Python 中的常量
Muhammad Waiz Khan
2023年1月30日
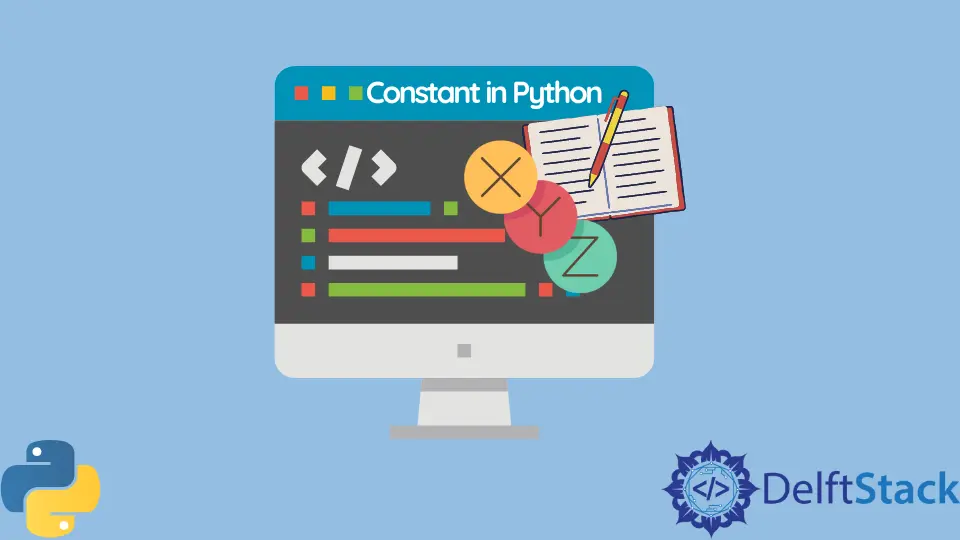
本教程将介绍在 Python 中创建常量或实现类常量属性的多种方法。我们不能在 Python 中声明一个常量,因为没有关键字 const
。但是我们可以通过以下方式创建我们自己的常量。
通过定义类来定义 Python 常量
一种方法是创建一个类并将其属性声明为只读以用作常量。下面的示例代码演示了如何在 Python 中将 class
属性声明为只读。
class constant(object):
__slots__ = ()
val = 457
a = constant()
print(a.val)
a.val = 23
输出:
457
AttributeError: 'constant' object attribute 'val' is read-only
在 Python 中创建常量的另一种方法是创建一个类并覆盖 __setattr__(self, *_)
以在有人尝试为类属性分配新值时返回异常。
我们可以通过以下方式在 Python 中使用类属性作为常量。
class Constant(object):
CONST = "abcd"
def __setattr__(self, *_):
raise Exception("Tried to change the value of a constant")
a = Constant()
print(a.CONST)
a.CONST_NAME = "a"
输出:
abcd
Exception: Tried to change the value of a constant
使用函数的 Python 常量
我们还可以创建一个函数,该函数始终返回我们想要分配给常量的相同值。下面的示例代码演示了如何使用该函数在 Python 中获取常量值。
def constant():
return "xyz"
constant()
输出:
xyz
使用 namedtuple()
函数的 Python 常量
我们还可以使用 collections
模块的 namedtuple(typename, field_names)
函数在 Python 中创建一个常量。namedtuple()
函数返回名称为 typename
的新元组子类,而 field_names
是字符串标识符列表。就像在 Python 中一样,元组是不可变的,新元组子类的值不能更改,因此它可以用作常量。
下面的示例演示了如何使用 namedtuple()
函数在 Python 中创建常量。
from collections import namedtuple
my_consts = namedtuple("Constants", ["const1", "const2"])
consts = my_consts(54, "abc")
print(consts.const1)
print(consts.const2)
输出:
54
abc
现在让我们尝试更改元组的值。
from collections import namedtuple
my_consts = namedtuple("Constants", ["const1", "const2"])
consts = my_consts(54, "abc")
consts.const1 = 0
输出:
AttributeError: can't set attribute