Python 中的 Smith-Waterman 算法
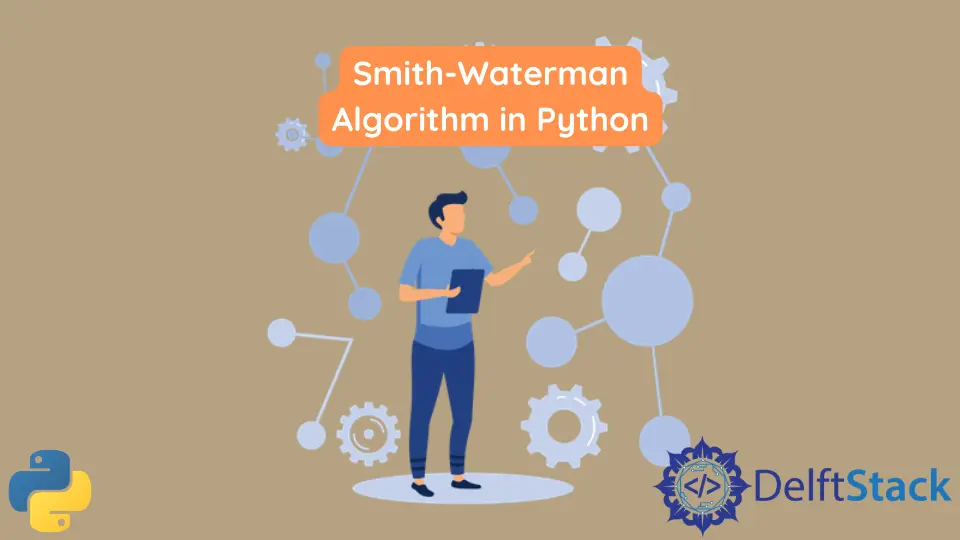
Smith-Waterman 算法用于执行字符串的局部序列比对。这些字符串主要代表 DNA 链或蛋白质序列。
本文讨论了 Smith-Waterman 算法在 Python 中的实现。
Python 中的 Smith-Waterman 算法
swalign
模块包含几个在 Python 中实现 Smith-Waterman 算法的函数。你可以通过在命令行中执行以下语句来使用 PIP
安装 swalign
模块。
pip3 install swalign
上述语句为 Python 版本 3 安装模块。要在 Python 版本 2 中安装模块,可以使用以下命令。
pip install swalign
安装 swalign
模块后,我们将使用以下步骤在 Python 程序中实现 Smith-Waterman 算法。
-
首先,我们将使用
import
语句导入swalign
模块。 -
要进行比对,我们必须创建一个核苷酸评分矩阵。在矩阵中,我们为每个匹配和不匹配提供一个分数。
通常,我们使用 2 表示匹配分数,使用 -1 表示不匹配。
-
要创建核苷酸评分矩阵,我们将使用
NucleotideScoringMatrix()
方法。NucleotideScoringMatrix()
将匹配分数作为其第一个输入参数,将不匹配分数作为其第二个输入参数。执行后,它返回一个
IdentityScoringMatrix
对象。 -
一旦我们得到核苷酸矩阵,我们将使用
LocalAlignment()
方法创建一个LocalAlignment
对象。LocalAlignment()
方法将核苷酸评分矩阵作为其输入并返回一个LocalAlignment
对象。 -
一旦我们得到
LocalAlignment
对象,我们可以使用align()
方法执行 Smith-Waterman 算法。 -
align()
方法,当在LocalAlignment
对象上调用时,将代表 DNA 链的字符串作为其第一个输入参数。它需要另一个代表参考 DNA 链的字符串。 -
执行后,
align()
方法返回一个Alignment
对象。Alignment
对象包含输入字符串的匹配详细信息和不匹配以及其他一些详细信息。
你可以在以下示例中观察整个过程。
import swalign
dna_string = "ATCCACAGC"
reference_string = "ATGCAGCGC"
match_score = 2
mismatch_score = -1
matrix = swalign.NucleotideScoringMatrix(match_score, mismatch_score)
lalignment_object = swalign.LocalAlignment(matrix)
alignment_object = lalignment_object.align(dna_string, reference_string)
alignment_object.dump()
输出:
Query: 1 ATGCAGC-GC 9
||.|| | ||
Ref : 1 ATCCA-CAGC 9
Score: 11
Matches: 7 (70.0%)
Mismatches: 3
CIGAR: 5M1I1M1D2M
结论
本文讨论了如何使用 Python 的 swalign
模块实现 Smith-Waterman 算法。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub