在 Python 中逐字符比较两个字符串
Vaibhav Vaibhav
2022年5月17日
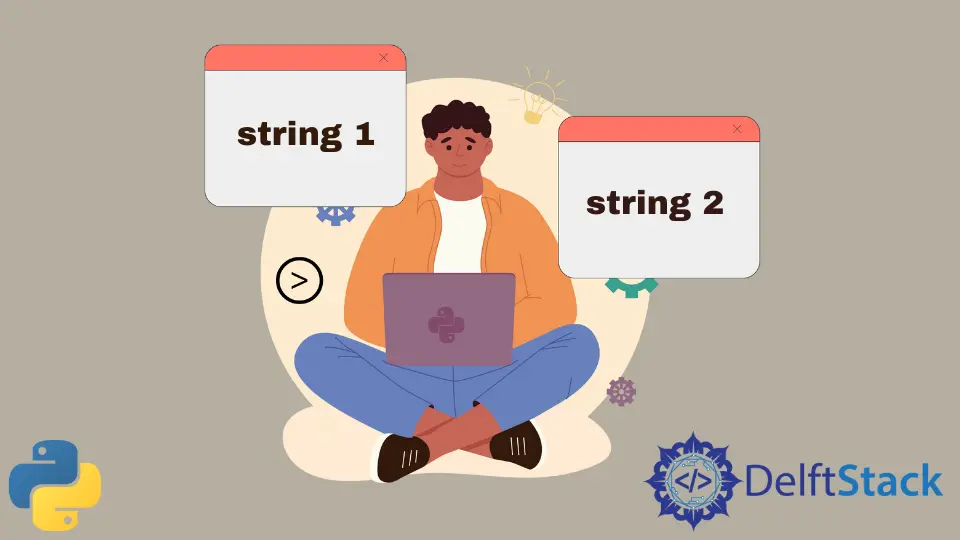
Python 是一种通用语言,它使得处理整数类型、字符串类型、浮点类型、双精度类型等数据成为一项相对无缝的任务,其易于理解的语法和强大的 API 在幕后工作。
本文将讨论一些涉及字符串的此类任务。任务是使用 Python 逐个字符地比较两个字符串。
在 Python 中逐字符比较字符串
在 Python 中,我们可以使用 for
循环或 while
循环逐个字符地比较两个字符串。
由于两个字符串可以有不同的长度,我们必须确保我们只考虑较小的长度来迭代字符串进行比较。为了进行比较,我们将计算位于相同索引处的两个字符串中相同字符的数量。
请注意,这只是比较两个字符串的一种方法。
可以计算字符串中每个字符出现的频率以进行比较或计算汉明距离。汉明距离是字符串中字符不同的索引个数。
下面的 Python 代码实现了我们上面讨论的内容。
def compare_strings(a, b):
if a is None or b is None:
print("Number of Same Characters: 0")
return
size = min(len(a), len(b)) # Finding the minimum length
count = 0 # A counter to keep track of same characters
for i in range(size):
if a[i] == b[i]:
count += 1 # Updating the counter when characters are same at an index
print("Number of Same Characters:", count)
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
输出:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
上面代码的时间复杂度是 O(n)
,空间复杂度是 O(1)
,因为我们只存储了计数和最小长度。
上面的代码使用了一个 for
循环。如上所述,我们还可以使用 while
循环来实现相同的功能。相同的参考下面的代码。
def compare_strings(a, b):
if a is None or b is None:
print("Number of Same Characters: 0")
return
size = min(len(a), len(b)) # Finding the minimum length
count = 0 # A counter to keep track of same characters
i = 0
while i < size:
if a[i] == b[i]:
count += 1 # Updating the counter when characters are same at an index
i += 1
print("Number of Same Characters:", count)
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
输出:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
上面代码的时间复杂度是 O(n)
,空间复杂度是 O(1)
,因为我们只存储了计数和最小长度。
作者: Vaibhav Vaibhav