How to Use Circular List in Python
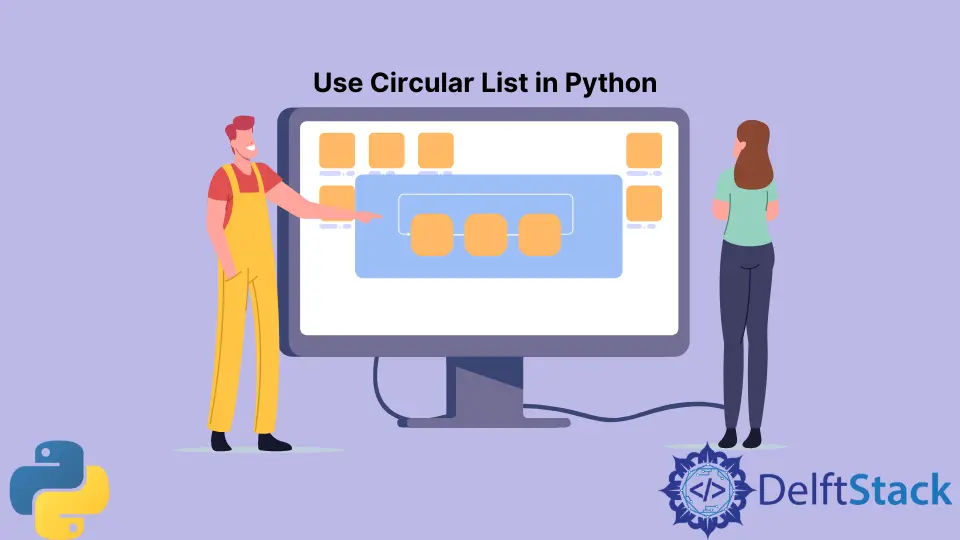
A regular linked list has a root node where each node has a pointer to the next node and a piece of data except for the last node, which does not have a next node (so holds a Null
value) that tells us that’s the last node. However, in a circular linked list, the last node has a pointer back to the first node in the list.
So, circular linked lists are linked lists structured such that all the nodes within the said structure form a circle. This linear and dynamic data structure whose nodes can’t be None
; has the last node connected to the first node so every node can lead to the previous or the next, and on and on.
Typically, an iterator
object allows us to move from one element to another within a list or dictionary data structure. With a circular list, the connection between each node allows for that especially using built-in functions.
This article discusses implementing and using a circular list in Python, natively or with modules.
Use itertools.cycle
to Use Circular List in Python
Python has a built-in module called itertools
that enables sequences with iterators and functional looping. Within this module, iterators (methods) help work out efficient systems to loop across different contexts, from short input sequences to combinatoric scenarios.
More important to use, however, is the cycle()
iterator which is an infinite iterator that takes a list and iterates its infinitely.
This occurs by creating an iterator that returns its elements and copies each element from the iterable. Afterward, it returns the items from the saved copy after the iterable has finished.
To use the module itertools
and the method cycle()
, we make use of this import
statement:
from itertools import cycle
All we have to do from here is pass a list to the cycle()
method, and we have a circular linked list. In addition, we can use a built-in function, next()
, to access and return the next element or node from the iterator, which is the circular linked list.
from itertools import cycle
numList = ["one", "two", "three", "four", "five"]
circularList = cycle(numList)
print(next(circularList))
print(next(circularList))
Output:
one
two
So, with the next()
method, we can easily advance the iterator one by one instead of using the for
loop, which loops forever.
from itertools import cycle
numList = ["one", "two", "three", "four", "five"]
circularList = cycle(numList)
for num in circularList:
print(num)
Output:
one
two
three
four
five
one
two
three
four
Use generators
to Use Circular List in Python
With generators, we can create iterations and functions that return an iterator which we can iterate over. Unlike typical functions where we use return
statements, we use the yield
statements in generators
.
Unlike return
statements, the yield
statement pauses the function, saves all its environment variables (or state), and carries one after that with subsequent calls. In addition, a generator
function can contain a return
statement and more than one yield
statement.
To create a circular list, we can use the yield
statement to save the function’s state and wait on successive calls.
def circular(args):
while True:
for element in args:
yield element
numList = ["one", "two", "three", "four", "five"]
circularList = circular(numList)
print(circularList)
Output:
<generator object circular at 0x0000018AFFD39A10>
With the generator
function called circular()
, we can pass an iterable argument to it. It loops forever using the while True
statement but can pause the function to return the current state (which means the current element or node it’s on) and continue when another call has been made.
This helps us create a circular list.
You can use the next()
method to retrieve the next element within the circular list.
def circular(args):
while True:
for element in args:
yield element
numList = ["one", "two", "three", "four", "five"]
circularList = circular(numList)
print(next(circularList))
print(next(circularList))
Output:
one
two
Or loop infinitely too.
def circular(args):
while True:
for element in args:
yield element
numList = ["one", "two", "three", "four", "five"]
circularList = circular(numList)
for i in circularList:
print(i)
Output:
one
two
three
four
five
one
two
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python