Python で 2つの文字列を文字ごとに比較する
Vaibhav Vaibhav
2022年1月22日
Python
Python String
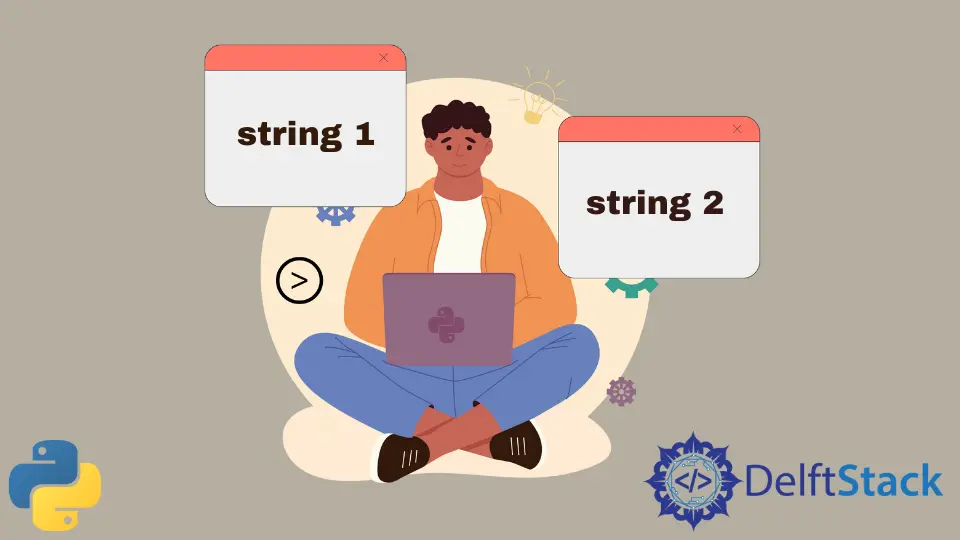
Python は、整数型、文字列型、浮動小数点型、倍精度型などのデータを操作できる汎用言語であり、理解しやすい構文と堅牢な API が舞台裏で機能する比較的シームレスなタスクです。
この記事では、文字列を含むそのようなタスクについて説明します。そして、タスクは、Python を使用して、2つの文字列を文字ごとに比較することです。
Python で文字列を文字ごとに比較する
Python では、for
ループまたは while
ループのいずれかを使用して、2つの文字列を文字ごとに比較できます。
2つの文字列の長さは異なる可能性があるため、比較のために文字列を反復処理する場合は、短い方の長さのみを考慮する必要があります。比較のために、同じインデックスにある両方の文字列の同じ文字の数を数えます。
これは、2つの文字列を比較する 1つの方法にすぎないことに注意してください。
比較のために文字列に存在する各文字の頻度を数えたり、ハミング距離を計算したりできます。ハミング距離は、文字列の文字が異なるインデックスの数です。
次の Python コードは、上記で説明した内容を実装しています。
def compare_strings(a, b):
if a is None or b is None:
print("Number of Same Characters: 0")
return
size = min(len(a), len(b)) # Finding the minimum length
count = 0 # A counter to keep track of same characters
for i in range(size):
if a[i] == b[i]:
count += 1 # Updating the counter when characters are same at an index
print("Number of Same Characters:", count)
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
出力:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
上記のコードの時間計算量は O(n)
であり、カウントと最小長のみを格納しているため、スペース計算量は O(1)
です。
上記のコードは for
ループを使用しています。上記のように、while
ループを使用して同じ機能を実装することもできます。同じことについては、次のコードを参照してください。
def compare_strings(a, b):
if a is None or b is None:
print("Number of Same Characters: 0")
return
size = min(len(a), len(b)) # Finding the minimum length
count = 0 # A counter to keep track of same characters
i = 0
while i < size:
if a[i] == b[i]:
count += 1 # Updating the counter when characters are same at an index
i += 1
print("Number of Same Characters:", count)
compare_strings("homophones", "homonyms")
compare_strings("apple", "orange")
compare_strings("apple", "applepie")
compare_strings("pasta", "pizza")
compare_strings(None, None)
compare_strings(None, "valorant")
compare_strings("minecraft", None)
出力:
Number of Same Characters: 4
Number of Same Characters: 0
Number of Same Characters: 5
Number of Same Characters: 2
Number of Same Characters: 0
Number of Same Characters: 0
Number of Same Characters: 0
上記のコードの時間計算量は O(n)
であり、スペースの複雑さは O(1)
です。これは、カウントと最小長のみを格納しているためです。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Vaibhav Vaibhav