Python 中的 Smith-Waterman 演算法
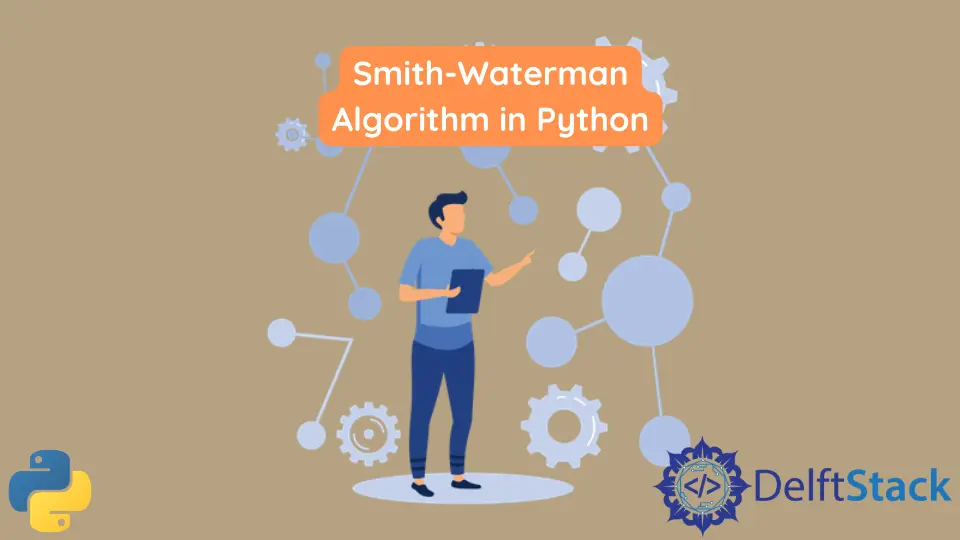
Smith-Waterman 演算法用於執行字串的區域性序列比對。這些字串主要代表 DNA 鏈或蛋白質序列。
本文討論了 Smith-Waterman 演算法在 Python 中的實現。
Python 中的 Smith-Waterman 演算法
swalign
模組包含幾個在 Python 中實現 Smith-Waterman 演算法的函式。你可以通過在命令列中執行以下語句來使用 PIP
安裝 swalign
模組。
pip3 install swalign
上述語句為 Python 版本 3 安裝模組。要在 Python 版本 2 中安裝模組,可以使用以下命令。
pip install swalign
安裝 swalign
模組後,我們將使用以下步驟在 Python 程式中實現 Smith-Waterman 演算法。
-
首先,我們將使用
import
語句匯入swalign
模組。 -
要進行比對,我們必須建立一個核苷酸評分矩陣。在矩陣中,我們為每個匹配和不匹配提供一個分數。
通常,我們使用 2 表示匹配分數,使用 -1 表示不匹配。
-
要建立核苷酸評分矩陣,我們將使用
NucleotideScoringMatrix()
方法。NucleotideScoringMatrix()
將匹配分數作為其第一個輸入引數,將不匹配分數作為其第二個輸入引數。執行後,它返回一個
IdentityScoringMatrix
物件。 -
一旦我們得到核苷酸矩陣,我們將使用
LocalAlignment()
方法建立一個LocalAlignment
物件。LocalAlignment()
方法將核苷酸評分矩陣作為其輸入並返回一個LocalAlignment
物件。 -
一旦我們得到
LocalAlignment
物件,我們可以使用align()
方法執行 Smith-Waterman 演算法。 -
align()
方法,當在LocalAlignment
物件上呼叫時,將代表 DNA 鏈的字串作為其第一個輸入引數。它需要另一個代表參考 DNA 鏈的字串。 -
執行後,
align()
方法返回一個Alignment
物件。Alignment
物件包含輸入字串的匹配詳細資訊和不匹配以及其他一些詳細資訊。
你可以在以下示例中觀察整個過程。
import swalign
dna_string = "ATCCACAGC"
reference_string = "ATGCAGCGC"
match_score = 2
mismatch_score = -1
matrix = swalign.NucleotideScoringMatrix(match_score, mismatch_score)
lalignment_object = swalign.LocalAlignment(matrix)
alignment_object = lalignment_object.align(dna_string, reference_string)
alignment_object.dump()
輸出:
Query: 1 ATGCAGC-GC 9
||.|| | ||
Ref : 1 ATCCA-CAGC 9
Score: 11
Matches: 7 (70.0%)
Mismatches: 3
CIGAR: 5M1I1M1D2M
まとめ
本文討論瞭如何使用 Python 的 swalign
模組實現 Smith-Waterman 演算法。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub