在 Python 中訪問文件字串
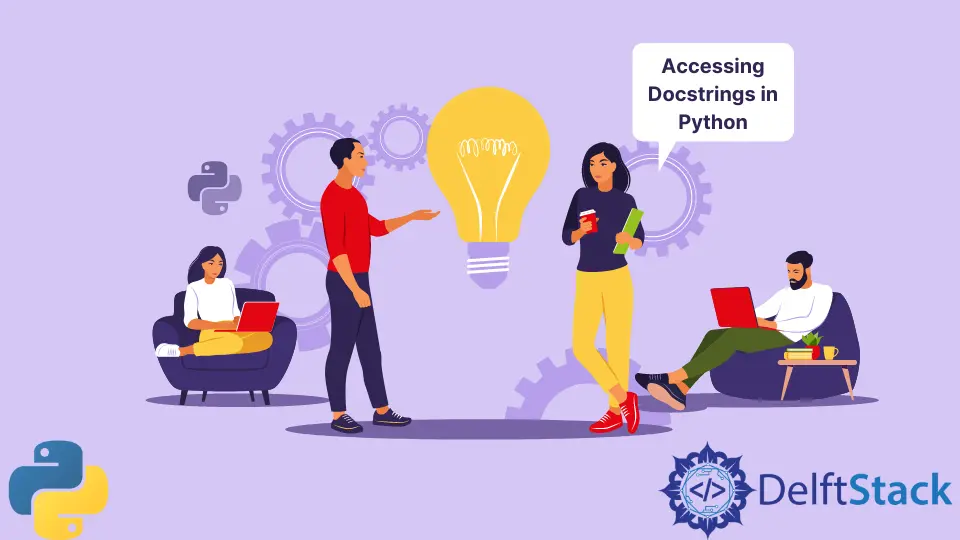
根據 python 詞彙表,docstring 是在定義類、方法或函式後立即出現的第一個字串文字。
在任何物件的定義之後定義的文件字串通常與該特定物件相關聯,並且可以使用 __doc__
屬性與 print 或 help()
函式一起訪問。文件字串通常通過將字串文字包含在三重單引號或三重雙引號中來定義,如下所示:
def addtwo(x):
"""Takes in a number x and adds two to it."""
return x + 2
print(addtwo.__doc__)
輸出:
Takes in a number x and adds two to it.
編寫乾淨程式碼的最佳實踐之一是提供描述特定函式或模組如何工作的文件字串。除了向開發人員提供重要資訊外,還可以提取 Sphinx 文件字串以建立不同格式的精美文件,例如純文字、HTML、ePub 或 PDF。
文件字串主要分為兩類:
- 單線
- 多線
Python 單行文件字串
按照慣例,只有當開始和結束三重單或三重雙在同一行上時,文件字串才被視為單行。
單行通常遵循類似的格式並且不太具有描述性。相反,它們提供了物件的作用及其返回值的簡短說明。
此外,單行文件字串不應有前導空格,並且應始終以大寫字母開頭並以句號結尾。__doc__
屬性也可用於訪問單行文件字串,如下所示。
def square(x):
"""Takes in a number x and returns its square."""
return x ** 2
print(square(10))
print(square.__doc__)
輸出:
100
Takes in a number x and adds two to it.
Python 多行文件字串
類似地,多行文件字串也通過將字串文字包含在三重單引號或三重雙引號中來定義。但是,多行文件字串通常遵循不同的結構或格式。
多行文件字串通常跨越多行。第一行通常專用於僅提供物件的簡短描述。
此描述後跟一個空行和更詳細的引數描述(如果有),並在後續行中返回物件的引數。大型庫,如 scikit learn
或 pandas
還包括一個部分,列出該庫中可用的包。
儘管多行文件字串通常具有相似的結構,但某些差異取決於物件型別。在函式物件中,文件字串將遵循以下結構。
def add_numbers(x, y):
"""
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
"""
sum = x + y
return sum
print(add_numbers.__doc__)
輸出:
Function takes to arguments and returns the sum of the two
Parameter:
x (int): An integer
y (int): Another integer
Returns:
sum(int): Returns an integer sum of x and y
在諸如 Scikit
、NumPy
或 Pandas
等大型模組中,文件字串遵循以下格式。
我們還可以使用 help()
函式和 __doc__
屬性來訪問文件字串,我們將在後面看到。我們可以使用 __doc__
屬性來訪問模組中的文件字串,如下所示。
import pandas as pd
print(pd.__doc__)
輸出:
在類下建立的文件字串應該說明類的功能,列出該特定類的所有例項變數以及所有公共方法。從主類繼承的類,也稱為子類,應該有可以單獨訪問的文件字串。
如下所示,可以使用 __doc___
屬性在類中建立和訪問文件字串。
class Car:
"""
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
"""
def __init__(self, manufacturer, model, color):
"""
Constructs all the attributes for the car object.
Parameters
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
"""
self.model = model
self.color = color
self.manufacturer = manufacturer
def display_info(self, color, model, manufacturer):
"""
Prints the model of the car its color and the manufacturer.
Parameters
----------
model : str
color : str
manufacture: str
Returns
-------
None
"""
print(f"The {self.color} {self.model} is manufactured by {self.manufacturer}")
print(Car.__doc__)
help(Car)
輸出:
A class to represent a car.
...
Attributes
----------
manufacturer : str
company that manufactured the car
model : str
model of the car
color : str
color of the car
Methods
-------
display_info():
Prints out the car manufacturer, model and color
Help on class Car in module __main__:
class Car(builtins.object)
| Car(manufacturer, model, color)
|
| A class to represent a car.
|
| ...
|
| Attributes
| ----------
雖然 Python 文件字串在幫助開發人員理解他們的程式碼方面似乎扮演著類似的角色,但直譯器會忽略註釋。在 Python 中,單行註釋前面有一個雜湊符號,並且不能超過一行。
儘管多行註釋也寫在三雙或三單引號內,但註釋通常不遵循特定的結構。與 docstrings 似乎根據使用環境的不同而略有不同的格式不同,另一方面,無論程式如何,註釋通常都以相同的方式使用。
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn