How to Rename Python Function Pointer
-
Rename Function Using Its Pointer With the
getattr()
Function in Python -
Rename Function Using Its Pointer With the
import
Statement in Python -
Rename Function Using Its Pointer With the
package.function
Statement in Python - Rename Function Using Its Pointer With a Reference Dictionary in Python
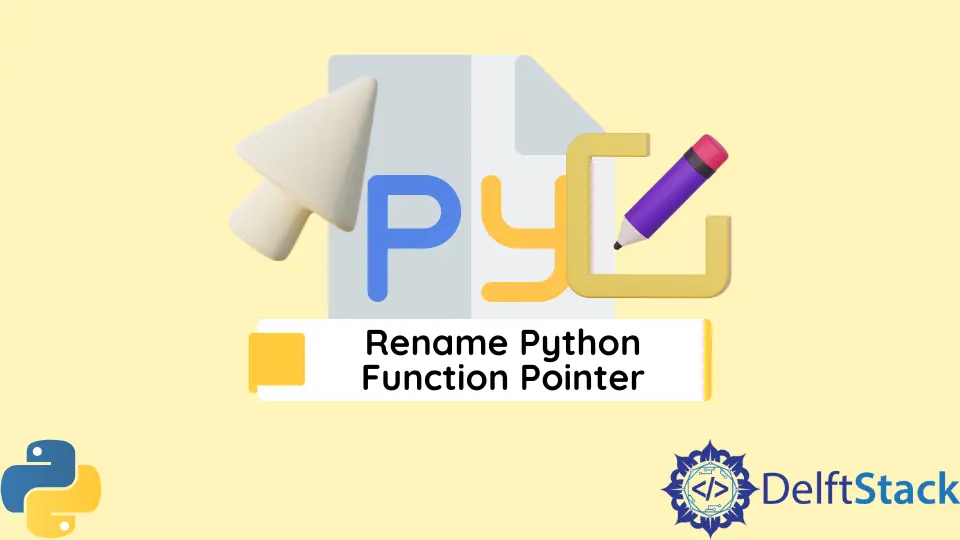
This tutorial will discuss different methods to rename a function using a pointer in Python.
Rename Function Using Its Pointer With the getattr()
Function in Python
We have a file named functions.py
where we’ve defined two functions named square
and cube
.
We can use several methods to access these functions with some other name. There is a well-known notion that everything in Python is an object.
And as we all know, objects are called by reference. Even though Python has no support for user-defined pointers, we can still work around this problem with the following methods.
Our first method involves using the getattr()
function in Python. The getattr()
is a built-in function that returns the value of the named attribute of an object.
It can be used with the __import__()
function. The __import__()
is another built-in function used to import packages, libraries, and modules in our code in Python.
Once imported, we can then assign a new name to the original function by assigning the return value of the getattr()
function to a new variable. The code snippet demonstrates the working of this phenomenon in Python.
pkg = __import__("functions")
func = getattr(pkg, "cube")
print(func(3))
Output:
27
We imported the file functions.py
with the __import__()
function and assigned its value to the pkg
object.
We then fetched the original cube()
function inside the pkg
object and assigned its value to the func
variable. We can now call the functions.cube
function with just the func()
function.
In the end, we printed the cube of 3 with the func(3)
.
Rename Function Using Its Pointer With the import
Statement in Python
This method involves using the import
statement to rename the function.
The import
statement is used to import packages, libraries, or modules in our code. We can use the as
statement with our import
statement to rename our packages, libraries, or modules.
The code snippet for renaming our packages with the import
and as
statements is below.
from functions import square as sq
print(sq(2))
Output:
4
We imported our square()
function inside the functions.py
file as sq
.
Now, we can use the square()
function inside our current file by writing just sq()
. This method is very convenient and easy to understand.
But, this method fails if we want to use multiple names for our one function. The methods mentioned in the next sections address this problem and provide a solution that can also handle multiple names for just one single function.
Rename Function Using Its Pointer With the package.function
Statement in Python
Our square()
function is inside the functions.py
file, we can reference this function by first importing the funcitons.py
file inside our current code and writing function.square()
.
This serves as a reference to the original function. We can assign its value to any variable in our code and use that variable to call the function.
The code snippet provided below allows us to understand how this method works.
import functions
sq = functions.square
print(sq(3))
Output:
9
We renamed our functions.square()
function to sq()
by assigning the reference of the functions.square
to sq
.
We then used the renamed sq()
function to calculate and print the square of 3
. This method is very helpful when assigning multiple names to the same function.
We have to assign the value of functions.square
to multiple variables to do this. The following code example shows us how we can achieve this.
import functions
sq = functions.square
sq1 = functions.square
sq2 = functions.square
print(sq(3))
print(sq1(3))
print(sq2(3))
Output:
9
9
9
We called the same functions.square()
function with three different names in the same code file. This method works great with multiple names for a function.
It can also be used for multiple functions. The only problem with this method is that it is a little code intensive and feels very redundant.
The method discussed in the next section addresses this issue.
Rename Function Using Its Pointer With a Reference Dictionary in Python
We can use the dictionary of references to the original functions to assign multiple names to multiple original functions.
This method is straightforward to implement. We have to assign a key to the original reference.
We can then access the reference to the original function by using the dictionary name with the assigned key. The following code snippet shows a working implementation of this method.
import functions
functionsdict = {"square": functions.square, "cube": functions.cube}
c = functionsdict["cube"]
print(c(3))
Output:
27
We created a dictionary that assigns a key to all references of the original functions. We then renamed the functions.cube
to c
using the functionsdict
dictionary and the corresponding key cube
.
In the end, we used the c()
function to print the cube of 3
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn