Python의 상수
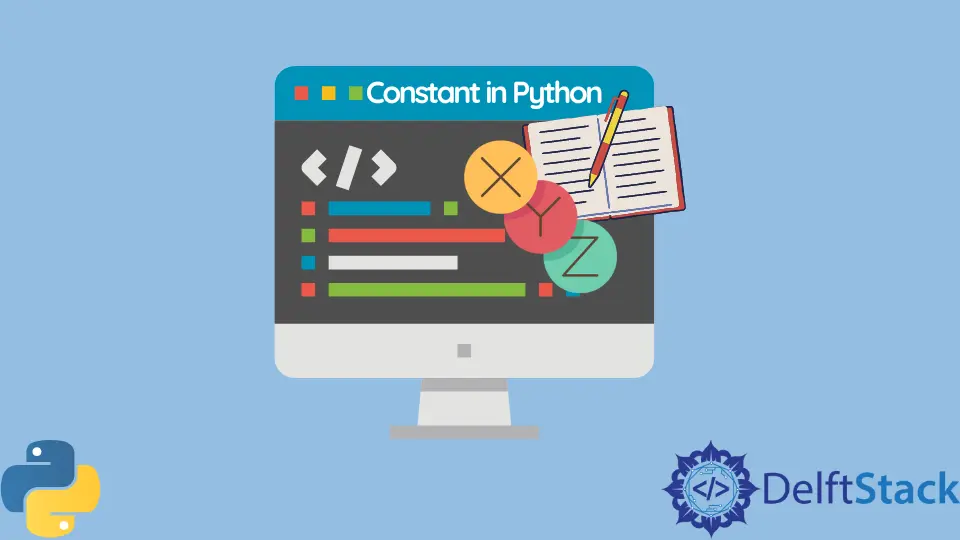
이 튜토리얼에서는 Python에서 상수를 생성하거나 상수와 유사한 속성을 달성하는 여러 방법을 소개합니다. const
와 같은 키워드가 없기 때문에 Python에서는 상수를 선언할 수 없습니다. 그러나 다음과 같은 방법으로 상수를 생성할 수 있습니다.
클래스를 정의하여 파이썬 상수
한 가지 방법은 클래스를 만들고 해당 속성을 상수로 사용할 읽기 전용으로 선언하는 것입니다. 아래 예제 코드는 Python에서 class 속성을 읽기 전용으로 선언하는 방법을 보여줍니다.
class constant(object):
__slots__ = ()
val = 457
a = constant()
print(a.val)
a.val = 23
출력:
457
AttributeError: 'constant' object attribute 'val' is read-only
파이썬에서 상수를 만드는 또 다른 방법은 클래스를 만들고 __setattr__(self, *_)
을 재정의하여 누군가가 클래스 속성에 새 값을 할당하려고 할 때마다 예외를 반환하도록 하는 것입니다.
다음과 같은 방법으로 Python에서 클래스 속성을 상수로 사용할 수 있습니다.
class Constant(object):
CONST = "abcd"
def __setattr__(self, *_):
raise Exception("Tried to change the value of a constant")
a = Constant()
print(a.CONST)
a.CONST_NAME = "a"
출력:
abcd
Exception: Tried to change the value of a constant
함수를 사용하는 파이썬 상수
상수에 할당하려는 동일한 값을 항상 반환하는 함수를 만들 수도 있습니다. 아래 예제 코드는 함수를 사용하여 Python에서 상수 값을 가져오는 방법을 보여줍니다.
def constant():
return "xyz"
constant()
출력:
xyz
namedtuple()
함수를 사용하는 Python 상수
또한 collections
모듈의 namedtuple(typename, field_names)
함수를 사용하여 Python에서 상수를 생성할 수도 있습니다. namedtuple()
함수는 typename
이름의 새 튜플 하위 클래스를 반환하고 field_names
는 문자열 식별자 목록입니다. 파이썬에서와 같이 튜플은 불변이며, 새로운 튜플 서브클래스의 값은 상수로 사용될 수 있도록 변경할 수 없습니다.
아래 예제는 namedtuple()
함수를 사용하여 Python에서 상수를 만드는 방법을 보여줍니다.
from collections import namedtuple
my_consts = namedtuple("Constants", ["const1", "const2"])
consts = my_consts(54, "abc")
print(consts.const1)
print(consts.const2)
출력:
54
abc
이제 튜플의 값을 변경해 보겠습니다.
from collections import namedtuple
my_consts = namedtuple("Constants", ["const1", "const2"])
consts = my_consts(54, "abc")
consts.const1 = 0
출력:
AttributeError: can't set attribute