How to Delete Line From File in Python
- Use Line Number to Delete a Specific Line From a File in Python
- Deleting the First or the Last Line From a File in Python
- Delete a Line That Matches a Given Particular Text
- Delete a Line That Matches a Given Particular Word
- Delete the Shortest Line in the Given File in Python
- Delete All Lines From a Given Particular File in Python
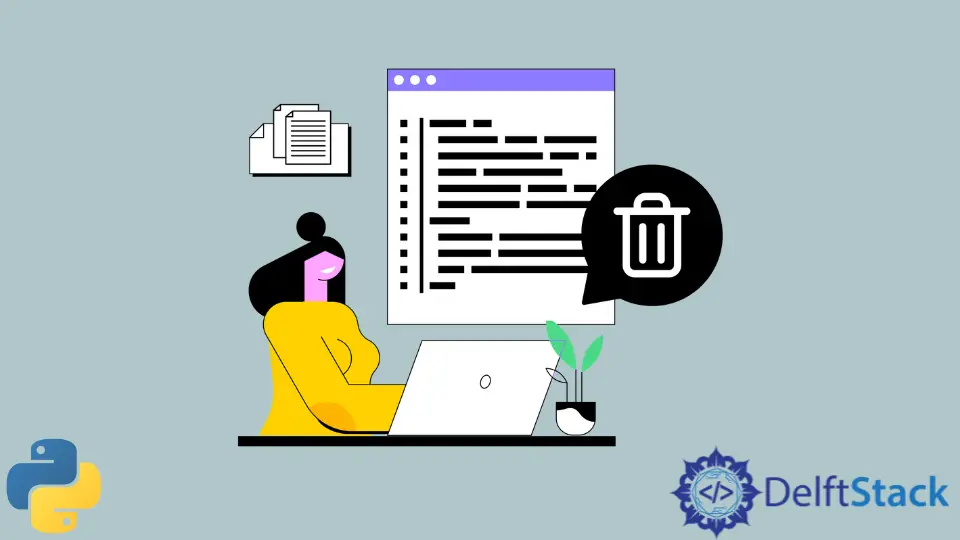
Data file handling is supported and allowed by Python, and it forms an essential part of the Python programming language. However, there aren’t any direct functions in Python that can delete a specific line in a given file.
This tutorial demonstrates the different ways available to delete a specific line from a file in Python.
This task of deleting a specific line can be implemented with the help of several different approaches.
Use Line Number to Delete a Specific Line From a File in Python
Method 1
This method, as specified above, utilizes the line number specified by the user to delete a line from a particular file in Python.
It makes use of the for
loop, the readlines()
method, and the enumerate()
method.
Let us take an example file, named test.txt
, whose content is detailed below.
Hello
My name is
Adam
I am
a
good
singer
cricketer dancer
The following code uses the enumerate()
and readlines()
methods to delete a line from a particular file in Python.
l1 = []
with open("temp.txt", "r") as fp:
l1 = fp.readlines()
with open("temp.txt", "w") as fp:
for number, line in enumerate(l1):
if number not in [4, 6]:
fp.write(line)
The above code makes the following changes:
Hello
My name is
Adam
I am
good
cricketer dancer
Code Explanation:
- First, the file is opened in
read
mode. - The content from the given file is then read into a list with the help of the
readlines()
function. - The file is then closed.
- The file is then again opened, but this time in the
write
mode. - Then, a
for
loop and theenumerate()
function are implemented to the created list. - The
if
condition is utilized in checking and selecting the line number. The line number mentioned is deleted. - The file is then closed.
Method 2
The seek()
method can also be utilized to implement the same task of deleting a line from a file using a line number. We will work on the same file, test.txt
, as mentioned in the method above.
By utilizing the seek()
method, we need not open the same file twice, making it easier and faster.
The following code uses the seek()
method to delete a line from a particular file in Python.
with open("temp.txt", "r+") as fp:
lines = fp.readlines()
fp.seek(0)
fp.truncate()
for number, line in enumerate(lines):
if number not in [4, 6]:
fp.write(line)
The above code makes the following changes:
Hello
My name is
Adam
I am
good
cricketer dancer
Code Explanation:
- The file is opened in the
r+
mode, which allows both reading and writing capabilities. - The file content is then read into a list with the help of the
readlines()
function. - The
seek()
method is then utilized to move the pointer back to the starting point of the list. - The file is then truncated with the help of the
truncate()
method. - Then, a
for
loop and theenumerate()
function are implemented to the created list. - The
if
condition is utilized in checking and selecting the line number. The line number mentioned is deleted. - The file is closed.
Deleting the First or the Last Line From a File in Python
To implement this method, we utilize list slicing while writing the contents of the file into a list.
For example, we will delete the first line from the same file mentioned above (test.txt
).
The following code deletes the first line from a given file in Python.
with open("temp.txt", "r+") as fp:
lines = fp.readlines()
fp.seek(0)
fp.truncate()
fp.writelines(lines[1:])
The above code makes the following changes and provides the following output:
My name is
Adam
I am
a
good
singer
cricketer dancer
Code Explanation:
- The file is opened in the
r+
mode, which allows both reading and writing capabilities. - The file content is read into a list with the help of the
readlines()
function. - The
seek()
method is then utilized to move the pointer back to the starting point of the list. - The file is then truncated with the help of the
truncate()
method. - All the lines from the file are then written to a list with the exception of the first line. This is made possible with the help of list slicing.
Delete a Line That Matches a Given Particular Text
This method can be utilized when there are multiple lines that contain a specific text. The lines that match the given text can then be deleted.
For example, we will delete a line that matches a given particular text from the same file (test.txt
) that is being utilized in the above methods.
This method utilizes the strip()
function and the write()
function to implement the task of deleting a line containing some given text.
The following code deletes a line that matches a given particular string in Python.
content = "cricketer dancer"
with open("temp.txt", "r+") as fp:
lines = fp.readlines()
fp.seek(0)
fp.truncate()
for line in lines:
if line.strip("\n") != content:
fp.writelines(line)
The above code makes the following changes and provides the following output:
Hello
My name is
Adam
I am
a
good
singer
Delete a Line That Matches a Given Particular Word
Similar to deleting a line by matching the entire string, we can also try and find a word that might be contained in a line and then delete that particular line.
For example, we will delete a line that matches a given particular word from the same file (test.txt
) that is being utilized in the above methods.
For this method, we utilize the os
module that Python provides. We also create another new file and write the data in it to implement this task.
The following code deletes a line that matches a given particular word in Python.
import os
with open("temp.txt", "r") as input:
with open("bb.txt", "w") as output:
for line in input:
if "cricketer" not in line.strip("\n"):
output.write(line)
os.replace("bb.txt", "temp.txt")
The above code makes the following changes and provides the following output:
Hello
My name is
Adam
I am
a
good
singer
Delete the Shortest Line in the Given File in Python
As its name suggests, this method finds and deletes the shortest line of the given file. Here, we will make use of the len()
method to implement this task.
For example, we will delete the shortest line from the same file (test.txt
) utilized in the above methods.
The following code deletes the shortest line in the given file in Python.
with open("temp.txt", "r") as rf:
lines = rf.readlines()
shortest = 1000
lineToDelete = ""
for line in lines:
if len(line) < shortest:
shortest = len(line)
lineToDelete = line
with open("temp.txt", "w") as write_file:
for line in lines:
if line == lineToDelete:
pass
else:
write_file.write(line)
Delete All Lines From a Given Particular File in Python
To delete all the lines of a particular file in Python, we can use the truncate()
function. Moreover, the file pointer is then set back to the inception of the file.
The following code deletes all lines from a given particular file in Python.
with open("temp.txt", "w+") as fp:
fp.truncate()
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn