How to Append to a Tuple in Python
- Initialize a Tuple in Python
-
Use Concatenation
+
to Append to a Tuple in Python - Performe Tuple to List Conversion to Append to a Tuple in Python
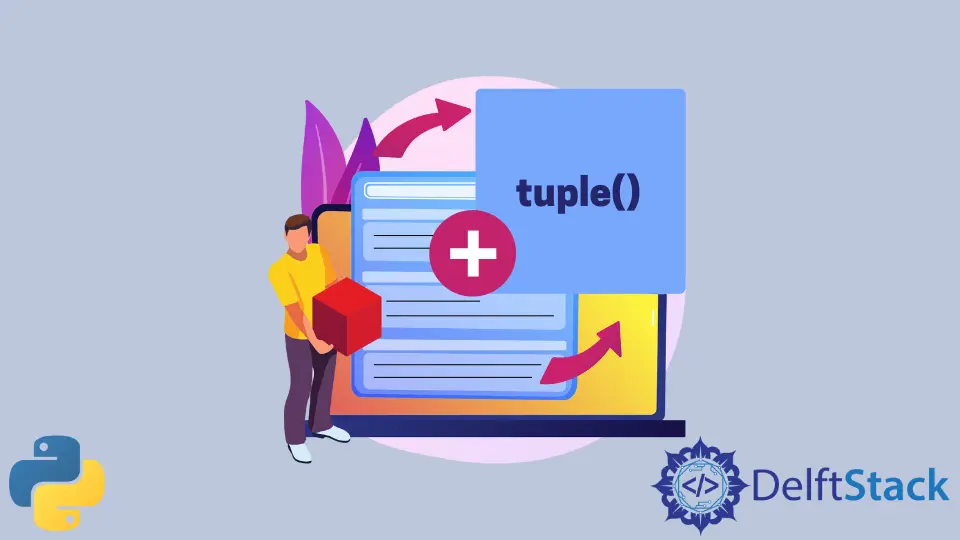
This tutorial will demonstrate how to append to a tuple in Python.
In Python, a tuple is an unordered, immutable data type that is used to store collections. Tuples are much like lists, but the difference is that a tuple has a fixed length and is immutable, unlike a list that is variable in length and mutable in nature.
Initialize a Tuple in Python
Tuple data types are initialized by encapsulating values using parentheses ()
. The values in the tuple do not have to be of the same type.
my_tuple = ("Jane Doe", 40, 0.05, "a", [1, 2, 3])
Here is an example of a tuple initialization containing four values of different data types. Nested tuples are also supported.
A list can also be converted into a tuple using the built-in function tuple()
, which converts any list into a tuple.
my_list = [1, 2, 3, 4, 5]
print(tuple(my_list))
Output:
(1, 2, 3, 4, 5)
Now that the initialization of a tuple is discussed, we can move on to how to append to an immutable tuple.
Use Concatenation +
to Append to a Tuple in Python
To reiterate, tuple data types are immutable, which means that any value that has been initialized can never be changed. Another example of an immutable data type is a string.
Much like strings, tuple values can be altered or appended by simply concatenating a new value onto the existing one. It combines two different sets of tuples into one and doesn’t actually change existing values, maintaining the immutability of the data type.
For example, here are two separate tuples. A third variable is initialized by concatenating these two tuples.
tuple_a = ("a", "b", "c")
tuple_b = ("d", "e", "f")
tuple_c = tuple_a + tuple_b
print(tuple_c)
Output:
('a', 'b', 'c', 'd', 'e', 'f')
Now the tuple_c
variable is initialized by the concatenated values of the two tuples.
Here’s another approach to concatenating tuples:
tuple_a = ("c", "d", "f")
tuple_b = ("a", "b") + tuple_a + ("g", "h")
print(tuple_b)
Output:
('a', 'b', 'c', 'd', 'f', 'g', 'h')
Alphabetically, the character e
is missing from the output above, and there isn’t any method to insert a value in the middle of a tuple using concatenation.
The only setback with this approach is that inserting a value in the middle of the tuple would not be possible since you can only concatenate either at the start or the end of an existing tuple.
Performe Tuple to List Conversion to Append to a Tuple in Python
A more flexible and convenient approach to append to a tuple in Python is by converting a tuple into a list.
In this way, built-in list functions can be performed on the values to perform convenient insertion and removal anywhere within the list. After all the manipulation, the list can then be converted back into an immutable tuple.
Here’s an example of a tuple-to-list conversion to insert new elements.
tuple_val = (11, 52, 67, 142)
list_val = list(tuple_val)
l.insert(1, 20)
print(l)
tuple_val = tuple(list_val)
print(tuple_val)
The tuple is first converted into a list, and the built-in insert()
function is used to insert a value in between the existing values. Afterward, the list is converted back to a tuple.
Output:
[11, 20, 52, 67, 142]
(11, 20, 52, 67, 142)
Converting the tuple into a list will open other possibilities using built-in functions like sort()
, remove()
, and other possible list manipulations.
In summary, tuples can’t be simply modified like lists because of their immutable nature. The most extensive way to append to a tuple is to convert the tuple into a list. If the only addition needed is either at the start or the end of the tuple, then simple concatenation +
can be used.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn