How to Create and Access Docstrings in Python
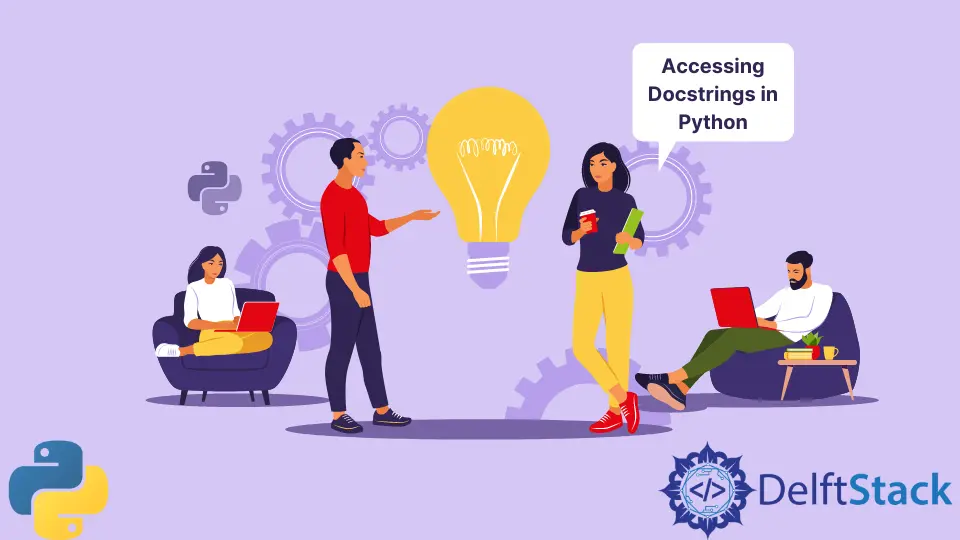
According to the python glossary, docstring is the first string literal that appears immediately after defining a class, method, or function.
Docstring defined after the definition of any object are often associated with that particular object and can be accessed using the __doc__
attribute alongside the print or help()
function. Docstrings are generally defined by enclosing string literals in triple-single or triple-double quote marks, as shown below:
def addtwo(x):
"""Takes in a number x and adds two to it."""
return x + 2
print(addtwo.__doc__)
Output:
Takes in a number x and adds two to it.
One of the best practices for writing clean code is providing a docstring that describes how a particular function or module works. Besides providing vital information to the developer, using Sphinx docstrings can be extracted to create beautiful documentation in different formats such as plain text, HTML, ePub, or PDF.
Docstrings are largely classified into two categories:
- Single line
- Multiline
Python Single Line Docstrings
Conventionally, docstrings are considered single line only if both the opening and closing triple-single or triple-double are on the same line.
Single lines often follow a similar format and are less descriptive. Instead, they provide a short explanation of what the object does and its return value.
Furthermore, single-line docs strings should not have a leading blank space and should always begin with a capital letter and a full stop marking the end. The __doc__
attribute can also be used to access single line docstrings, as shown below.
def square(x):
"""Takes in a number x and returns its square."""
return x ** 2
print(square(10))
print(square.__doc__)
Output:
100
Takes in a number x and adds two to it.
Python Multiline Docstrings
Similarly, multiline docstrings are also defined through the enclosure of string literals within triple-single or triple-double quotation marks. However, multiline docs strings generally follow a different structure or format.
Multiline docstrings usually span across more than one line. The first line is normally dedicated to solely providing a short description of the object.
This description is followed by one blank line and a more elaborate description of the parameter, if any, and return arguments of the object in subsequent lines. Large libraries such as scikit learn
or pandas
also include a section enlisting the packages available within that library.
Although multiline docstrings generally have a similar structure, some differences depend on the object type. In function objects, docstrings would follow the structure below.
def add_numbers(x, y):
"""
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
"""
sum = x + y
return sum
print(add_numbers.__doc__)
Output:
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
In large modules such as Scikit
, NumPy
, or Pandas
, docstrings follow the format below.
We can also use the help()
function and the __doc__
attribute to access the docstrings, as we will see later. We can use the __doc__
attribute to access docstrings in modules like below.
import pandas as pd
print(pd.__doc__)
Output:
Docstrings created under classes should state the class’s functionality, list all instance variables of that specific class, and all the public methods. Classes that inherit from the main class, also known as subclasses, should have their docstrings which can be accessed separately.
As shown below, docstrings can be created and accessed in classes using the __doc___
attribute.
class Car:
"""
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
"""
def __init__(self, manufacturer, model, color):
"""
Constructs all the attributes for the car object.
Parameters
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
"""
self.model = model
self.color = color
self.manufacturer = manufacturer
def display_info(self, color, model, manufacturer):
"""
Prints the model of the car its color and the manufacturer.
Parameters
----------
model : str
color : str
manufacture: str
Returns
-------
None
"""
print(f"The {self.color} {self.model} is manufactured by {self.manufacturer}")
print(Car.__doc__)
help(Car)
Output:
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
Help on class Car in module __main__:
class Car(builtins.object)
| Car(manufacturer, model, color)
|
| A class to represent a car.
|
| ...
|
| Attributes
| ----------
While Python docstrings seem to perform similar roles in helping developers understand their code, commenting is ignored by interpreters. In Python, single-line comments are preceded by a hash symbol and cannot be more than one line.
Although multiline comments are also written within triple-double or triple-single quotation marks, comments generally don’t follow a specific structure. Unlike docstrings which seem to have slightly different formats depending on the circumstances that they have been used, comments, on the other hand, are generally used in the same way regardless of the program.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn