Python の定数
Muhammad Waiz Khan
2023年1月30日
Python
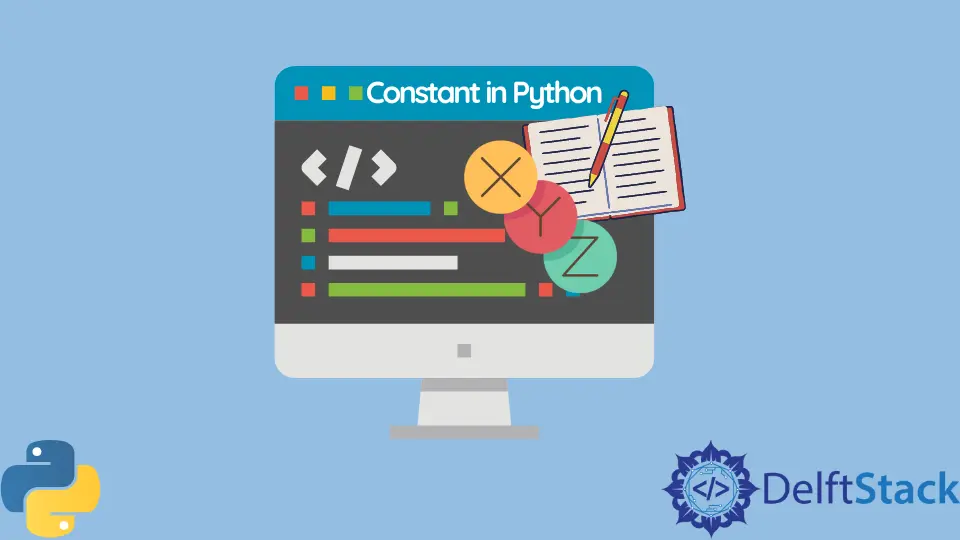
このチュートリアルでは、Python で定数を作成したり、定数のようなプロパティを実現したりするための複数のメソッドを紹介します。const
としてのキーワードがないため、Python で定数を宣言することはできません。ただし、次の方法で独自の定数を作成できます。
クラスを定義することによる Python 定数
1つの方法は、クラスを作成し、その属性を読み取り専用として宣言して、定数として使用することです。以下のサンプルコードは、Python でクラス属性を読み取り専用として宣言する方法を示しています。
class constant(object):
__slots__ = ()
val = 457
a = constant()
print(a.val)
a.val = 23
出力:
457
AttributeError: 'constant' object attribute 'val' is read-only
Python で定数を作成する別の方法は、クラスを作成し、__setattr__(self, *_)
をオーバーライドして、誰かがクラス属性に新しい値を割り当てようとするたびに例外を返すことです。
次の方法で、Python の定数としてクラス属性を使用できます。
class Constant(object):
CONST = "abcd"
def __setattr__(self, *_):
raise Exception("Tried to change the value of a constant")
a = Constant()
print(a.CONST)
a.CONST_NAME = "a"
出力:
abcd
Exception: Tried to change the value of a constant
関数を使用した Python 定数
定数に割り当てたいのと同じ値を常に返す関数を作成することもできます。以下のサンプルコードは、関数を使用して Python で定数値を取得する方法を示しています。
def constant():
return "xyz"
constant()
出力:
xyz
namedtuple()
関数を使用した Python 定数
collections
モジュールの namedtuple(typename, field_names)
関数を使用して、Python で定数を作成することもできます。namedtuple()
関数は、名前 typename
の新しいタプルサブクラスを返します。field_names
は、文字列識別子のリストです。Python と同様に、タプルは不変であり、新しいタプルサブクラスの値を変更して定数として使用することはできません。
以下の例は、namedtuple()
関数を使用して Python で定数を作成する方法を示しています。
from collections import namedtuple
my_consts = namedtuple("Constants", ["const1", "const2"])
consts = my_consts(54, "abc")
print(consts.const1)
print(consts.const2)
出力:
54
abc
それでは、タプルの値を変更してみましょう。
from collections import namedtuple
my_consts = namedtuple("Constants", ["const1", "const2"])
consts = my_consts(54, "abc")
consts.const1 = 0
出力:
AttributeError: can't set attribute
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe