How to Get Function Name in Python
-
Method 1: Use the
__name__
Property to Get the Function Name in Python -
Method 2: Using the
inspect
Module -
Method 3: Using
sys._getframe()
-
Method 4: Using
func.__qualname__
Attribute -
Method 5: Using the
traceback
Module - Conclusion
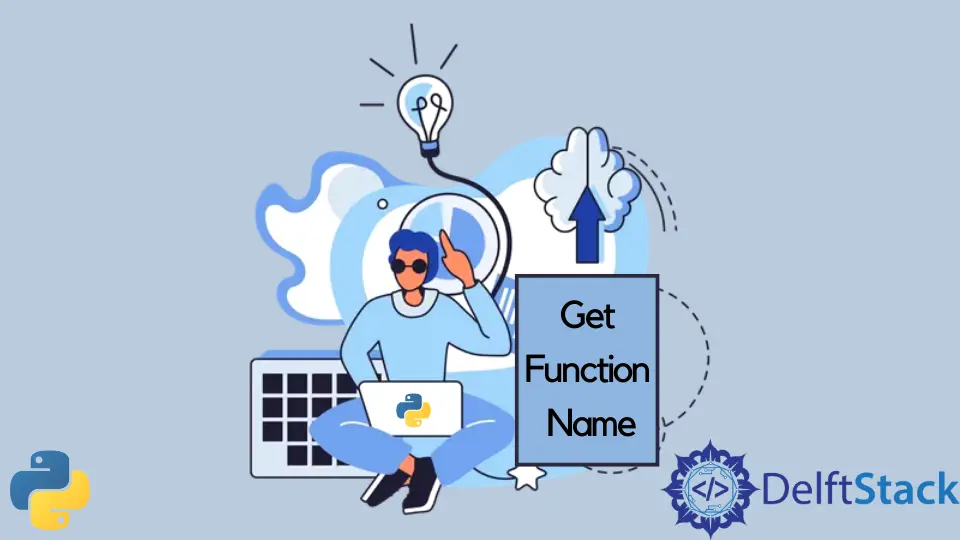
This tutorial will introduce how to get the function name in Python. This comprehensive article delves into various methods for retrieving a function’s name in Python, providing in-depth explanations and examples.
Method 1: Use the __name__
Property to Get the Function Name in Python
In Python, every single function that is declared and imported in your project will have the __name__
property, which you can directly access from the function.
To access the __name__
property, just put in the function name without the parentheses and use the property accessor .__name__
. It will then return the function name as a string.
The below example declares two functions, calls them, and prints out their function names.
def functionA():
print("First function called!")
def functionB():
print("\nSecond function called!")
functionA()
print("First function name: ", functionA.__name__)
functionB()
print("Second function name: ", functionB.__name__)
Output:
First function called!
First function name: functionA
Second function called!
Second function name: functionB
Note that this solution also works with the imported and pre-defined functions. Let’s try it out with the print()
function itself and a function from an imported Python module os
.
import os
print("Function name: ", print.__name__)
print("Imported function name: ", os.system.__name__)
Output:
Function name: print
Imported function name: system
Method 2: Using the inspect
Module
Python’s inspect
module provides a comprehensive way to access various attributes of live objects, including functions.
The inspect.currentframe()
function retrieves the current frame object. From there, access the f_code
attribute to obtain the code object, which contains information about the function.
Finally, the co_name
attribute within the code object provides the function’s name.
Here’s a detailed example:
import inspect
def get_function_name():
frame = inspect.currentframe()
code_obj = frame.f_code
function_name = code_obj.co_name
return function_name
# Accessing the function name using the inspect module
function_name = get_function_name()
print("Function name:", function_name)
Output:
Function name: get_function_name
This method offers more detailed information than the __name__
attribute and is especially helpful for debugging.
Method 3: Using sys._getframe()
The sys
module in Python provides access to system-specific parameters and functions. Within this module, the _getframe()
function is employed to access the current frame.
By specifying the parameter 0
, you can obtain the current function’s frame. From this frame, you can extract the function name using the f_code.co_name
attribute.
Here’s a detailed example:
import sys
def retrieve_function_name():
frame = sys._getframe(0)
code_obj = frame.f_code
function_name = code_obj.co_name
return function_name
# Accessing the function name using sys._getframe()
function_name = retrieve_function_name()
print("Function name:", function_name)
Output:
Function name: retrieve_function_name
Method 4: Using func.__qualname__
Attribute
In Python 3, the __qualname__
attribute provides a fully qualified name for a function. This attribute is especially useful when dealing with nested functions or methods within classes.
Here’s a detailed example:
class MyClass:
def my_method(self):
pass
# Accessing the method's qualified name using func.__qualname__
method_name = MyClass.my_method.__qualname__
print("Method name:", method_name)
Output:
Method name: MyClass.my_method
Method 5: Using the traceback
Module
The traceback
module in Python is dedicated to handling and manipulating tracebacks and frames. The traceback.extract_stack()
function retrieves a list of stack frame records. Each record holds information about a frame, including the function name.
Here’s a detailed example:
import traceback
def fetch_function_name():
stack = traceback.extract_stack()
current_frame = stack[-1]
function_name = current_frame.name
return function_name
# Accessing the function name using the traceback module
function_name = fetch_function_name()
print("Function name:", function_name)
Output:
Function name: fetch_function_name
Conclusion
Understanding various methods to retrieve a function’s name is vital for effective Python programming.
Each method has its strengths and applications, enabling you to choose the approach that aligns with your coding style and requirements.
Whether you’re debugging, documenting, or testing your code, having a clear understanding of these techniques empowers you to work efficiently in Python.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn