Python 中的常量
Muhammad Waiz Khan
2023年1月30日
Python
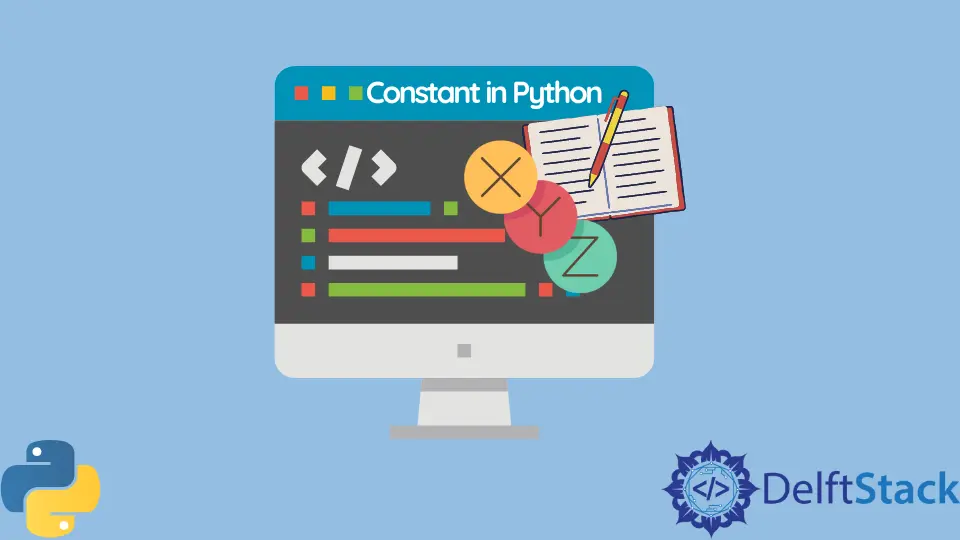
本教程將介紹在 Python 中建立常量或實現類常量屬性的多種方法。我們不能在 Python 中宣告一個常量,因為沒有關鍵字 const
。但是我們可以通過以下方式建立我們自己的常量。
通過定義類來定義 Python 常量
一種方法是建立一個類並將其屬性宣告為只讀以用作常量。下面的示例程式碼演示瞭如何在 Python 中將 class
屬性宣告為只讀。
class constant(object):
__slots__ = ()
val = 457
a = constant()
print(a.val)
a.val = 23
輸出:
457
AttributeError: 'constant' object attribute 'val' is read-only
在 Python 中建立常量的另一種方法是建立一個類並覆蓋 __setattr__(self, *_)
以在有人嘗試為類屬性分配新值時返回異常。
我們可以通過以下方式在 Python 中使用類屬性作為常量。
class Constant(object):
CONST = "abcd"
def __setattr__(self, *_):
raise Exception("Tried to change the value of a constant")
a = Constant()
print(a.CONST)
a.CONST_NAME = "a"
輸出:
abcd
Exception: Tried to change the value of a constant
使用函式的 Python 常量
我們還可以建立一個函式,該函式始終返回我們想要分配給常量的相同值。下面的示例程式碼演示瞭如何使用該函式在 Python 中獲取常量值。
def constant():
return "xyz"
constant()
輸出:
xyz
使用 namedtuple()
函式的 Python 常量
我們還可以使用 collections
模組的 namedtuple(typename, field_names)
函式在 Python 中建立一個常量。namedtuple()
函式返回名稱為 typename
的新元組子類,而 field_names
是字串識別符號列表。就像在 Python 中一樣,元組是不可變的,新元組子類的值不能更改,因此它可以用作常量。
下面的示例演示瞭如何使用 namedtuple()
函式在 Python 中建立常量。
from collections import namedtuple
my_consts = namedtuple("Constants", ["const1", "const2"])
consts = my_consts(54, "abc")
print(consts.const1)
print(consts.const2)
輸出:
54
abc
現在讓我們嘗試更改元組的值。
from collections import namedtuple
my_consts = namedtuple("Constants", ["const1", "const2"])
consts = my_consts(54, "abc")
consts.const1 = 0
輸出:
AttributeError: can't set attribute
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe