How to Prepend to a List in Python
-
Use the
insert()
Method to Prepend to a List in Python -
Use the
deque.appendleft()
Method to Prepend to a List in Python - Create a New List to Prepend to a List in Python
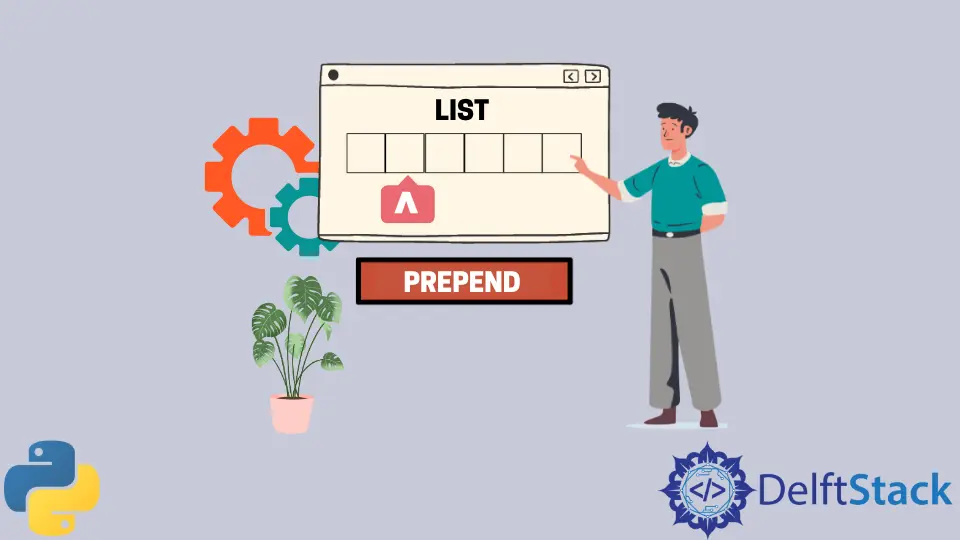
This tutorial describes how to prepend to a list in Python. The tutorial also lists some example codes to explain multiple ways of prepending to a list.
Use the insert()
Method to Prepend to a List in Python
Using insert()
is one of the prevalent and most used approaches. insert()
is provided by the list
library. The list.insert(pos, element)
takes two arguments, pos
and element
as its parameters. pos
defines the position of the element.
An example code to use this method is shown below:
lists = ["james", "tim", "jin"]
lists.insert(0, "steve")
print(lists)
Output:
['steve', 'james', 'tim', 'jin']
However, the time consumed by the list.insert()
operation is a little more. To improve the time performance, we can use the collections.deque
method.
Use the deque.appendleft()
Method to Prepend to a List in Python
The collections
module of Python offers multiple data structures. In Python 2.4, deque()
, a double-ended queue, is added to the collections
. It is a list like a container that is efficient in appending and pop up processes. The deque
data structure has an appendleft(element)
method. It takes an element and appends it at the beginning of the list.
An example code for this method is given below:
import collections
dequeue = collections.deque([5, 2, 6, 8, 1])
print(dequeue)
dequeue.appendleft(10)
print(dequeue)
Output:
deque([5, 2, 6, 8, 1])
deque([10, 5, 2, 6, 8, 1])
Create a New List to Prepend to a List in Python
A very simple and trivial solution can be creating a new list with the desired element, x, for example, at the 0th index of the list. Of course, you will not prepend x to the list but create a new list with x already at the first position in the list.
A basis code for this approach is given below.
lists = ["james", "tim", "jin"]
new_list = ["x"] + lists
print(new_list)
Output:
['x', 'james', 'tim', 'jin']
Use List Slicing to Prepend to a List in Python
List slicing is yet another method to prepend to a list. An element is prepended to the list by assigning the 0th
slice to it.
An example code for this method is as follows:
temp_list = [4, 5, 8, 10, 13]
print(temp_list)
temp_list[:0] = [12]
print(temp_list)
Output:
[4, 5, 8, 10, 13]
[12, 4, 5, 8, 10, 13]
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python