How to Clear a List in Python
-
Use
clear()
to Clear a List in Python - Set an Empty List Value to Clear a List in Python
-
Use
del
and the Slice Operator:
to Clear a List in Python -
Multiply Entire List With
0
to Clear a List in Python
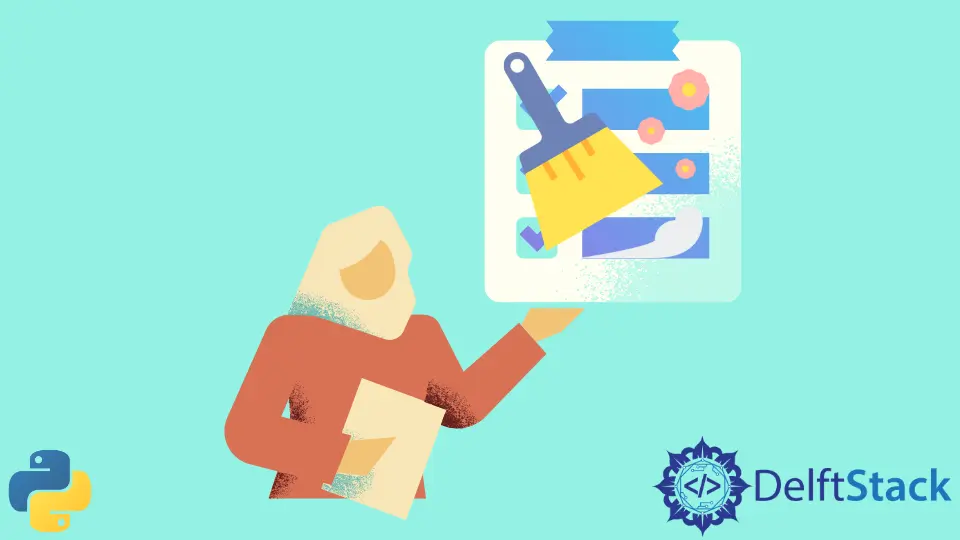
This tutorial will demonstrate different ways to clear a list in Python.
Use clear()
to Clear a List in Python
The easiest and most obvious way to clear a list in Python is to use the clear()
method, a predefined method within Python lists.
For example, initialize a list with integer values and use the clear()
function to erase all the values.
lst = [1, 6, 11, 16]
print(lst)
lst.clear()
print(lst)
Output:
[1, 6, 11, 16]
[]
Set an Empty List Value to Clear a List in Python
Another way to clear an existing list variable is to set an empty list to the variable using an empty pair of square brackets []
.
lst = [1, 6, 11, 16]
print(lst)
lst = []
print(lst)
Output:
[1, 6, 11, 16]
[]
Although this also does a quick job of clearing a list, this will not remove the values of the list from memory; instead, it creates a new instance of a list and points to the newly created instance.
If no other variable is pointing to the list variable’s previous values, then those variables would be unreachable and would be up for garbage collection.
This situation can be made clear if we made two variables point to the same list and set one of those variables to an empty list afterward.
lst = [1, 6, 11, 16]
lst2 = lst
print(lst)
print(lst2)
lst = []
print(lst)
print(lst2)
Output:
[1, 6, 11, 16]
[1, 6, 11, 16]
[]
[1, 6, 11, 16]
The second list, lst2
, was set to the value of lst
, but even after setting the new lst
value to an empty list, the list lst2
still retains the old values from before. In this case, the previous values are not eligible for garbage collection since there still is a variable pointing to it.
Use del
and the Slice Operator :
to Clear a List in Python
The Python keyword del
is used to delete objects and their references in memory completely. The del
keyword is used to delete Python objects, iterables, portions of lists, etc.
The colon operator :
in Python is an operator used to slice arrays into parts that accepts two integer arguments.
The :
operator is used to obtaining the actual reference of the list for deletion.
lst = [1, 6, 11, 16]
lst2 = lst
print(lst)
print(lst2)
del lst[:]
print(lst)
print(lst2)
Another solution that will do the same thing as this without using the del
keyword would be to set the list as an empty list but use the :
operator to replace the previous reference with the empty list.
lst = [1, 6, 11, 16]
lst2 = lst
print(lst)
print(lst2)
lst[:] = []
print(lst)
print(lst2)
The output of both solutions is as follows:
[1, 6, 11, 16]
[1, 6, 11, 16]
[]
[]
From the previous example, it is noted that setting an existing list variable to an empty list doesn’t actually delete the previous values. This solution will completely delete the previous values even if other variables point to the same reference of the values within the main list.
Multiply Entire List With 0
to Clear a List in Python
The quickest method of clearing a list would be to multiply the entire list with 0
. Multiplying the list variable itself with 0
will clear the list and clear its previous reference in memory.
lst = [1, 6, 11, 16]
lst2 = lst
print(lst)
print(lst2)
lst *= 0
print(lst)
print(lst2)
Output:
[1, 6, 11, 16]
[1, 6, 11, 16]
[]
[]
This method is the quickest performing solution out of all the mentioned solutions.
In summary, the most obvious way to clear a list is to use the built-in clear()
function in lists, though the quickest performing solution would be to multiply the list variable with the value 0
.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python