파이썬에서 독스트링 접근하기
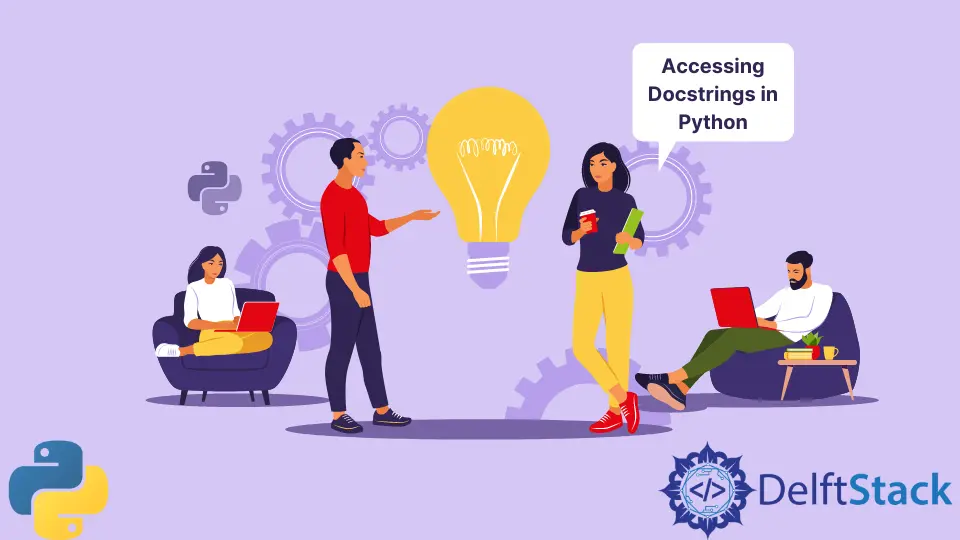
Python 용어집에 따르면 docstring은 클래스, 메서드 또는 함수를 정의한 직후에 나타나는 첫 번째 문자열 리터럴입니다.
객체 정의 후에 정의된 Docstring은 종종 해당 특정 객체와 연관되며 인쇄 또는 help()
함수와 함께 __doc__
속성을 사용하여 액세스할 수 있습니다. 독스트링은 일반적으로 아래와 같이 3중 작은 따옴표 또는 3중 큰 따옴표로 문자열 리터럴을 둘러싸서 정의됩니다.
def addtwo(x):
"""Takes in a number x and adds two to it."""
return x + 2
print(addtwo.__doc__)
출력:
Takes in a number x and adds two to it.
깨끗한 코드를 작성하기 위한 모범 사례 중 하나는 특정 기능이나 모듈이 작동하는 방식을 설명하는 독스트링을 제공하는 것입니다. 개발자에게 중요한 정보를 제공하는 것 외에도 Sphinx를 사용하여 독스트링을 추출하여 일반 텍스트, HTML, ePub 또는 PDF와 같은 다양한 형식의 아름다운 문서를 만들 수 있습니다.
독스트링은 크게 두 가지 범주로 분류됩니다.
- 하나의 선
- 여러 줄
파이썬 한 줄 독스트링
일반적으로 독스트링은 여는 트리플 싱글 또는 트리플 더블이 같은 줄에 있는 경우에만 한 줄로 간주됩니다.
한 줄은 종종 유사한 형식을 따르고 덜 설명적입니다. 대신 객체가 하는 일과 반환 값에 대한 간단한 설명을 제공합니다.
또한 한 줄 문서 문자열에는 선행 공백이 없어야 하며 항상 대문자로 시작하고 끝을 표시하는 마침표로 시작해야 합니다. __doc__
속성은 아래와 같이 한 줄 독스트링에 액세스하는 데 사용할 수도 있습니다.
def square(x):
"""Takes in a number x and returns its square."""
return x ** 2
print(square(10))
print(square.__doc__)
출력:
100
Takes in a number x and adds two to it.
파이썬 여러 줄 독스트링
유사하게, 여러 줄 독스트링은 삼중-작은따옴표 또는 삼중-큰 따옴표 안에 문자열 리터럴을 둘러싸서 정의됩니다. 그러나 여러 줄로 된 문서 문자열은 일반적으로 다른 구조나 형식을 따릅니다.
여러 줄 독스트링은 일반적으로 둘 이상의 줄에 걸쳐 있습니다. 첫 번째 줄은 일반적으로 개체에 대한 간단한 설명만 제공하는 데 사용됩니다.
이 설명 뒤에는 한 줄의 빈 줄과 매개 변수에 대한 자세한 설명(있는 경우)이 오고 다음 줄에는 개체의 인수가 반환됩니다. scikit learn
또는 pandas
와 같은 대규모 라이브러리에는 해당 라이브러리 내에서 사용 가능한 패키지를 나열하는 섹션도 포함되어 있습니다.
여러 줄 독스트링은 일반적으로 유사한 구조를 갖지만 일부 차이점은 개체 유형에 따라 다릅니다. 함수 객체에서 독스트링은 아래 구조를 따릅니다.
def add_numbers(x, y):
"""
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
"""
sum = x + y
return sum
print(add_numbers.__doc__)
출력:
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
Scikit
, NumPy
또는 Pandas
와 같은 대형 모듈에서 독스트링은 아래 형식을 따릅니다.
우리는 또한 help()
함수와 __doc__
속성을 사용하여 나중에 보게 되겠지만 독스트링에 액세스할 수 있습니다. __doc__
속성을 사용하여 아래와 같은 모듈의 독스트링에 액세스할 수 있습니다.
import pandas as pd
print(pd.__doc__)
출력:
클래스 아래에 생성된 독스트링은 클래스의 기능을 명시하고 해당 특정 클래스의 모든 인스턴스 변수와 모든 공용 메서드를 나열해야 합니다. 서브클래스라고도 하는 기본 클래스에서 상속하는 클래스에는 개별적으로 액세스할 수 있는 독스트링이 있어야 합니다.
아래와 같이 __doc__
속성을 사용하여 클래스에서 독스트링을 만들고 액세스할 수 있습니다.
class Car:
"""
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
"""
def __init__(self, manufacturer, model, color):
"""
Constructs all the attributes for the car object.
Parameters
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
"""
self.model = model
self.color = color
self.manufacturer = manufacturer
def display_info(self, color, model, manufacturer):
"""
Prints the model of the car its color and the manufacturer.
Parameters
----------
model : str
color : str
manufacture: str
Returns
-------
None
"""
print(f"The {self.color} {self.model} is manufactured by {self.manufacturer}")
print(Car.__doc__)
help(Car)
출력:
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
Help on class Car in module __main__:
class Car(builtins.object)
| Car(manufacturer, model, color)
|
| A class to represent a car.
|
| ...
|
| Attributes
| ----------
Python 독스트링은 개발자가 코드를 이해하는 데 도움이 되는 유사한 역할을 수행하는 것처럼 보이지만 인터프리터는 주석을 무시합니다. 파이썬에서 한 줄 주석은 해시 기호 앞에 오고 한 줄 이상일 수 없습니다.
여러 줄 주석도 삼중 이중 또는 삼중 작은 따옴표 안에 작성되지만 주석은 일반적으로 특정 구조를 따르지 않습니다. 사용되는 상황에 따라 약간 다른 형식을 갖는 것으로 보이는 독스트링과 달리 주석은 일반적으로 프로그램에 관계없이 동일한 방식으로 사용됩니다.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn