How to Get Function Signature
-
Use
signature()
to Get Function Call Details in Python - Use Decorators to Get Function Call Details in Python
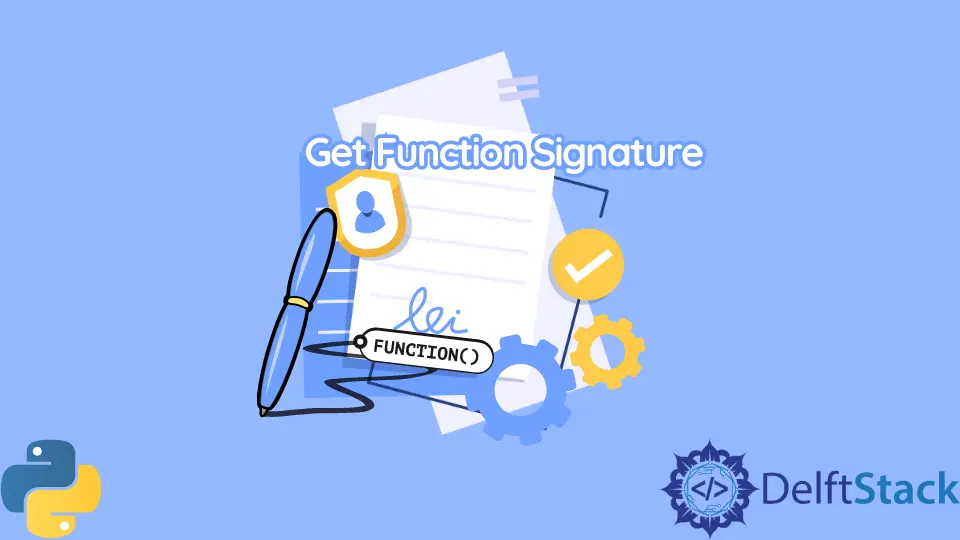
This tutorial will discuss a couple of methods for getting function call details quickly using Python. This is particularly useful when analyzing a large codebase, and figuring out what a function is doing at a glance becomes difficult.
Instead of going back and forth through the code, we have smarter solutions for this exact problem using signature()
functions and decorators.
Use signature()
to Get Function Call Details in Python
The easiest way to get this information is by using inspect.signature()
function. The document for Python 3.5+ recommends using this function.
inspect.signature()
accepts a variety of callables. This includes functions, classes and functools.partial()
objects.
The function returns the callable’s annotation. If no signature can be provided, the function raises a ValueError
; if the object type is not supported, it raises a TypeError
.
Example Code:
import inspect
def foo(x, y, a="anything"):
pass
print(inspect.signature(foo))
It should print the following on your terminal.
(x, y, a="anything")
Use Decorators to Get Function Call Details in Python
Functions in Python have a few attributes that we can make use of. Among these attributes is __code__
.
The __code__
attribute further has attributes we can use. Below, you can find each of them listed, explaining what they do.
Below that, we will include a code snippet to write your function that can perform this task. We use the __name__
attribute to get the function name.
-
co_varnames
returns a tuple of the names of the arguments passed to the function and the local variables used inside the function. -
co_argcount
returns the number of arguments. However, this count excludes the keyword-only arguments (kwargs
),*args
, and**args
.We have included a way to print all of these arguments in our snippet.
def get_func_dets(func):
# the point of using argcount is to ensure we only get
argument_names = func.__code__.co_varnames[: func.__code__.co_argcount]
func_name = func.__name__
# getting variable length arguments and keyword arguments
def inner_fun(*args, **kwargs):
print(func_name, "(", end="")
print(
", ".join(
"%s = %r" % ent
for ent in zip(argument_names, args[: len(argument_names)])
),
end=", ",
)
print("args = ", list(args[len(argument_names) :]), end=",")
print("kwargs =", kwargs, end="")
print(")")
return inner_func
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn