How to Compare Two Strings Character by Character in Python
-
Compare Strings Character by Character in Python Using a
for
Loop -
Compare Two Strings Character by Character in Python Using a
while
Loop -
Compare Two Strings Character by Character in Python Using the
==
Operator -
Compare Two Strings Character by Character in Python Using
all()
Withzip()
-
Compare Two Strings Character by Character in Python Using
enumerate()
-
Compare Two Strings Character by Character in Python Using
itertools.zip_longest()
- Conclusion
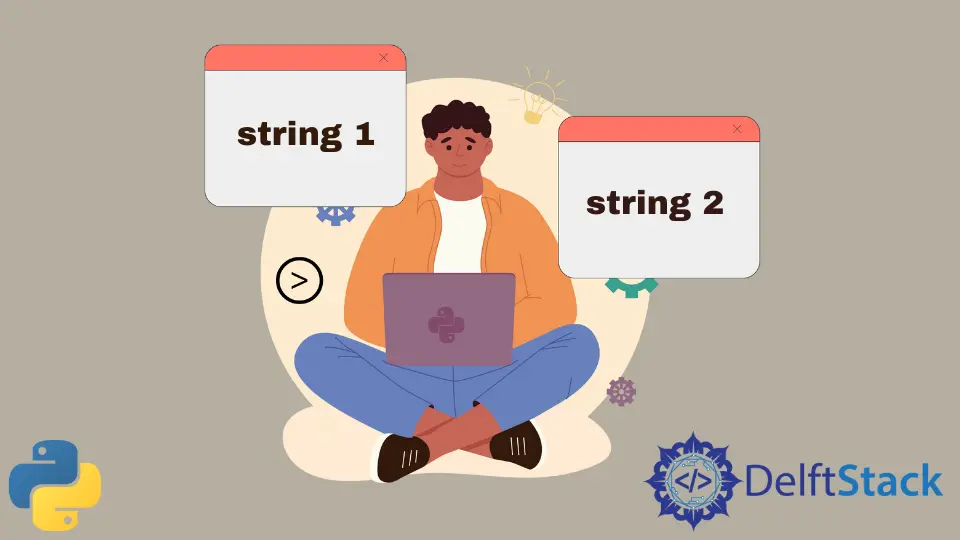
Strings are fundamental data types in Python, and comparing them character by character is a common operation in programming. In this article, we will explore several methods to compare two strings character by character in Python, each with its advantages and use cases.
Compare Strings Character by Character in Python Using a for
Loop
In Python, a for
loop is a convenient way to iterate over a sequence, making it well-suited for comparing two strings character by character. The idea is to use the zip()
function to pair up characters at the same positions in both strings.
We then iterate through these pairs, checking if the characters are equal. If a mismatch is encountered, we know the strings are different.
The counter is incremented for every match, providing the total count of identical characters.
The syntax of the zip()
function is as follows:
zip(iterable1, iterable2, ...)
Where:
iterable1
,iterable2
, …: These are the iterables (lists, tuples, strings, etc.) that you want to zip together.
This function will return an iterator of tuples where the i-th tuple has the i-th element from every input iterables. If the input iterables have different lengths, zip()
stops creating tuples when the shortest input iterable is exhausted.
Here is the complete Python code implementing the character-by-character comparison using a for
loop:
def compare_strings(str1, str2):
if str1 is None or str2 is None:
print("Number of Same Characters: 0")
return
size = min(len(str1), len(str2))
count = 0
for char1, char2 in zip(str1, str2):
if char1 == char2:
count += 1
print("Number of Same Characters:", count)
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
The compare_strings
function begins by checking if either of the input strings is None
. If so, it prints a message indicating that the number of identical characters is zero.
Next, it determines the minimum length of the two strings to ensure that we only iterate up to the length of the shorter string.
The for
loop utilizes the zip()
function to pair up characters from str1
and str2
. Within the loop, it compares each pair of characters, and if they are equal, it increments the count
variable.
Finally, the function prints the total count of identical characters.
Output:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
Compare Two Strings Character by Character in Python Using a while
Loop
Using a while
loop for character comparison offers an alternative to the for
loop. The idea is to initialize an index variable, typically named i
, to track our position in the strings.
We continue iterating while i
is less than the minimum length of the two strings. Within the loop, we compare characters at the current index, and if they are equal, we increment a counter to keep track of identical characters.
The loop continues until we reach the end of the shorter string.
Here is the complete Python code implementing the character-by-character comparison using a while
loop:
def compare_strings(str1, str2):
if str1 is None or str2 is None:
print("Number of Same Characters: 0")
return
size = min(len(str1), len(str2))
count = 0
i = 0
while i < size:
if str1[i] == str2[i]:
count += 1
i += 1
print("Number of Same Characters:", count)
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
The compare_strings
function begins by checking if either of the input strings is None
, and if so, it prints a message indicating that the number of identical characters is zero. Next, it determines the minimum length of the two strings to ensure that we only iterate up to the length of the shorter string.
A while
loop is used for iteration, with the loop condition ensuring that we stay within the bounds of the shorter string. Within the loop, the function compares characters at the current index (i
) in both strings.
If they are equal, the count
variable is incremented. The loop continues until we reach the end of the shorter string.
Output:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
Compare Two Strings Character by Character in Python Using the ==
Operator
When it comes to comparing two strings character by character in Python, the ==
operator provides another straightforward solution.
The ==
operator in Python is used for equality comparison. When applied to strings, it checks if the content of the two strings is identical.
In the context of comparing two strings character by character, we can directly use the ==
operator in a boolean expression. This approach leverages Python’s built-in string comparison functionality, making the code clean and easy to understand.
Here is the complete Python code implementing the character-by-character comparison using the ==
operator:
def compare_strings(str1, str2):
if str1 is None or str2 is None:
print("Number of Same Characters: 0")
return
size = min(len(str1), len(str2))
count = sum(char1 == char2 for char1, char2 in zip(str1, str2))
print("Number of Same Characters:", count)
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
The compare_strings
function starts by checking if either of the input strings is None
. If so, it prints a message indicating that the number of identical characters is zero.
Then, it finds the minimum length of the two strings to ensure that we only compare up to the length of the shorter string.
The heart of the comparison lies in the line that uses the ==
operator within a generator expression passed to the sum()
function. This generates a sequence of True
and False
values, indicating whether characters at corresponding positions are equal.
The sum()
function then counts the number of True
values, giving us the total count of identical characters.
Output:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
Compare Two Strings Character by Character in Python Using all()
With zip()
A more concise approach for comparing two strings character by character in Python involves the combination of all()
with zip()
. The zip()
function in Python is employed to combine elements from multiple iterables, creating pairs of corresponding elements.
When combined with the all()
function, which returns True
if all elements in an iterable are true, we can efficiently compare characters at the same positions in two strings. The result is a boolean indicating whether all character pairs are equal.
Here is the complete Python code implementing the character-by-character comparison using all()
with zip()
:
def compare_strings(str1, str2):
if str1 is None or str2 is None:
print("Number of Same Characters: 0")
return
size = min(len(str1), len(str2))
are_equal = all(char1 == char2 for char1, char2 in zip(str1, str2))
if are_equal:
print(f"All characters are the same in the first {size} positions.")
else:
print(f"Not all characters are the same in the first {size} positions.")
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
The compare_strings
function begins by checking if either of the input strings is None
. If so, it prints a message indicating that the number of identical characters is zero.
Next, it determines the minimum length of the two strings to ensure that we only compare up to the length of the shorter string.
The crucial line uses a generator expression within the all()
function, which iterates through pairs of characters from str1
and str2
created by the zip()
function. The all()
function checks if all the character pairs are equal, resulting in a boolean value (are_equal
).
The function then prints a message based on the boolean result, indicating whether all characters are the same in the specified positions.
Output:
All characters are the same in the first 9 positions.
Not all characters are the same in the first 5 positions.
All characters are the same in the first 5 positions.
Not all characters are the same in the first 4 positions.
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
Compare Two Strings Character by Character in Python Using enumerate()
When the need arises to compare two strings character by character in Python, the enumerate()
function comes in handy. The enumerate()
function in Python is designed to add a counter to an iterable, providing both the element and its index during iteration.
Its syntax is as follows:
enumerate(iterable, start=0)
Where:
iterable
: This is the iterable (e.g., a list, tuple, string, or any other iterable) that you want to enumerate.start
: This is an optional parameter that specifies the starting value of the counter. The default is0
.
When applied to comparing strings character by character, enumerate()
allows us to access both the character and its position in the string. This enables a more intricate comparison process where the index of characters can be utilized for additional operations.
Here is the complete Python code implementing the character-by-character comparison using enumerate()
:
def compare_strings(str1, str2):
if str1 is None or str2 is None:
print("Number of Same Characters: 0")
return
size = min(len(str1), len(str2))
count = sum(char1 == char2 for _, (char1, char2) in enumerate(zip(str1, str2)))
print("Number of Same Characters:", count)
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
The compare_strings
function starts by checking if either of the input strings is None
. If so, it prints a message indicating that the number of identical characters is zero.
Next, it determines the minimum length of the two strings to ensure that we only compare up to the length of the shorter string.
The key to this approach lies in the line that utilizes enumerate()
within the generator expression passed to the sum()
function. The enumerate()
function provides both the index and character during iteration, allowing us to access and compare characters directly.
The sum()
function then counts the number of identical characters.
Output:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
Compare Two Strings Character by Character in Python Using itertools.zip_longest()
Another approach we can use for comparing two strings character by character in Python is the itertools.zip_longest()
function. The itertools.zip_longest()
function in Python is designed to combine multiple iterables of unequal lengths.
Its syntax is as follows:
itertools.zip_longest(iterable1, iterable2, ..., fillvalue=None)
Where:
iterable1
,iterable2
, …: These are the input iterables (lists, tuples, strings, etc.) that you want to combine.fillvalue
: This is an optional parameter that specifies the value used to fill in missing values in the tuples. The default isNone
.
Unlike zip()
, which truncates at the length of the shortest iterable, zip_longest()
fills in missing values with a specified fill value.
In the context of comparing two strings character by character, this function is valuable when dealing with strings of different lengths. It ensures that the comparison considers all characters, even if one string is longer than the other.
Here is the complete Python code implementing the character-by-character comparison using itertools.zip_longest()
:
from itertools import zip_longest
def compare_strings(str1, str2):
if str1 is None or str2 is None:
print("Number of Same Characters: 0")
return
are_equal = (
char1 == char2 for char1, char2 in zip_longest(str1, str2, fillvalue="")
)
print("Number of Same Characters:", sum(are_equal))
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
The compare_strings
function begins by checking if either of the input strings is None
. If so, it prints a message indicating that the number of identical characters is zero.
The function then utilizes zip_longest()
within a generator expression to create pairs of characters from str1
and str2
. The fillvalue=''
ensures that missing values are filled with an empty string.
The result of the function is a generator of boolean values (True
if characters are equal, False
otherwise). The function then prints a message based on the boolean result, indicating whether all characters are the same.
Output:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
Conclusion
Choosing the right method to compare strings in Python depends on the specific requirements of your project. Whether you prioritize simplicity, performance, or customization, the methods outlined here offer a range of options for character-by-character string comparison.
Consider the characteristics of your data and the desired level of control to select the method that best fits your needs.