Linear Search in Python
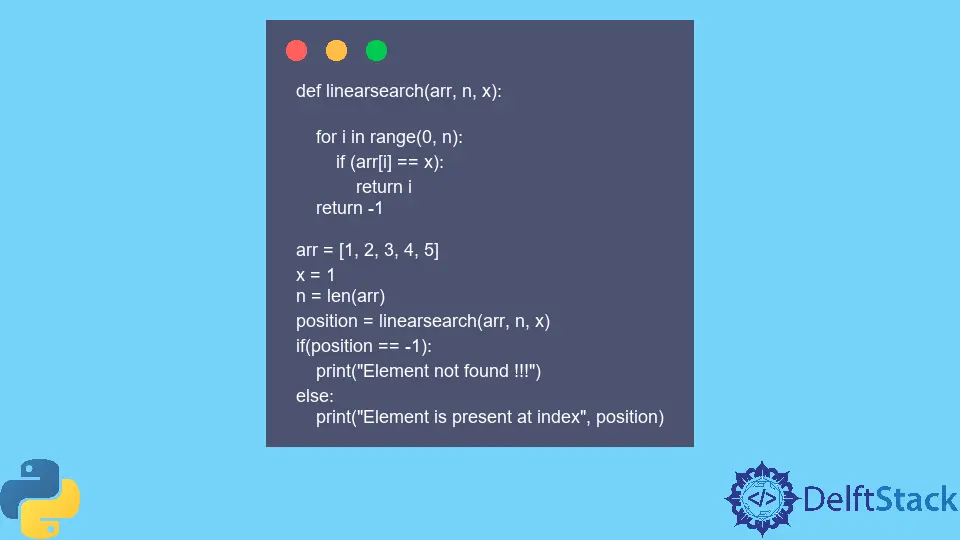
Searching for an element in a list or array is a common task in programming, and understanding how to implement different search algorithms is crucial for any aspiring developer. One of the simplest and most intuitive search methods is the Linear Search Algorithm.
In this tutorial, we will dive into the concept of linear search, explore its implementation in Python, and discuss its advantages and limitations. Whether you’re a beginner or looking to refresh your knowledge, this guide will provide you with valuable insights and practical code examples to help you master linear search in Python.
What is Linear Search?
Linear search, also known as sequential search, is a straightforward algorithm that checks each element in a list one by one until it finds the target value or reaches the end of the list. This method is easy to understand and implement, making it a popular choice for small datasets. However, as the size of the dataset increases, linear search may become inefficient compared to more advanced algorithms.
Implementing Linear Search in Python
Basic Linear Search
Let’s start with the most basic implementation of linear search in Python. The function will take a list and a target value as inputs, and it will return the index of the target if found or -1 if not found.
def linear_search(arr, target):
for index in range(len(arr)):
if arr[index] == target:
return index
return -1
# Example usage
numbers = [3, 5, 2, 9, 6]
result = linear_search(numbers, 9)
Output:
3
In this code, we define the linear_search
function, which iterates through each element of the array arr
. We use a simple for
loop to check if the current element matches the target. If a match is found, the function returns the index of that element. If the loop completes without finding the target, the function returns -1, indicating that the target is not present in the list. This implementation is straightforward but can be inefficient for large datasets due to its O(n) time complexity.
Linear Search with Early Exit
To optimize our linear search, we can implement an early exit strategy. This technique allows us to stop searching as soon as we find the target, potentially saving time.
def linear_search_early_exit(arr, target):
for element in arr:
if element == target:
return True
return False
# Example usage
numbers = [3, 5, 2, 9, 6]
result = linear_search_early_exit(numbers, 5)
Output:
True
In this version of the linear search, we use a for
loop to iterate through each element of the array. As soon as we find a match, we return True
, indicating that the target exists in the list. If the loop completes without finding the target, we return False
. This method is slightly more efficient than the basic implementation, as it avoids unnecessary comparisons after the target is found, maintaining an O(n) time complexity in the worst case while improving performance in many scenarios.
Linear Search with Count
Another interesting variation of linear search is to count the occurrences of a target value in the list. This can be useful when you want to know how many times a particular element appears.
def linear_search_count(arr, target):
count = 0
for element in arr:
if element == target:
count += 1
return count
# Example usage
numbers = [3, 5, 2, 9, 5, 6]
result = linear_search_count(numbers, 5)
Output:
2
In this implementation, we maintain a count
variable that increments each time we find a match for the target. At the end of the loop, we return the count. If the target is not found, the function will return 0. This method is particularly useful for datasets where duplicates are common and provides a clear example of how linear search can be adapted to meet specific needs.
Conclusion
Linear search is a fundamental algorithm that serves as a stepping stone to understanding more complex search techniques. While it may not be the most efficient method for large datasets, its simplicity and ease of implementation make it an excellent choice for beginners. By mastering linear search in Python, you’re building a solid foundation for exploring more advanced algorithms in the future. Whether you’re working on small projects or preparing for technical interviews, understanding linear search will undoubtedly be beneficial.
FAQ
-
What is linear search?
Linear search is an algorithm that checks each element in a list sequentially to find a target value. -
What are the advantages of linear search?
The main advantages are its simplicity and ease of implementation. It works on unsorted lists and requires no additional data structures. -
What is the time complexity of linear search?
The time complexity of linear search is O(n), where n is the number of elements in the list. -
When should I use linear search?
Linear search is suitable for small datasets or when you need to search through unsorted data. -
Can linear search be optimized?
Yes, implementing early exit strategies can optimize linear search by stopping the search as soon as the target is found.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn