在 Python 中访问文档字符串
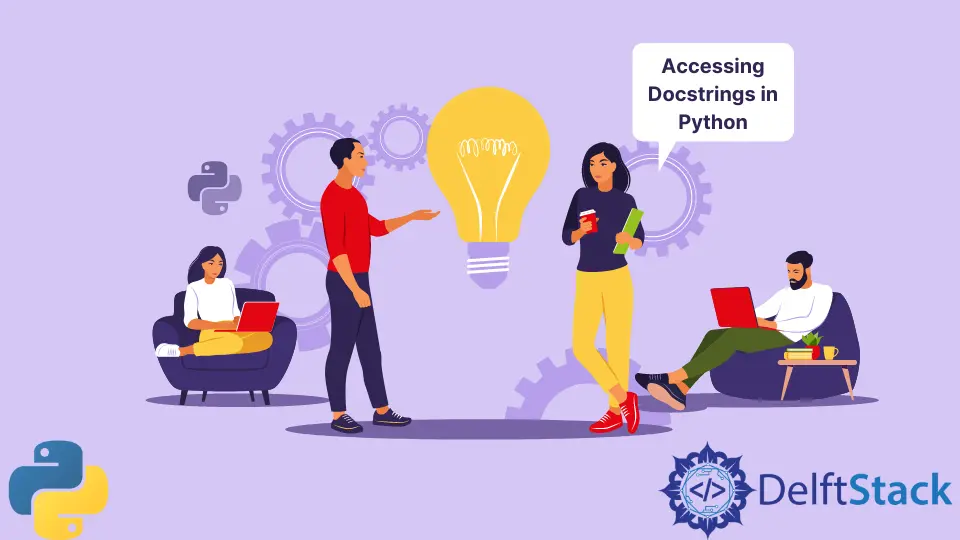
根据 python 词汇表,docstring 是在定义类、方法或函数后立即出现的第一个字符串文字。
在任何对象的定义之后定义的文档字符串通常与该特定对象相关联,并且可以使用 __doc__
属性与 print 或 help()
函数一起访问。文档字符串通常通过将字符串文字包含在三重单引号或三重双引号中来定义,如下所示:
def addtwo(x):
"""Takes in a number x and adds two to it."""
return x + 2
print(addtwo.__doc__)
输出:
Takes in a number x and adds two to it.
编写干净代码的最佳实践之一是提供描述特定函数或模块如何工作的文档字符串。除了向开发人员提供重要信息外,还可以提取 Sphinx 文档字符串以创建不同格式的精美文档,例如纯文本、HTML、ePub 或 PDF。
文档字符串主要分为两类:
- 单线
- 多线
Python 单行文档字符串
按照惯例,只有当开始和结束三重单或三重双在同一行上时,文档字符串才被视为单行。
单行通常遵循类似的格式并且不太具有描述性。相反,它们提供了对象的作用及其返回值的简短说明。
此外,单行文档字符串不应有前导空格,并且应始终以大写字母开头并以句号结尾。__doc__
属性也可用于访问单行文档字符串,如下所示。
def square(x):
"""Takes in a number x and returns its square."""
return x ** 2
print(square(10))
print(square.__doc__)
输出:
100
Takes in a number x and adds two to it.
Python 多行文档字符串
类似地,多行文档字符串也通过将字符串文字包含在三重单引号或三重双引号中来定义。但是,多行文档字符串通常遵循不同的结构或格式。
多行文档字符串通常跨越多行。第一行通常专用于仅提供对象的简短描述。
此描述后跟一个空行和更详细的参数描述(如果有),并在后续行中返回对象的参数。大型库,如 scikit learn
或 pandas
还包括一个部分,列出该库中可用的包。
尽管多行文档字符串通常具有相似的结构,但某些差异取决于对象类型。在函数对象中,文档字符串将遵循以下结构。
def add_numbers(x, y):
"""
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
"""
sum = x + y
return sum
print(add_numbers.__doc__)
输出:
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
在诸如 Scikit
、NumPy
或 Pandas
等大型模块中,文档字符串遵循以下格式。
我们还可以使用 help()
函数和 __doc__
属性来访问文档字符串,我们将在后面看到。我们可以使用 __doc__
属性来访问模块中的文档字符串,如下所示。
import pandas as pd
print(pd.__doc__)
输出:
在类下创建的文档字符串应该说明类的功能,列出该特定类的所有实例变量以及所有公共方法。从主类继承的类,也称为子类,应该有可以单独访问的文档字符串。
如下所示,可以使用 __doc___
属性在类中创建和访问文档字符串。
class Car:
"""
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
"""
def __init__(self, manufacturer, model, color):
"""
Constructs all the attributes for the car object.
Parameters
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
"""
self.model = model
self.color = color
self.manufacturer = manufacturer
def display_info(self, color, model, manufacturer):
"""
Prints the model of the car its color and the manufacturer.
Parameters
----------
model : str
color : str
manufacture: str
Returns
-------
None
"""
print(f"The {self.color} {self.model} is manufactured by {self.manufacturer}")
print(Car.__doc__)
help(Car)
输出:
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
Help on class Car in module __main__:
class Car(builtins.object)
| Car(manufacturer, model, color)
|
| A class to represent a car.
|
| ...
|
| Attributes
| ----------
虽然 Python 文档字符串在帮助开发人员理解他们的代码方面似乎扮演着类似的角色,但解释器会忽略注释。在 Python 中,单行注释前面有一个哈希符号,并且不能超过一行。
尽管多行注释也写在三双或三单引号内,但注释通常不遵循特定的结构。与 docstrings 似乎根据使用环境的不同而略有不同的格式不同,另一方面,无论程序如何,注释通常都以相同的方式使用。
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn