How to Escape Curly Braces in F-String in Python
- Curly Braces in F-String in Python
- Use Double Curly Braces to Escape Curly Braces in F-String
- Use Triple Curly Braces to Escape Curly Braces in F-String
- Mixing Escaped and Unescaped Curly Braces in F-Strings
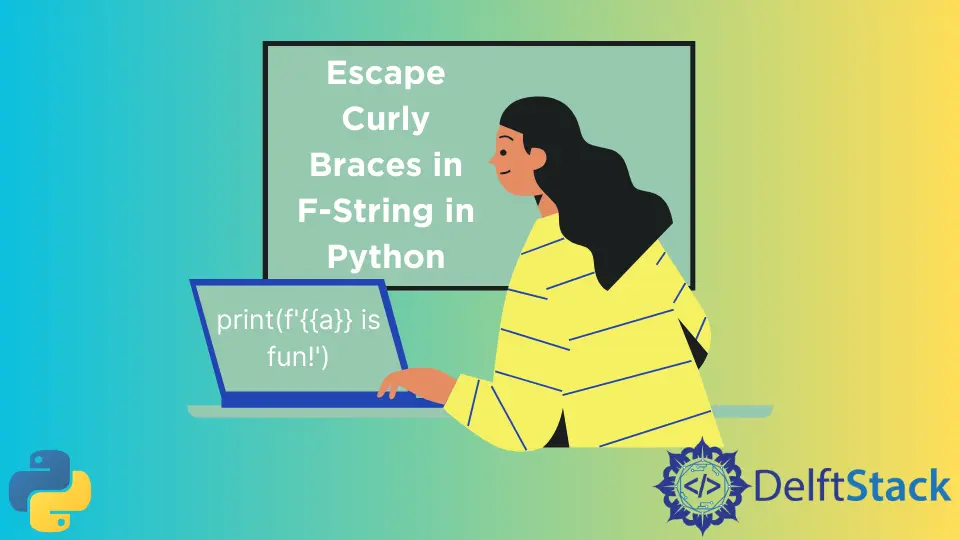
F-strings, introduced in Python 3.6 and later versions, revolutionized the way Python developers format strings. These formatted string literals, denoted by the f prefix, allow for the seamless inclusion of expressions within strings, providing dynamic and readable outputs.
While f-strings offer a versatile approach to string formatting, they also introduce unique challenges when working with curly braces {}. Curly braces are special characters in f-strings used to enclose expressions that Python evaluates and inserts into the final string. However, there are scenarios where you may need to include literal curly braces within your formatted strings.
In this exploration of curly braces in Python’s f-strings, we’ll delve into various techniques and examples to master their usage. We’ll cover escaping curly braces, handling formatting intricacies, and even mixing escaped and unescaped curly braces to craft precisely the strings your Python code demands.
Curly Braces in F-String in Python
A function called f-string is introduced in Python 3.6 and its newer versions to format a string. The same function, format()
, is used in older versions, but the f-string is faster and more concise than the format()
method.
First, we will create an f-string. To create an f-string, precede the string you want to format with f
, and then inside curly brackets, we have to specify the variable of that string which we want to format our own way.
Consider the following example:
# Python 3.x
a = "programming"
print(f"{a} is fun!")
Output:
programming is fun!
As you can see, the string is formatted, but the problem is we cannot get the curly brackets in the output. So for this purpose, we will use double braces instead of one.
Use Double Curly Braces to Escape Curly Braces in F-String
Example:
# Python 3.x
a = "programming"
print(f"{{a}} is fun!")
Output:
{a} is fun!
In this Python code snippet, we are using an f-string to format a string that includes curly braces. The variable a
contains the string "programming"
.
In the f-string "{{a}} is fun!"
, we use double curly braces {{
and }}
to escape the curly braces and treat them as literal characters. This is necessary because single curly braces {
and }
are special characters in f-strings and are used to enclose expressions that will be evaluated and included in the final string.
To overcome this problem, we will use 3 braces in total.
Use Triple Curly Braces to Escape Curly Braces in F-String
When we run above code, we observe that the output contains the text {a}
instead of the value of the variable a
. This is because the inner pair of curly braces {a}
is treated as a literal string, including the curly braces themselves.
To actually include the value of the variable a
in the output, we need to modify the f-string as follows:
# Python 3.x
a = "programming"
print(f"{{{a}}} is fun!")
Output:
{programming} is fun!
Now, we have used triple curly braces {{{
and }}}
to escape the curly braces and include the value of the variable a
within the string. The expression inside the f-string is {a}
, which will be evaluated, and its result (the value of a
) will replace it in the final string.
So to print curly brackets while using the f-string method, we need to use triple braces instead of one.
Mixing Escaped and Unescaped Curly Braces in F-Strings
As we’ve seen earlier, curly braces {}
play a crucial role in f-strings by enclosing expressions that will be evaluated and included in the final string. However, there are scenarios where you may need to include literal curly braces within your formatted string. This can be achieved by mixing escaped (double) curly braces {{
and }}
with unescaped (single) curly braces {
and }
in an f-string.
Let’s explore this concept with an example:
# Python 3.x
a = "programming"
print(f"{{a}} is fun! {{{a}}} is also fun!")
In this example, we have an f-string that combines escaped and unescaped curly braces. Here’s a breakdown of the f-string:
{{a}}
is enclosed within double curly braces,{{
and}}
. This is used to escape the inner curly braces and treat them as literal characters. As a result, the expression{a}
inside these braces is treated as a plain string and not evaluated.{{{a}}}
contains triple curly braces{{{
,{a}
, and}}}
. The double curly braces{
and}
are escaped, while the single curly braces{a}
are unescaped. This means that the expression{a}
inside the unescaped curly braces will be evaluated, and its result (the value ofa
) will be included in the final string.
Output:
{a} is fun! {programming} is also fun!
As we can see from the output, the f-string correctly handles both escaped and unescaped curly braces. The text "{a} is fun!"
is included as-is, while "{programming}"
is replaced with the value of the variable a
, resulting in the string "programming is also fun!"
.
Mixing escaped and unescaped curly braces in f-strings provides you with the flexibility to include literal curly braces in your formatted strings while still benefiting from the expressive power of f-string expressions. This technique is particularly useful when you need to create complex string templates or when dealing with string formats that contain special characters.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn