How to List the Alphabet in Python
-
Use Utils From Module
string
to List the Alphabet in Python -
Use
range()
to List the Alphabet in Python
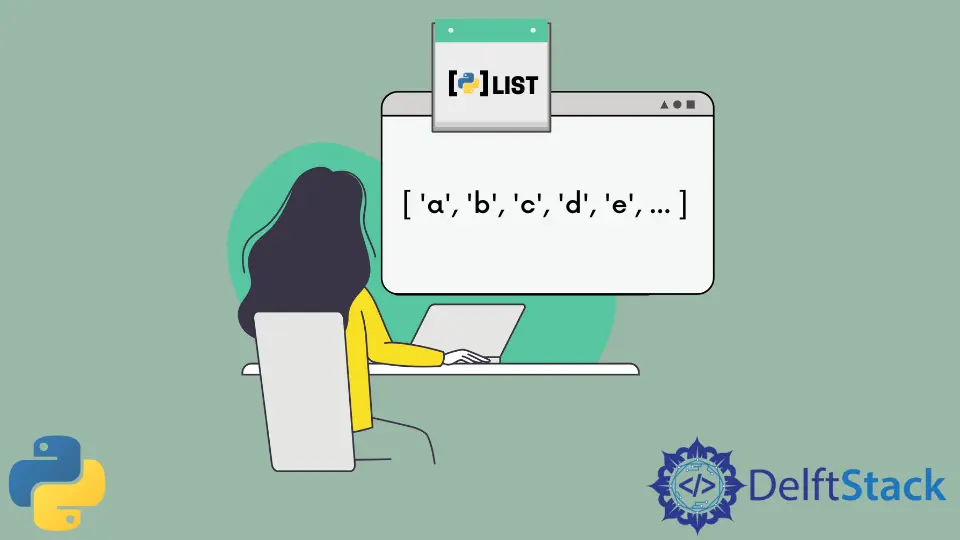
This tutorial shows you how to list the alphabet by the range in Python.
In this tutorial, we want to store the English alphabet’s 26 lowercase characters in a Python list. The quickest way to solve this problem is by making use of the ASCII values of each character and using pre-existing functions in Python.
Use Utils From Module string
to List the Alphabet in Python
The Python module string
is readily available and contains pre-defined constant values that we can use for this problem. The constant string.ascii_lowercase
contains all 26 lowercase characters in string format.
If you perform print(string.ascii_lowercase)
, it will result in the following output:
'abcdefghijklmnopqrstuvwxyz'
Therefore, we can use this constant and convert it into a list of characters to produce a list of the alphabet.
import string
def listAlphabet():
return list(string.ascii_lowercase)
print(listAlphabet())
Output:
[
"a",
"b",
"c",
"d",
"e",
"f",
"g",
"h",
"i",
"j",
"k",
"l",
"m",
"n",
"o",
"p",
"q",
"r",
"s",
"t",
"u",
"v",
"w",
"x",
"y",
"z",
]
If you prefer the alphabet list to be in uppercase, then you should use string.ascii_uppercase
and reuse the code above and will produce the same output, but in uppercase format.
Use range()
to List the Alphabet in Python
range()
is a function that outputs a series of numbers. You can specify when the function starts and stops with the first and second arguments.
range()
and map()
map()
is a function that accepts two arguments: the second argument of the function is an iterable or a collection; the first argument is a function to iterate over and handle the second argument.
We’re going to use these two methods to generate a list of the alphabet using the lowercase letters’ ASCII values and map them with the function chr()
, which converts integers into their ASCII counterpart.
def listAlphabet():
return list(map(chr, range(97, 123)))
print(listAlphabet())
range()
is used to list the integers from 97 until 122. 97 is the ASCII value of the lowercase a
and 122 is for z
, so if we use map()
and chr()
to perform the conversion from its ASCII counterpart to its corresponding characters, we would successfully output a list of the lowercase alphabet.
The second argument for range()
is 123 because the second argument is exclusive, which means it uses it as a stop condition.
range()
and ord()
ord()
is practically the reverse of chr()
because it converts characters into its ASCII counterpart.
We’ll use ord()
as the arguments of range()
to make a list of lowercase alphabets.
def listAlphabet():
return [chr(i) for i in range(ord("a"), ord("z") + 1)]
print(listAlphabet())
We loop every output of range()
and convert them into lowercase alphabet using chr()
.
Both will produce the same output:
['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z']
To summarize, the easiest way to list the alphabet in Python, whether lowercase or uppercase, is to use pre-defined methods that can handle ASCII values and convert them into their actual counterparts. You can use the constants from the string
module and convert them into a list, or you can use range()
and use the ASCII values as arguments to generate a list of the alphabet.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python