Constant in Python
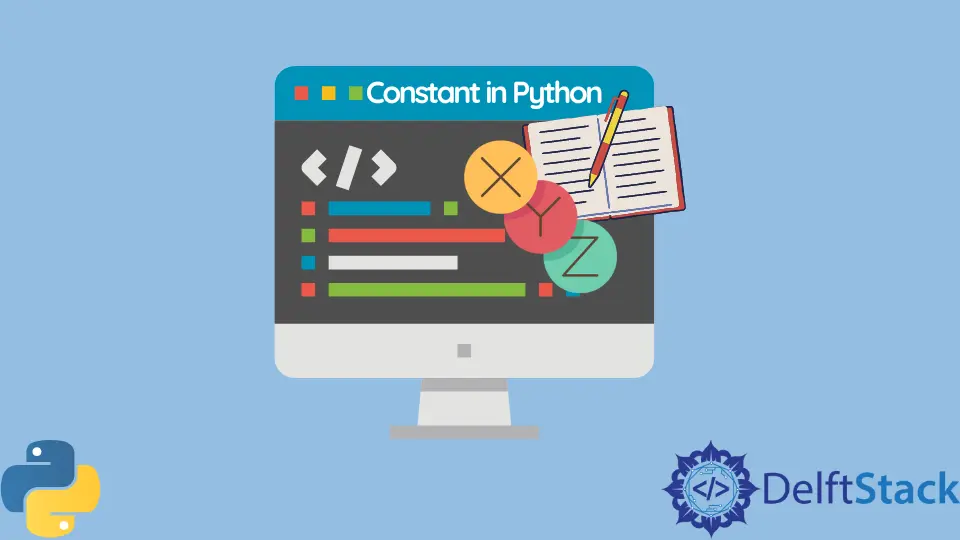
In the world of programming, constants are values that remain unchanged throughout the execution of a program. In Python, while there isn’t a built-in constant type, developers often use naming conventions to indicate that a variable should be treated as a constant.
This tutorial will explore different methods for creating constants in Python, providing you with practical examples and clear explanations. Whether you’re a beginner or an experienced programmer, understanding how to implement constants effectively can enhance your code’s readability and maintainability. Let’s dive into the various approaches to creating constants in Python!
Using Naming Conventions
One of the simplest and most common methods to define a constant in Python is by following naming conventions. By convention, constants are typically named using all uppercase letters with underscores separating words. This approach signals to other developers that these variables should not be modified.
Here’s an example:
PI = 3.14159
GRAVITY = 9.81
SPEED_OF_LIGHT = 299792458
In this code snippet, we have defined three constants: PI
, GRAVITY
, and SPEED_OF_LIGHT
. By using uppercase letters, we make it clear that these values should remain unchanged throughout the program. This method relies on the discipline of the developers to avoid altering these values, as Python does not enforce immutability.
Using this naming convention is straightforward and effective. It enhances code readability since anyone reading the code can easily identify which variables are meant to be constants. However, it’s worth noting that this method does not prevent accidental reassignment. Therefore, while it’s a widely accepted practice, it requires a level of responsibility from the developer.
Creating Constants Using a Class
Another approach to creating constants in Python is by using a class. By defining constants within a class, you can encapsulate them and prevent accidental changes. This method is particularly useful when you want to group related constants together.
Here’s how you can do it:
class Constants:
PI = 3.14159
GRAVITY = 9.81
SPEED_OF_LIGHT = 299792458
In this example, we define a class named Constants
that contains three class attributes: PI
, GRAVITY
, and SPEED_OF_LIGHT
. By accessing these constants through the class, such as Constants.PI
, you can ensure that they are treated as constants.
Using a class to store constants has several advantages. It provides a clear structure for organizing constants, especially when dealing with a large number of them. Additionally, it minimizes the risk of accidental reassignment since class attributes can be made read-only by overriding the __setattr__
method, though this requires a bit more coding.
This method also enhances code readability, as developers can easily see the context in which the constants are used. Overall, using a class for constants is a robust approach, particularly for larger projects where organization is key.
Using Enums for Constants
Python’s enum
module provides a powerful way to define constants, especially when working with a fixed set of related values. Enums can enhance code clarity and prevent invalid values from being assigned, making them an excellent choice for constants.
Here’s an example of how to use enums:
from enum import Enum
class Color(Enum):
RED = 1
GREEN = 2
BLUE = 3
In this code, we define an enum called Color
with three members: RED
, GREEN
, and BLUE
. Each member is assigned a unique value. You can access these constants using Color.RED
, Color.GREEN
, and Color.BLUE
.
Using enums for constants offers several benefits. First, it provides a clear and descriptive way to represent a set of related constants. Second, enums prevent the accidental assignment of invalid values, as you can only use the defined members. This makes your code more robust and less prone to errors.
Enums also improve code readability. When you see Color.RED
, it’s immediately clear that this refers to a specific color constant, enhancing the self-documenting nature of your code. Overall, using enums is a modern and effective way to define constants in Python, especially when working with groups of related values.
Conclusion
In this tutorial, we explored various methods for creating constants in Python. From using naming conventions to defining constants within classes and utilizing enums, each method has its own advantages and can be chosen based on the specific needs of your project. By implementing these practices, you can enhance your code’s readability, maintainability, and robustness. Remember, while Python does not enforce constant values, following these conventions and methods can help you and your team write cleaner and more effective code. Happy coding!
FAQ
-
What is a constant in Python?
A constant in Python refers to a value that is intended to remain unchanged throughout the execution of a program. -
Can Python enforce constants?
No, Python does not have built-in support for constants, but developers use naming conventions and other methods to indicate which variables should be treated as constants. -
What is the best way to define constants in Python?
The best way depends on your needs. Using naming conventions is simple, while using classes or enums can provide better organization and prevent accidental changes. -
Are constants in Python truly immutable?
No, constants in Python are not enforced as immutable. Developers must adhere to conventions to avoid modifying them. -
Can I define constants in a module?
Yes, you can define constants in a module using naming conventions, and they will be accessible when you import the module.