How to Find the Index of an Element in a List in Python
-
Use the List
index()
Method to Find the Index of a List in Python -
Use
numpy.where()
to Find the Index of a List in Python
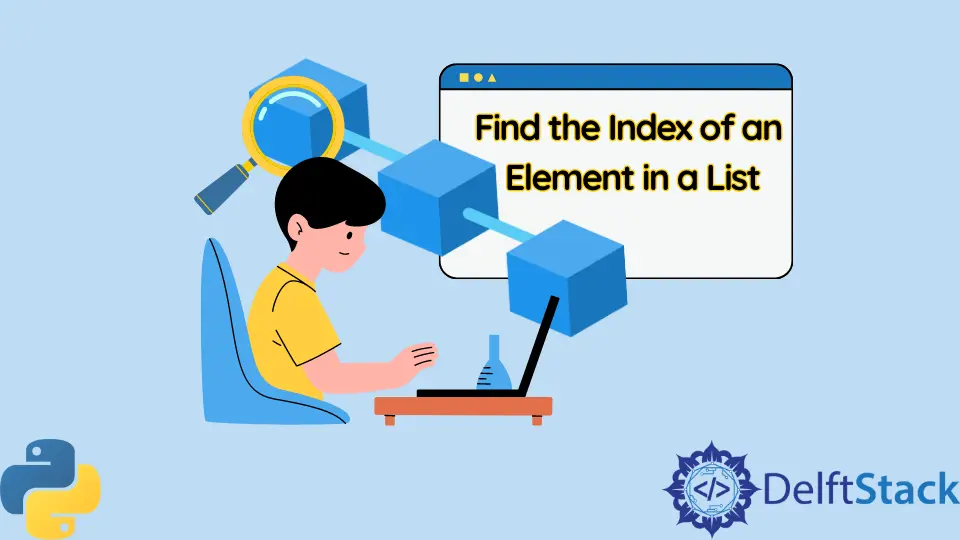
This tutorial will demonstrate how to find the position or index of an element in a Python list.
Use the List index()
Method to Find the Index of a List in Python
Python list has a built-in method called index()
, which accepts a single parameter representing the value to search within the existing list. The function returns the index of the first occurrence that it finds starting from index 0
regardless of how many times it occurs within the list.
For example, declare a list with a repeating value of 20
and call the function index(20)
and print what it returns.
lst = [13, 4, 20, 15, 6, 20, 20]
print(lst.index(20))
Output:
2
The first occurrence of the value 20
found within the lst
array was on index 2
, which is the result of the function call. The other elements with the same value are ignored since it already has found a match within the list.
What happens when a value does not exist within the given list, and we call index()
passing the non-existent value? Let’s take this for example.
lst = [13, 4, 20, 15, 6, 20, 20]
print(lst.index(21))
Output:
ValueError: 21 is not in list
The function will throw an error if the index isn’t found within the list. In some cases, this might be unfavorable to invoke an error. To avoid this, catch the error with a try...except
block and make it so that if the index does not exist within the list, assign it as -1
.
lst = [13, 4, 20, 15, 6, 20, 20]
try:
ndx = lst.index(21)
except:
ndx = -1
print(ndx)
Output:
-1
This way, an explicit error won’t have to be invoked, and the program can continue running after the operation.
Use numpy.where()
to Find the Index of a List in Python
The NumPy
module has a pre-defined function called where()
which deals with locating multiple items within a list and accepts a condition.
In this case, we will exclusively use where()
to locate a given value’s indices. Unlike the built-in index()
function, the where()
function can return a list of indices where the value is located if it exists more than once within a list. This is useful if you need all the occurrences of the value instead of just the first occurrence.
The first step is to convert a Python list into a NumPy
array. To do this, call the function np.array()
.
import numpy as np
lst = np.array(lst=[13, 4, 20, 15, 6, 20, 20])
After initializing the NumPy
array, we only need to fill the first parameter of where()
. Initialize the first parameter as lst == 20
to locate the given list’s indices with the value 20
.
import numpy as np
lst = [13, 4, 20, 15, 6, 20, 20]
lst = np.array(lst)
result = np.where(lst == 20)
print(result)
Output:
(array([2, 5, 6]),)
Since NumPy
mainly deals with matrices, the where()
function returns a tuple of arrays instead of just a single list. If outputting only the single list is preferred, then call the first index of the result and output it using print()
.
import numpy as np
lst = [13, 4, 20, 15, 6, 20, 20]
lst = np.array(lst)
result = np.where(lst == 20)
print(result[0])
Output:
[2 5 6]
Note that NumPy
arrays are delimited by single whitespace instead of the normal commas.
In summary, the index()
function is the easiest way to find the position of an element within a Python list. Although, this function only returns the index of the first occurrence of the given value.
To return multiple indices if multiple instances of the value exist, then you can opt to use the where()
function in the NumPy
module.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python