Python Dynamic Variable Name
-
Use the
for
Loop to Create Dynamic Variable Names in Python - Use a Dictionary to Create a Dynamic Variable Name in Python
-
Use the
exec()
Function to Create a Dynamic Variable Name in Python -
Create Dynamic Variable Names in Python Using
setattr()
for Objects -
Create Dynamic Variable Names in Python Using
locals() with update()
- Conclusion
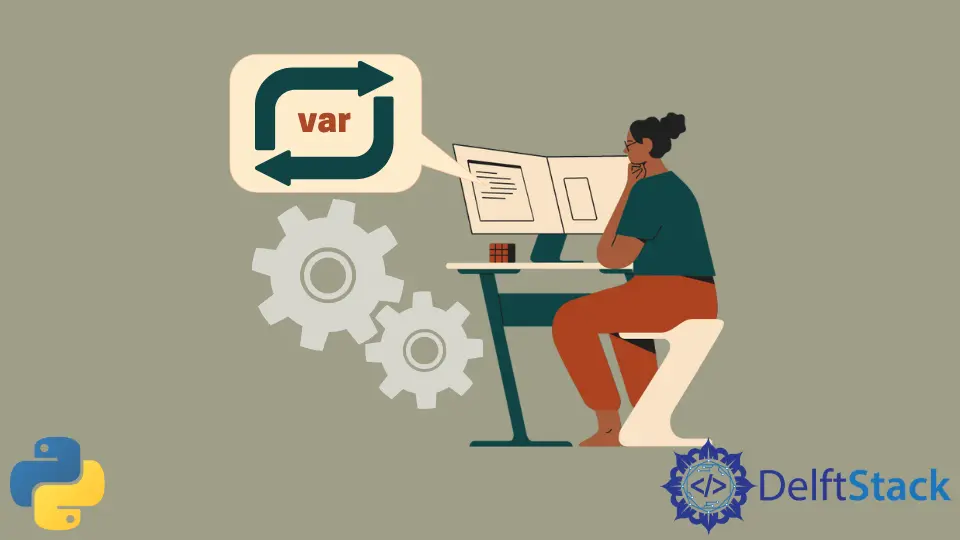
Dynamic variable names, also known as variable variables, enable Python developers to dynamically generate and manage variables within their code. In this article, we’ll explore various methods to achieve dynamic variable creation, leveraging the inherent flexibility of Python.
We’ll cover methods such as using the for
loop, dictionaries, exec()
function, setattr()
for objects, and locals()
with update()
.
Use the for
Loop to Create Dynamic Variable Names in Python
The for
loop, combined with the globals()
function, offers a powerful way to create dynamic variable names.
The for
loop in Python is an iterative tool that allows you to repeat a block of code a specified number of times. When combined with the globals()
function, which provides access to the global symbol table as a dictionary, you can dynamically create variables.
In the following example, we will use the for
loop to iterate over a range of numbers. Inside the loop, we will create dynamic variables using the globals()
function.
for n in range(0, 7):
globals()["dynamic_var%s" % n] = "Hello"
for x in range(0, 7):
globals()[f"variable{x}"] = f"Hello from variable number {x}!"
print(variable5)
In the first loop, for n in range(0, 7)
, we iterate over the numbers from 0
to 6
. Inside the loop, globals()["dynamic_var%s" % n] = "Hello"
creates dynamic variables named dynamic_var0
through dynamic_var6
, each initialized with the string Hello
.
In the second loop, for x in range(0, 7)
, we use an f-string with globals()[f"variable{x}"] = f"Hello from variable number {x}!"
to create dynamic variables named variable0
through variable6
, each containing a unique string that includes the variable number.
After running the provided code, the output will be:
Hello from variable number 5!
In this output, the dynamically created variable variable5
is printed, demonstrating how the for
loop and globals()
function can be utilized to generate dynamic variable names in Python.
Remember that while dynamic variable names offer flexibility, it’s important to use them judiciously to maintain code clarity and readability.
Use a Dictionary to Create a Dynamic Variable Name in Python
Another approach to achieving dynamic variable names involves using a dictionary, a versatile data structure in Python.
A dictionary in Python is a collection of key-value pairs, providing a flexible way to store and retrieve data. By utilizing the key-value structure, we can dynamically create variable names based on the keys of the dictionary.
In this example, we’ll see how this concept can be applied to create dynamic variables.
variable_name = "dynamic_var"
value = "Hello"
dynamic_variables = {variable_name: value}
print(dynamic_variables["dynamic_var"])
In the provided code, variable_name
is assigned the value dynamic_var
, and value
is assigned the string Hello
. We then create a dictionary named dynamic_variables
using the key-value pair {variable_name: value}
.
This effectively associates the value Hello
with the key dynamic_var
in the dictionary. Finally, we print the value of the dynamic variable using print(dynamic_variables["dynamic_var"])
.
Output:
Hello
This output confirms that we successfully created a dynamic variable named dynamic_var
and accessed its value through the dictionary.
Use the exec()
Function to Create a Dynamic Variable Name in Python
Another method to generate dynamic variable names involves the use of the exec()
function. While this approach offers flexibility, it’s essential to exercise caution as exec()
can pose security risks if not used carefully.
The exec()
function in Python is a built-in function that dynamically executes a string of Python code. It takes a string as an argument, interprets it as a sequence of Python statements, and executes them.
This can be useful for creating dynamic variable names on the fly.
Its syntax is as follows:
exec(object[, globals[, locals]])
Where:
object
: A string representing a block of Python code to be executed.globals
(optional): A dictionary representing the global symbol table.locals
(optional): A dictionary representing the local symbol table.
Here’s an example illustrating the use of the exec()
function to create dynamic variables:
variable_name = "dynamic_var"
value = "Hello"
exec(f"{variable_name} = '{value}'")
print(dynamic_var)
In the provided code, we have a variable variable_name
assigned to the string dynamic_var
and another variable value
assigned to the string Hello
. Using the exec()
function, we dynamically create a variable named dynamic_var
with the value Hello
by executing the string "{variable_name} = '{value}'"
.
The {}
placeholders in the string are filled in by the values of variable_name
and value
using an f-string. The resulting code is equivalent to writing dynamic_var = 'Hello'
in our Python script.
Finally, we print the value of the dynamically created variable using print(dynamic_var)
.
Output:
Hello
While the exec()
function offers flexibility, it should be used with caution, especially when you’re handling user input, to prevent security risks associated with code injection.
Create Dynamic Variable Names in Python Using setattr()
for Objects
The setattr()
function provides a way to dynamically set attributes or create variables for objects. This method is particularly useful when working with classes and objects.
The setattr()
function is a built-in Python function that allows you to set the value of an attribute for an object. When it comes to dynamic variable creation, setattr()
can be used to assign values to dynamically generated attributes.
Its syntax is as follows:
setattr(object, attribute, value)
Where:
object
: The object for which the attribute is being set.attribute
: The name of the attribute to set.value
: The value to assign to the attribute.
Let’s delve into an example demonstrating the use of setattr()
to create dynamic variables for objects:
class MyClass:
pass
obj = MyClass()
variable_name = "dynamic_var"
value = "Hello"
setattr(obj, variable_name, value)
print(obj.dynamic_var)
In this example, we define a simple class, MyClass
. We then create an instance of this class named obj
.
Next, we have a variable variable_name
assigned the value dynamic_var
and another variable value
assigned the string Hello
. The setattr()
function is used to dynamically create an attribute for the object obj
with the name specified by variable_name
and assign it the value of value
.
The line setattr(obj, variable_name, value)
is equivalent to obj.dynamic_var = 'Hello'
, dynamically creating an attribute named dynamic_var
for the object obj
.
Finally, we print the value of the dynamically created attribute using print(obj.dynamic_var)
.
Output:
Hello
Using setattr()
in this manner can be particularly beneficial when dealing with object-oriented programming and dynamic attribute assignment.
Create Dynamic Variable Names in Python Using locals() with update()
The combination of the locals()
function and the update()
method offers another dynamic approach to creating variable names within the local scope. This method is particularly useful when you need to create variables dynamically within a specific context.
The locals()
function in Python returns a dictionary representing the current local symbol table. When combined with the update()
method, which adds key-value pairs to the dictionary, it allows for the dynamic creation of variables within the local scope.
Let’s delve into an example that demonstrates the use of locals()
with update()
:
variable_name = "dynamic_var"
value = "Hello"
locals().update({variable_name: value})
print(dynamic_var)
In this example, variable_name
is assigned the value dynamic_var
, and value
is assigned the string Hello
. The locals()
function is used to obtain the local symbol table, and update()
is applied to add a key-value pair to this dictionary.
The key is specified by variable_name
, and its corresponding value is given by value
.
Here, the line locals().update({variable_name: value})
is equivalent to creating a local variable dynamically, such as dynamic_var = 'Hello'
, within the local scope.
Finally, we print the value of the dynamically created variable using print(dynamic_var)
.
Output:
Hello
While this method provides flexibility for dynamic variable creation, it’s crucial to consider the appropriate scope for your variables and use this approach judiciously.
Conclusion
Dynamic variable creation in Python provides flexibility but should be approached judiciously. Consider the specific requirements of your code and choose the method that aligns with your needs.
Whether using loops, dictionaries, exec()
, setattr()
, or locals()
with update()
, understanding these techniques allows you to manage variables dynamically in Python.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn