How to Convert Seconds Into Hours, Minutes, and Seconds in Python
- Make a Custom Function Using Mathematical Calculations to Convert Seconds Into Hours, Minutes, and Seconds in Python
-
Use the
divmod()
Function to Convert Seconds Into Hours, Minutes, and Seconds in Python - Use the DateTime Module to Convert Seconds Into Hours, Minutes, and Seconds in Python
- Use the Time Module to Convert Seconds Into Hours, Minutes, and Seconds in Python
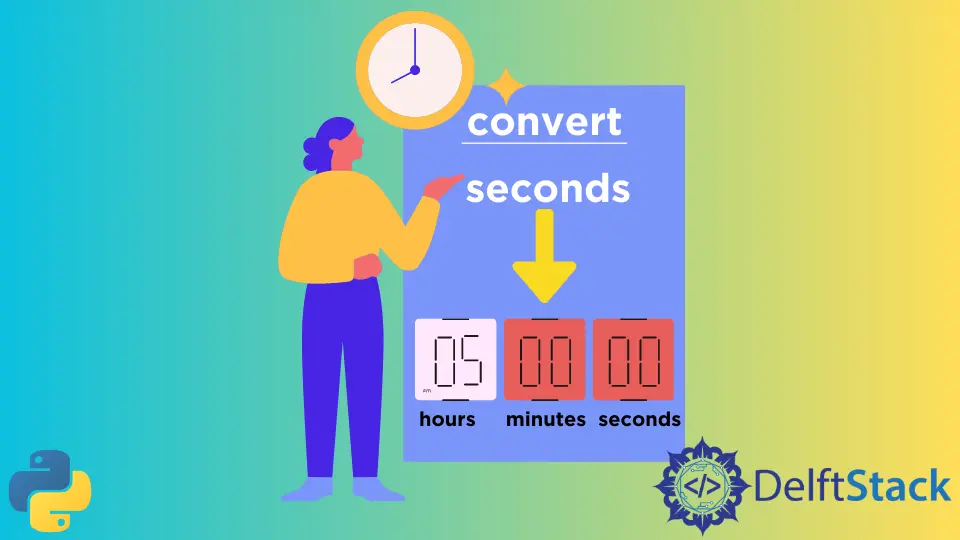
This tutorial will discuss using, managing, and converting seconds into days, hours, minutes, and seconds using four different methods in Python.
Now, let’s start by discussing these methods and using them in some examples.
Make a Custom Function Using Mathematical Calculations to Convert Seconds Into Hours, Minutes, and Seconds in Python
This method will store the seconds we want to convert into a variable. Now we will divide the seconds to get hours, minutes, and seconds as shown below.
Example:
# python
SecToConvert = 56000
RemainingSec = SecToConvert % (24 * 3600)
HoursGet = RemainingSec // 3600
RemainingSec %= 3600
MinutesGet = RemainingSec // 60
print("%d:%02d:%02d" % (HoursGet, MinutesGet, RemainingSec))
Output:
As you can see in the example, we can easily convert seconds into hours, minutes, and seconds using simple mathematical calculations.
Use the divmod()
Function to Convert Seconds Into Hours, Minutes, and Seconds in Python
The divmod()
function can convert seconds into hours, minutes, and seconds. The divmod()
accepts two integers as parameters and returns a tuple containing the quotient and remainder of their division.
We can also use divmod()
in many other mathematical conditions like checking for divisibility of numbers and whether the number is prime. Now, let’s use this function to convert the seconds, as shown below.
Example:
# python
SecToConvert = 56000
MinutesGet, SecondsGet = divmod(SecToConvert, 60)
HoursGet, MinutesGet = divmod(MinutesGet, 60)
print("Total hours are: ", HoursGet)
print("Total minutes are: ", MinutesGet)
print("Total seconds are: ", SecondsGet)
Output:
As you can see from the example, it is much simpler than the custom method, and it takes lesser lines of code to perform complex functions.
Use the DateTime Module to Convert Seconds Into Hours, Minutes, and Seconds in Python
Python provides a DateTime module with classes and functions to manipulate dates and times. We can use these classes and functions to work with dates, times, and time intervals for various tasks.
The DateTime module offers the timedelta()
function to convert seconds into hours, minutes, and seconds. This function accepts the parameter seconds
and returns it in format (hours, minutes, and seconds).
Now, let’s use this function in our example to understand how it works, as shown below.
Example:
# python
import datetime
SecToConvert = 56000
ConvertedSec = str(datetime.timedelta(seconds=SecToConvert))
print("Converted Results are: ", ConvertedSec)
Output:
As you can see in the example, using the DateTime module is much faster and quicker than the divmod()
function. The DateTime module provides the format, which helps automate the task efficiently.
Use the Time Module to Convert Seconds Into Hours, Minutes, and Seconds in Python
Python provides another module, Time, with features to express time in code, including objects and integers. This module also provides a function for a wait during the processes.
The strftime()
function in the Time module can convert the given seconds into a time format, such as hours, minutes, and seconds. Another function time.gmtime()
is taken as an argument.
strftime()
outputs the seconds in the required format, and gmtime()
converts seconds to the required format required by the strftime()
function. Now, let’s use the Time module to convert the seconds, as shown below.
Example:
# python
import time
SecToConvert = 56000
Convertedformat = time.strftime("%H:%M:%S", time.gmtime(SecToConvert))
print("After converting the seconds :", Convertedformat)
Output:
In the example, we can also use the Time module to convert the seconds into hours, minutes, and seconds. Like the DateTime module, the Time module also converts the seconds into time format, but we can specify the format.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn