Python で Docstring にアクセスする
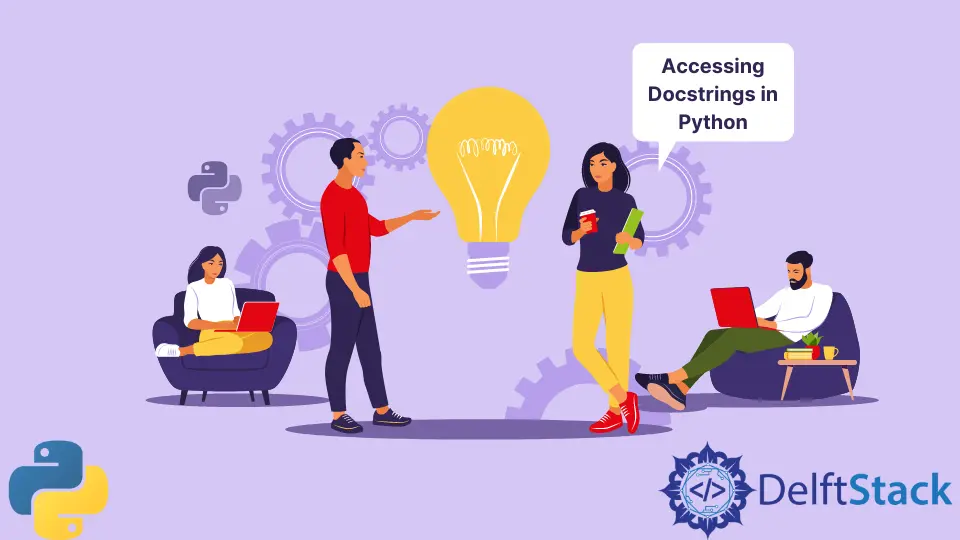
Python の用語集によると、docstring は、クラス、メソッド、または関数を定義した直後に表示される最初の文字列リテラルです。
オブジェクトの定義後に定義された Docstring は、多くの場合、その特定のオブジェクトに関連付けられており、print または help()
関数と一緒に __doc__
属性を使用してアクセスできます。Docstring は通常、以下に示すように、文字列リテラルをトリプルシングルまたはトリプルダブルの引用符で囲むことによって定義されます。
def addtwo(x):
"""Takes in a number x and adds two to it."""
return x + 2
print(addtwo.__doc__)
出力:
Takes in a number x and adds two to it.
クリーンなコードを作成するためのベストプラクティスの 1つは、特定の関数またはモジュールがどのように機能するかを説明する docstring を提供することです。開発者に重要な情報を提供するだけでなく、Sphinx docstring を使用して抽出し、プレーンテキスト、HTML、ePub、PDF などのさまざまな形式で美しいドキュメントを作成できます。
Docstring は、大きく 2つのカテゴリに分類されます。
- 単一行
- マルチライン
Python 単一行 Docstrings
従来、docstring は、開始と終了の両方のトリプルシングルまたはトリプルダブルが同じ行にある場合にのみ、単一行と見なされます。
多くの場合、1 行は同様の形式に従い、説明が少なくなります。代わりに、オブジェクトの機能とその戻り値について簡単に説明します。
さらに、1 行のドキュメント文字列には先頭に空白を入れないでください。常に大文字で始め、終止符で終わりを示す必要があります。以下に示すように、__doc__
属性を使用して、単一行の docstring にアクセスすることもできます。
def square(x):
"""Takes in a number x and returns its square."""
return x ** 2
print(square(10))
print(square.__doc__)
出力:
100
Takes in a number x and adds two to it.
Python マルチライン Docstrings
同様に、複数行の docstring も、トリプルシングルまたはトリプルダブルの引用符で囲まれた文字列リテラルによって定義されます。ただし、複数行のドキュメント文字列は通常、異なる構造または形式に従います。
複数行の docstring は通常、複数の行にまたがっています。最初の行は通常、オブジェクトの簡単な説明のみを提供するためのものです。
この説明の後には、1 行の空白行と、パラメーターのより詳細な説明(存在する場合)が続き、後続の行でオブジェクトの引数を返します。scikit learn
や pandas
などの大規模なライブラリには、そのライブラリ内で利用可能なパッケージを一覧表示するセクションも含まれています。
複数行の docstring は一般的に同様の構造を持っていますが、いくつかの違いはオブジェクトタイプによって異なります。関数オブジェクトでは、docstring は以下の構造に従います。
def add_numbers(x, y):
"""
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
"""
sum = x + y
return sum
print(add_numbers.__doc__)
出力:
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
Scikit
、NumPy
、Pandas
などの大規模なモジュールでは、docstring は以下の形式に従います。
後で説明するように、help()
関数と __doc__
属性を使用して docstring にアクセスすることもできます。__doc__
属性を使用して、以下のようなモジュールの docstring にアクセスできます。
import pandas as pd
print(pd.__doc__)
出力:
クラスの下に作成された Docstring は、クラスの機能を記述し、その特定のクラスのすべてのインスタンス変数とすべてのパブリックメソッドをリストする必要があります。サブクラスとも呼ばれるメインクラスから継承するクラスには、個別にアクセスできる docstring が必要です。
以下に示すように、docstring は、__doc___
属性を使用してクラスで作成およびアクセスできます。
class Car:
"""
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
"""
def __init__(self, manufacturer, model, color):
"""
Constructs all the attributes for the car object.
Parameters
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
"""
self.model = model
self.color = color
self.manufacturer = manufacturer
def display_info(self, color, model, manufacturer):
"""
Prints the model of the car its color and the manufacturer.
Parameters
----------
model : str
color : str
manufacture: str
Returns
-------
None
"""
print(f"The {self.color} {self.model} is manufactured by {self.manufacturer}")
print(Car.__doc__)
help(Car)
出力:
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
Help on class Car in module __main__:
class Car(builtins.object)
| Car(manufacturer, model, color)
|
| A class to represent a car.
|
| ...
|
| Attributes
| ----------
Python docstring は、開発者がコードを理解するのを支援する上で同様の役割を果たしているように見えますが、コメントはインタプリタによって無視されます。Python では、1 行のコメントの前にハッシュ記号を付け、複数行にすることはできません。
複数行コメントもトリプルダブルまたはトリプルシングル引用符で囲まれていますが、コメントは通常、特定の構造に従いません。使用状況によってフォーマットが若干異なるように見える docstring とは異なり、コメントはプログラムに関係なく一般的に同じように使用されます。
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn