Algorithme de Smith-Waterman en Python
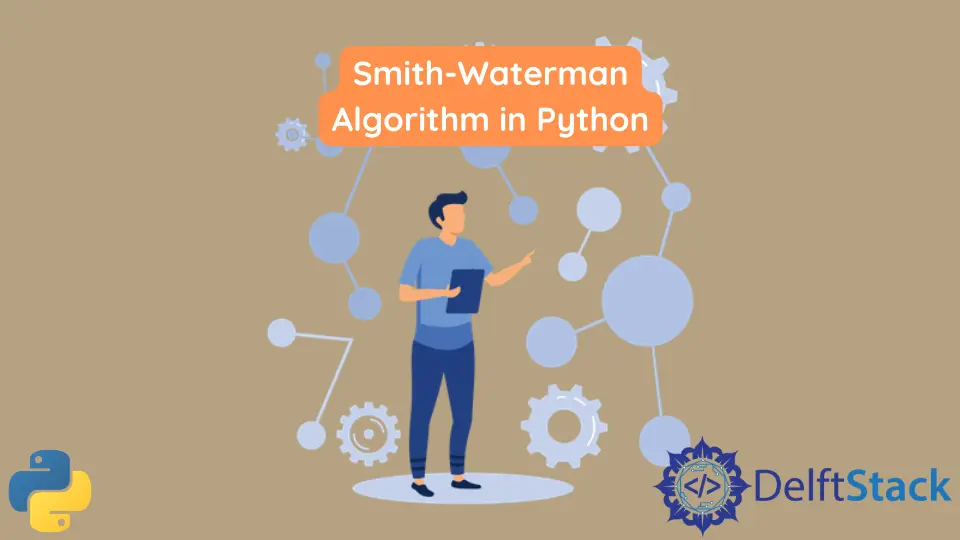
L’algorithme Smith-Waterman est utilisé pour effectuer un alignement de séquence local des chaînes. Les chaînes représentent principalement des brins d’ADN ou des séquences de protéines.
Cet article traite de l’implémentation de l’algorithme Smith-Waterman en Python.
Algorithme de Smith-Waterman en Python
Le module swalign
contient plusieurs fonctions pour implémenter l’algorithme de Smith-Waterman en Python. Vous pouvez installer le module swalign
à l’aide de PIP
en exécutant l’instruction suivante dans la ligne de commande.
pip3 install swalign
L’instruction ci-dessus installe le module pour Python version 3. Pour installer le module dans Python version 2, vous pouvez utiliser la commande suivante.
pip install swalign
Après avoir installé le module swalign
, nous utiliserons les étapes suivantes pour implémenter l’algorithme Smith-Waterman dans notre programme Python.
-
Tout d’abord, nous allons importer le module
swalign
à l’aide de l’instructionimport
. -
Pour effectuer l’alignement, nous devons créer une matrice de notation des nucléotides. Dans la matrice, nous fournissons un score pour chaque correspondance et non-concordance.
Généralement, nous utilisons 2 pour un score de correspondance et -1 pour un décalage.
-
Pour créer la matrice de notation des nucléotides, nous utiliserons la méthode
NucleotideScoringMatrix()
. LeNucleotideScoringMatrix()
prend le score de correspondance comme premier argument d’entrée et le score de non-concordance comme deuxième argument d’entrée.Après exécution, il retourne un objet
IdentityScoringMatrix
. -
Une fois la matrice de nucléotides obtenue, nous allons créer un objet
LocalAlignment
en utilisant la méthodeLocalAlignment
. La méthodeLocalAlignment
prend la matrice de notation des nucléotides en entrée et renvoie un objetLocalAlignment
. -
Une fois que nous avons obtenu l’objet
LocalAlignment
, nous pouvons exécuter l’algorithme de Smith-Waterman en utilisant la méthodealign()
. -
La méthode
align()
, lorsqu’elle est invoquée sur un objetLocalAlignment
, prend une chaîne représentant un brin d’ADN comme premier argument d’entrée. Il prend une autre chaîne représentant le brin d’ADN de référence. -
Après exécution, la méthode
align()
retourne un objetAlignment
. L’objetAlignment
contient les détails de correspondance et de non-concordance des chaînes d’entrée et plusieurs autres détails.
Vous pouvez observer l’ensemble du processus dans l’exemple suivant.
import swalign
dna_string = "ATCCACAGC"
reference_string = "ATGCAGCGC"
match_score = 2
mismatch_score = -1
matrix = swalign.NucleotideScoringMatrix(match_score, mismatch_score)
lalignment_object = swalign.LocalAlignment(matrix)
alignment_object = lalignment_object.align(dna_string, reference_string)
alignment_object.dump()
Production:
Query: 1 ATGCAGC-GC 9
||.|| | ||
Ref : 1 ATCCA-CAGC 9
Score: 11
Matches: 7 (70.0%)
Mismatches: 3
CIGAR: 5M1I1M1D2M
Conclusion
Cet article explique comment nous pouvons implémenter l’algorithme de Smith-Waterman en utilisant le module swalign
de Python.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub