Python 中的線性搜尋
Harshit Jindal
2023年1月30日
Python
Python Algorithm
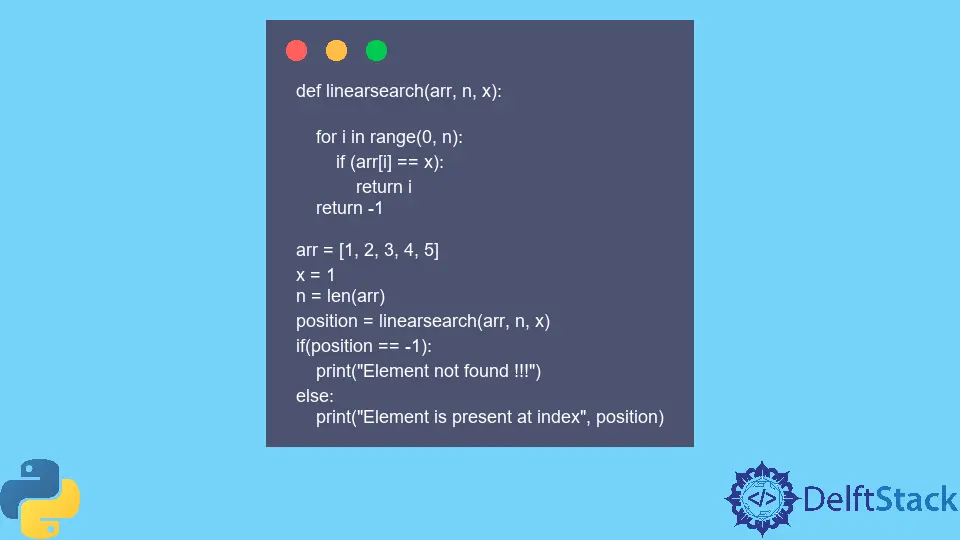
注意
如果你想詳細瞭解線性搜尋,那麼可以參考線性搜尋演算法一文。
線性搜尋演算法
假設我們有一個未排序的陣列 A[]
,包含 n
個元素,我們想找到一個元素-X
。
-
使用
for
迴圈從最左邊的元素開始遍歷陣列中的所有元素,並執行以下操作。- 如果
A[i]
的值與X
匹配,則返回索引i
。(如果有多個元素與X
匹配,則不返回索引i
,而是列印所有索引或將所有索引儲存在一個陣列中並返回該陣列。) - 否則繼續下一個元素。
- 如果你在陣列的最後一個元素,退出
for
迴圈。
- 如果
-
如果沒有一個元素匹配,那麼返回
-1
。
線性搜尋的 Python 實現
def linearsearch(arr, n, x):
for i in range(0, n):
if arr[i] == x:
return i
return -1
arr = [1, 2, 3, 4, 5]
x = 1
n = len(arr)
position = linearsearch(arr, n, x)
if position == -1:
print("Element not found !!!")
else:
print("Element is present at index", position)
輸出:
Element is found at index: 1
上述演算法的時間複雜度為 O(n)
。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Harshit Jindal
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn