How to Catch All Exceptions in Python
- Understanding Exceptions in Python
- Using a Generic Exception Handler
- Catching Specific Exceptions
- Using Finally for Cleanup
- Raising Exceptions
- Conclusion
- FAQ
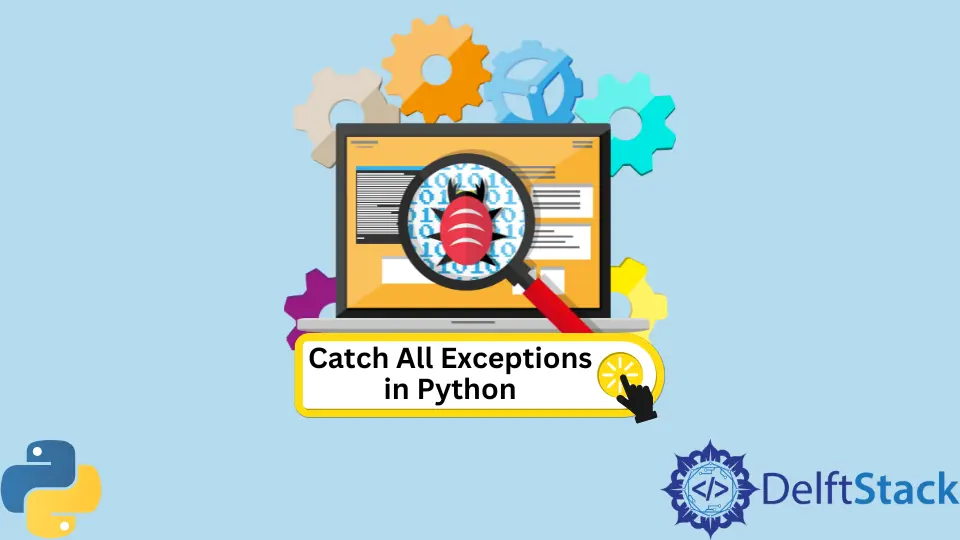
Catching exceptions in Python is an essential skill for developers. It allows you to handle errors gracefully, ensuring that your program continues to run smoothly even when things go wrong.
In this tutorial, we will explore how to catch all exceptions in Python, providing you with the tools to manage errors effectively. We will delve into various methods, showcasing practical examples to illustrate each technique. Whether you’re a beginner looking to enhance your coding skills or an experienced developer in need of a refresher, this guide will equip you with the knowledge to handle exceptions like a pro. Let’s dive in!
Understanding Exceptions in Python
Before we get into the nitty-gritty of catching exceptions, it’s important to understand what exceptions are. In Python, exceptions are events that disrupt the normal flow of a program. They can arise from various situations, such as trying to divide by zero, accessing an out-of-range index in a list, or attempting to open a file that doesn’t exist. When an exception occurs, Python raises an error, which can lead to program termination if not handled properly.
To catch exceptions, Python provides a robust mechanism using the try
and except
blocks. By wrapping your code in a try
block, you can specify what to do when an error occurs in the associated except
block. Let’s explore how to catch all exceptions effectively.
Using a Generic Exception Handler
One of the simplest ways to catch exceptions in Python is by using a generic exception handler. This method allows you to capture any exception that might occur, giving you the ability to respond appropriately. Here’s how it works:
try:
# Code that may raise an exception
result = 10 / 0
except Exception as e:
print("An error occurred:", e)
Output:
An error occurred: division by zero
In this example, we attempt to divide 10 by zero, which raises a ZeroDivisionError
. The except Exception as e
clause catches this error, and we can access the error message through the variable e
. This method is beneficial because it doesn’t require you to specify each type of exception individually. However, keep in mind that catching all exceptions can sometimes mask underlying issues in your code, so use this approach judiciously.
Catching Specific Exceptions
While catching all exceptions is useful, there are times when you might want to handle specific exceptions differently. Python allows you to catch multiple exceptions in a single except
block or to create separate blocks for different exceptions. Here’s an example of both approaches:
try:
# Code that may raise multiple exceptions
value = int("not a number")
except (ValueError, TypeError) as e:
print("Value error or type error occurred:", e)
Output:
Value error or type error occurred: invalid literal for int() with base 10: 'not a number'
In this case, we attempt to convert a string that cannot be converted into an integer, which raises a ValueError
. By specifying (ValueError, TypeError)
in the except
clause, we can catch both types of exceptions in one go. This approach allows for more precise error handling, making your code cleaner and easier to debug.
Using Finally for Cleanup
Sometimes, you may need to ensure that certain cleanup actions occur regardless of whether an exception was raised. This is where the finally
block comes in handy. The code inside the finally
block will execute whether an exception occurs or not. Here’s how to use it:
try:
file = open("example.txt", "r")
content = file.read()
except FileNotFoundError as e:
print("File not found:", e)
finally:
if 'file' in locals():
file.close()
Output:
File not found: [Errno 2] No such file or directory: 'example.txt'
In this example, we attempt to open a file that doesn’t exist, raising a FileNotFoundError
. Regardless of whether the file is found, the finally
block ensures that the file is closed if it was opened. This is particularly useful for managing resources like files or network connections, ensuring that they are properly released.
Raising Exceptions
In some cases, you may want to raise exceptions intentionally. This can be useful for enforcing certain conditions in your code or for propagating errors up the call stack. You can raise exceptions using the raise
keyword. Here’s an example:
def check_positive(number):
if number < 0:
raise ValueError("The number must be positive")
return number
try:
check_positive(-5)
except ValueError as e:
print("Caught an exception:", e)
Output:
Caught an exception: The number must be positive
In this code, we define a function check_positive
that raises a ValueError
if the provided number is negative. When we call this function with -5, the exception is raised and caught in the except
block. This method allows you to create custom error messages and handle specific error conditions more effectively.
Conclusion
Catching exceptions in Python is a crucial skill that can significantly enhance the robustness of your programs. By understanding how to use generic handlers, catch specific exceptions, implement cleanup with finally
, and even raise exceptions intentionally, you can create code that is not only functional but also resilient to errors. Remember to strike a balance between catching all exceptions and addressing specific ones to ensure that your code remains clean and maintainable. With these techniques in your toolkit, you’ll be well-equipped to handle whatever your Python journey throws at you.
FAQ
-
What is an exception in Python?
An exception is an event that disrupts the normal flow of a program, often due to errors such as invalid input or resource unavailability. -
How do I catch specific exceptions in Python?
You can catch specific exceptions by specifying them in theexcept
clause, allowing you to handle different errors in distinct ways. -
What is the purpose of the finally block?
Thefinally
block is used to execute cleanup code, ensuring that certain actions occur regardless of whether an exception was raised. -
Can I raise my own exceptions in Python?
Yes, you can raise your own exceptions using theraise
keyword to enforce specific conditions or propagate errors. -
Why is it important to handle exceptions?
Handling exceptions is crucial for maintaining the stability of your program, allowing it to respond gracefully to unexpected situations.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn