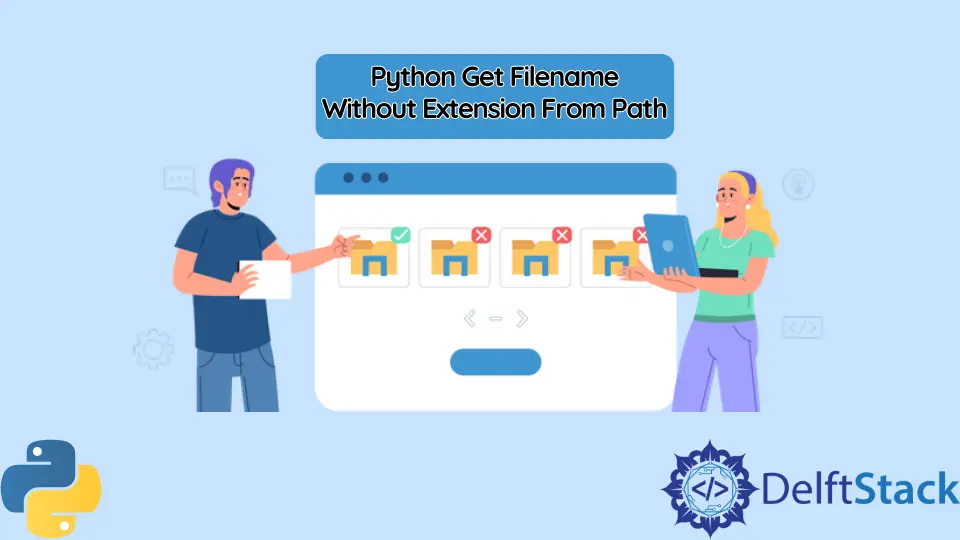
本教程将演示在 Python 中从文件路径中获取不带扩展名的文件名的各种方法。假设我们的目标是从以字符串形式存在的文件路径列表中获取文件名,比如从路径 Desktop/folder/myfile.txt
中,我们只得到没有 .txt
扩展名的文件名 myfile
。
在 Python 中使用 pathlib.path().stem
方法从路径中获取没有扩展名的文件名
path().stem
方法将文件路径作为输入,通过从文件路径中提取文件名来返回。例如,从路径 Desktop/folder/myfile.txt
中,它将返回不含 .txt
扩展名的 myfile
。
下面的代码示例演示了如何使用 path().stem
从文件路径中获取不含文件扩展名的文件名。
from pathlib import Path
file_path = "Desktop/folder/myfile.txt"
file_name = Path(file_path).stem
print(file_name)
输出:
myfile
使用 Python 中的 os.path.splitext()
和 string.split()
方法从路径中获取不带扩展名的文件名
os
模块的 path.splitext()
方法将文件路径作为字符串输入,并将文件路径和文件扩展名作为输出。
由于我们要从文件路径中获取文件名,所以可以先用 os.path.splitext()
方法将文件路径中的文件扩展名去掉。拆分结果的第一个元素就是没有扩展名的文件路径。这个结果使用/
作为分隔符进一步分割它。最后一个元素是没有扩展名的文件名。下面的代码示例演示了如何使用 path.splitext()
和 string.split()
方法从文件路径中获取不带扩展名的文件名。
import os
file_path = "Desktop/folder/myfile.txt"
file_path = os.path.splitext(file_path)[0]
file_name = file_path.split("/")[-1]
print(file_name)
输出:
test
在 Python 中使用 os.path.basename()
和 os.path.splitext()
方法从路径中获取文件名
在 Python 中,os
模块的 path.basename()
方法将文件路径作为输入,并返回从文件路径中提取的基名。例如,Desktop/folder/myfile.txt
的基名是 myfile.txt
。
由于我们要从文件路径中获取文件名,可以使用 path.basename()
方法提取基名,使用 path.splitext()
提取文件名。下面的代码示例演示了如何使用 path.basename()
和 path.splitext()
方法从文件路径中获取文件名。
import os
file_path = "Desktop/folder/myfile.txt"
basename = os.path.basename(file_path)
file_name = os.path.splitext(basename)[0]
print(file_name)
输出:
myfile
myfile.tar.gz
,则上述所有方法都会返回 myfile.tar
作为文件名。假设我们需要从路径 Desktop/folder/myfile.tar.gz
中获取不带 .
后面的部分的文件名,比如 myfile
而不是 myfile.tar
,可以使用 string.index()
方法从 myfile.tar
中只提取 myfile
。但是这个方法的缺点是,如果 .
是文件名的一部分,比如 my.file.tar.gz
,它将返回 my
作为文件名。
下面的代码示例,我们如何使用 string.index()
从上面解释的方法的输出 myfile.tar
中删除 .tar
。
file_name = "myfile.tar"
index = file_name.index(".")
file_name = file_name[:index]
print(file_name)
file_name = "my.file.tar"
index = file_name.index(".")
file_name = file_name[:index]
print(file_name)
输出:
myfile
my