How to Print String to Text File Using Python
-
Write to Text File With the
open()
Method in Python -
Write to Text File With the
open()
Method and Context Manager in Python -
Write to Text File With the
pathlib
Module in Python
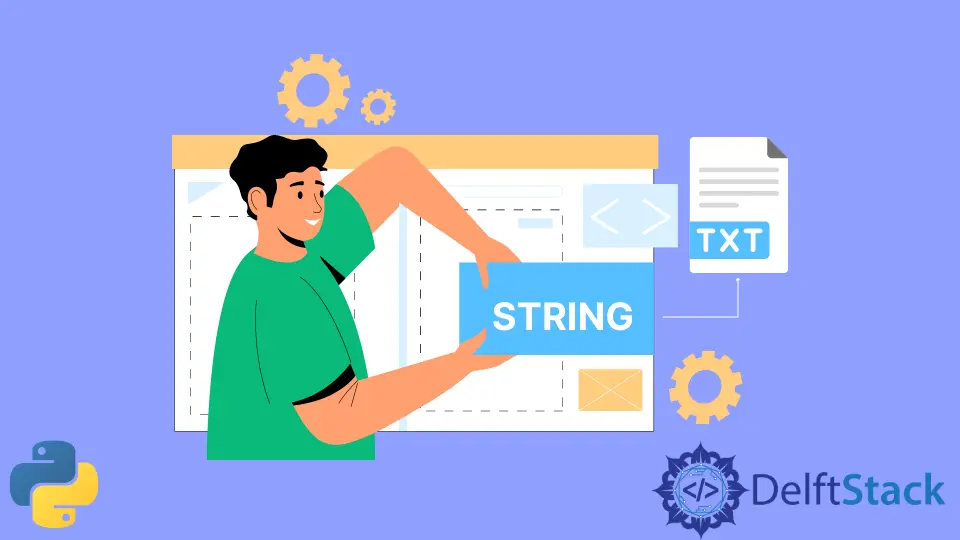
This tutorial will discuss the methods we can use to write data to a text file in Python.
Write to Text File With the open()
Method in Python
The built-in method open()
can interact with text files in Python. The open()
method takes the file path and the mode as input parameters, and it opens the file and returns its file object.
To read the data, we need to specify r
as the mode, and to write the data, we need to specify w
as the mode. Once we have opened a file in write mode, we can then access the write()
method of the previously returned file object to write data to the file.
After writing all the data to the file, we need to close our file with the close()
method. This is necessary if we want another program to be able to read our data, and if not done correctly, our data can get corrupted.
Code:
data = "This is some data"
File = open("File.txt", "w")
File.write("Data: %s" % data)
File.close()
File.txt:
Data: This is some data
In the above code, we opened the file File.txt
in write mode and wrote the data
inside this file with the write()
method. Although this method works well if we are careful enough to close our file every time we open it, it isn’t advisable.
Write to Text File With the open()
Method and Context Manager in Python
A context manager automatically closes a previously opened file when no read or write operations are performed. The syntax of the context manager involves writing a with
followed by the open()
and an alias.
This creates an indented block of code. Our file is only open inside the context of this block, and the context manager automatically closes the file when this block ends.
The following code example demonstrates how to utilize the open()
method with the context manager to write some data to a text file in Python.
Code:
data = "This is still some data"
with open("File.txt", "w") as File:
File.write("Data: %s" % data)
File.txt:
Data: This is still some data
We opened a file with the open()
method and context manager in the above code. The context manager method is far superior to the simple open()
method and should always be preferred over it while working with multiple files.
Write to Text File With the pathlib
Module in Python
The pathlib
module provides methods to interact with the file system of our machine in Python. We can use the Path()
method of the pathlib
module to open our desired text file.
The Path()
method takes the file’s path as an input parameter and returns its object. We can then use the previously returned object’s write_text()
method to write some data to our file.
Using the write_text
method, we don’t have to worry about explicitly opening and closing our files.
Code:
import pathlib
data = "This is some data"
File = pathlib.Path("File.txt")
File.write_text("Data: %s" % data)
File.txt:
Data: This is some more data
In the code, we wrote the data
variable inside our File.txt
file with the pathlib
module.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn