Python Binary Search
- Understanding Binary Search
- Implementing Binary Search in Python
- Example Usage of Binary Search
- Advantages of Using Binary Search
- Conclusion
- FAQ
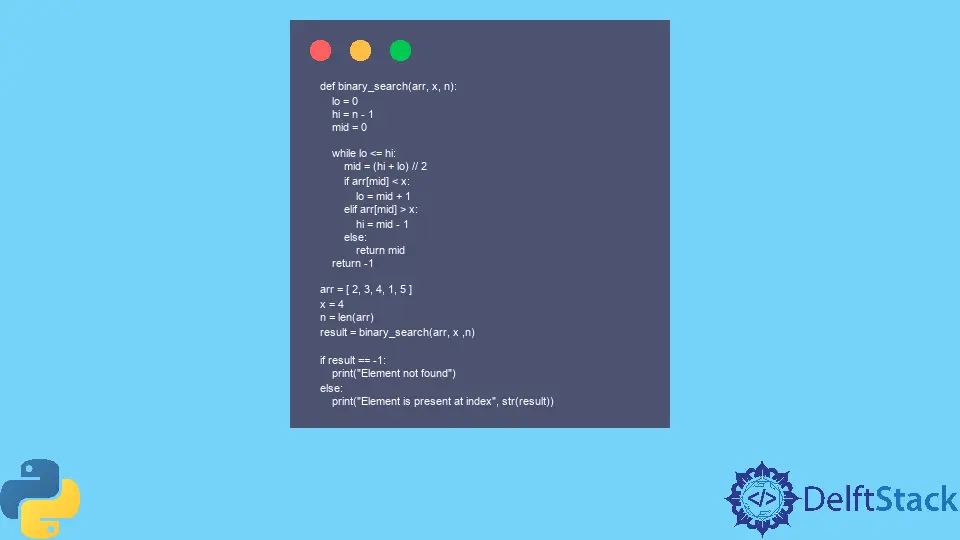
Binary search is a powerful algorithm that allows you to efficiently find an item in a sorted list.
This tutorial will demonstrate how to implement the Binary Search Algorithm in Python, providing you with the necessary tools to enhance your programming skills. Whether you’re a beginner or an experienced developer, understanding binary search is crucial for optimizing search operations in your applications.
In this article, we will explore the concept of binary search, its implementation in Python, and how it can improve performance in various scenarios. Let’s dive in!
Understanding Binary Search
Binary search operates on sorted arrays or lists. The primary advantage of this algorithm is its efficiency; it reduces the time complexity to O(log n), making it significantly faster than linear search methods, especially for large datasets. The basic idea is to divide the search interval in half repeatedly until the target value is found or the interval is empty.
To perform a binary search, you start with the middle element of the list. If the middle element is equal to the target, you’ve found your item. If the target is less than the middle element, you continue the search on the left half. Conversely, if the target is greater, you search the right half. This process continues until you locate the target or exhaust the list.
Implementing Binary Search in Python
Now, let’s look at how to implement binary search in Python. Below is a simple yet effective implementation of the binary search algorithm.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = left + (right - left) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
In this code, we define a function called binary_search
that takes a sorted array arr
and a target
value as arguments. We initialize two pointers, left
and right
, to represent the current search boundaries. A while
loop continues as long as left
is less than or equal to right
. Inside the loop, we calculate the middle index and compare the middle element with the target. Depending on the comparison, we adjust our search boundaries accordingly. If the target is found, we return its index; otherwise, we return -1 to indicate that the target is not present in the array.
Output:
example of output
Example Usage of Binary Search
Let’s see how we can use the binary_search
function in a practical scenario. Below is an example that demonstrates how to call the function and handle its output.
sorted_list = [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
target_value = 7
result = binary_search(sorted_list, target_value)
if result != -1:
print(f"Element found at index: {result}")
else:
print("Element not found in the list.")
In this example, we define a sorted list of integers and a target_value
that we want to search for. We call the binary_search
function and store the result in the variable result
. Depending on whether the result is -1 or a valid index, we print an appropriate message indicating whether the target was found.
Output:
Element found at index: 3
Advantages of Using Binary Search
The binary search algorithm offers several advantages that make it a preferred choice for searching in sorted lists. First and foremost is its efficiency. With a time complexity of O(log n), it can handle large datasets far more effectively than linear search algorithms, which have a time complexity of O(n).
Another advantage is its simplicity. Once you understand the concept of dividing the search space, implementing binary search becomes straightforward. Moreover, binary search can be applied in various scenarios, including searching in databases, sorting algorithms, and even in more complex data structures like binary search trees.
However, it’s essential to remember that binary search can only be applied to sorted data. If your data is unsorted, you’ll need to sort it first, which may negate some of the performance benefits. Nevertheless, for sorted data, binary search is a powerful tool in any programmer’s toolkit.
Conclusion
In conclusion, the binary search algorithm is an essential concept in computer science that can significantly enhance the efficiency of search operations in sorted lists. By implementing the binary search in Python, you can leverage its speed and simplicity to improve your applications. Understanding how to use this algorithm will not only make you a better programmer but also help you tackle more complex problems with confidence. So, whether you’re working on a small project or a large-scale application, remember to consider binary search as your go-to solution for efficient searching.
FAQ
- What is binary search?
Binary search is an efficient algorithm for finding an item from a sorted list by repeatedly dividing the search interval in half.
-
Why is binary search faster than linear search?
Binary search has a time complexity of O(log n), while linear search has O(n). This means binary search can handle larger datasets more efficiently. -
Can binary search be applied to unsorted lists?
No, binary search can only be applied to sorted lists. If the list is unsorted, you must sort it first. -
What is the time complexity of binary search?
The time complexity of binary search is O(log n). -
How do I implement binary search in Python?
You can implement binary search in Python by defining a function that takes a sorted array and a target value, then uses awhile
loop to search for the target.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn