Positional Arguments vs Keyword Arguments in Python
- What Is an Argument in Python?
- What Is a Positional Argument in Python?
- What Is a Keyword Argument in Python?
- Positional Arguments vs. Keyword Arguments: What Should You Use?
- Conclusion
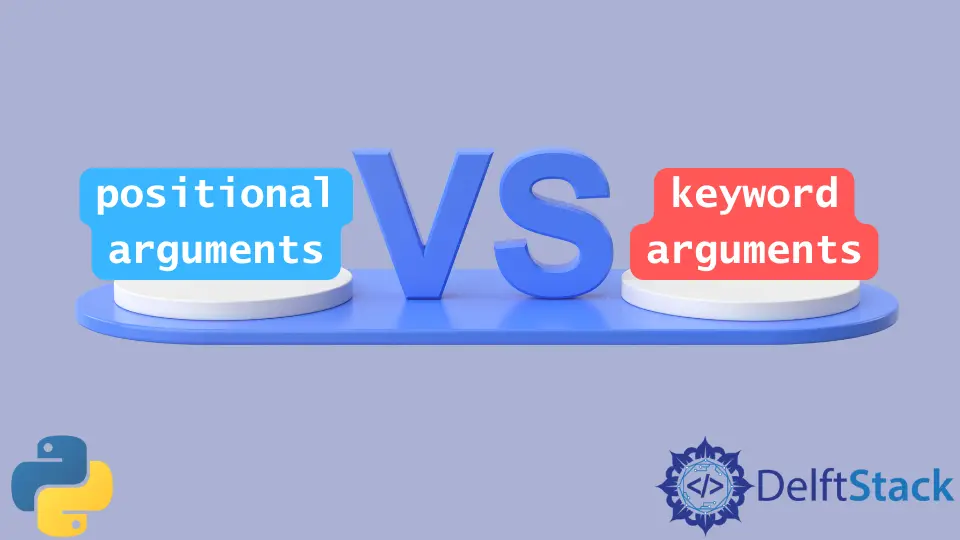
While calling a function in Python, we often need to pass input values to it. In this article, we will discuss positional arguments and keyword arguments in Python. We will also have a discussion on positional argument vs. keyword argument in which we will discuss the advantages and disadvantages of both these approaches to provide inputs to functions in Python.
What Is an Argument in Python?
To understand an argument, let us declare a function printDetails()
that takes four values, i.e. name
, identity_number
, age
, and weight
of a person, and then prints them.
def printDetails(name, identity_number, age, weight):
print("Name is:", name)
print("Identity Number is:", identity_number)
print("Age is:", age)
print("Weight is:", weight)
Here, name
, identity_number
, age
, and weight
are called parameters of the function printDetails()
. So, we can say that a parameter is part of the signature/declaration of a function.
Now, suppose that we want to call the function printDetails()
with the name
- Aditya
, identity_number
- TM2017001
, age
- 23
, and weight
- 65
as input. We can do it as follows.
def printDetails(name, identity_number, age, weight):
print("Name is:", name)
print("Identity Number is:", identity_number)
print("Age is:", age)
print("Weight is:", weight)
printDetails("Aditya", "TM2017001", 23, 65)
Output:
Name is: Aditya
Identity Number is: TM2017001
Age is: 23
Weight is: 65
Here, Aditya
, TM2017001
, 23
, and 65
are arguments of the function printDetails()
. Upon the execution of the function, the value Aditya
is assigned to the parameter name
, TM2017001
is assigned to the parameter identity_number
, 23
is assigned to the parameter age
, and 65
is assigned to the parameter weight
. Hence, we can say that an argument is a value that is passed to a function, and a parameter is the variable declared in the function to which the argument is assigned.
To assign an argument to a parameter, we can either pass the values in the order in which the parameters are declared in the function. Alternatively, we can also directly assign the arguments to the parameters. Based on this, arguments in Python are classified as positional arguments and keyword arguments. Let us discuss them one by one.
What Is a Positional Argument in Python?
When we pass an argument to a function directly, and it is assigned to a parameter based on its position, then it is called a positional argument. For instance, when we call the function printDetails()
with values Aditya
, TM2017001
, 23
, and 65
as follows,
printDetails("Aditya", "TM2017001", 23, 65)
All the input arguments will be assigned to the parameters based on their position in the function call and the position of parameters in the function declaration. Let us write the function call and function declaration together to understand this in a better way.
def printDetails(name, identity_number, age, weight):
print("Name is:", name)
print("Identity Number is:", identity_number)
print("Age is:", age)
print("Weight is:", weight)
printDetails("Aditya", "TM2017001", 23, 65)
Output:
Name is: Aditya
Identity Number is: TM2017001
Age is: 23
Weight is: 65
Here, you can observe that the printDetails() function has parameters in the order of name
, identity_number
, age
, and weight
. Hence, Aditya
is assigned to name
, TM2017001
is assigned to identity_number
, 23
is assigned to age
, and 65
is assigned to weight
.
What Is a Keyword Argument in Python?
Instead of passing only values in the function, we can assign them directly to the parameters as follows.
printDetails(name="Aditya", identity_number="TM2017001", age=23, weight=65)
Here, each parameter works like a key, and each argument works as a value. Hence, the arguments are termed keyword arguments. On execution, the function works in the same way as it did with positional arguments. You can observe this in the following example.
def printDetails(name, identity_number, age, weight):
print("Name is:", name)
print("Identity Number is:", identity_number)
print("Age is:", age)
print("Weight is:", weight)
printDetails(name="Aditya", identity_number="TM2017001", age=23, weight=65)
Output:
Name is: Aditya
Identity Number is: TM2017001
Age is: 23
Weight is: 65
Positional Arguments vs. Keyword Arguments: What Should You Use?
If we talk about execution, both the positional arguments and keyword arguments have the same efficiency. The choice of using any of these approaches depends on your convenience.
While using positional arguments, changing the position of input arguments may attract unwanted results. For instance, look at the following example.
def printDetails(name, identity_number, age, weight):
print("Name is:", name)
print("Identity Number is:", identity_number)
print("Age is:", age)
print("Weight is:", weight)
printDetails(65, "TM2017001", 23, "Aditya")
Output:
Name is: 65
Identity Number is: TM2017001
Age is: 23
Weight is: Aditya
Here, the 65
has been assigned to the parameter name
and Aditya
has been assigned to the parameter weight
. So, it is important to pass the positional arguments in the same order in which the corresponding parameters have been defined in the function declaration.
On the other hand, we can pass the arguments in any order while using keyword arguments. It does not affect the output of the function. You can observe this in the following example.
def printDetails(name, identity_number, age, weight):
print("Name is:", name)
print("Identity Number is:", identity_number)
print("Age is:", age)
print("Weight is:", weight)
printDetails(weight=65, identity_number="TM2017001", age=23, name="Aditya")
Output:
Name is: Aditya
Identity Number is: TM2017001
Age is: 23
Weight is: 65
So, if you want to avoid any possible error due to a change in position of the arguments, using the keyword arguments would be better for you.
We can also use positional and keyword arguments in a single function call, as shown in the following program.
def printDetails(name, identity_number, age, weight):
print("Name is:", name)
print("Identity Number is:", identity_number)
print("Age is:", age)
print("Weight is:", weight)
printDetails("Aditya", "TM2017001", age=23, weight=65)
Output:
Name is: Aditya
Identity Number is: TM2017001
Age is: 23
Weight is: 65
Here, you can observe that positional arguments are passed to the function at the same place where the corresponding parameter has been defined. In comparison, keyword arguments can be passed in any order. Also, we cannot pass positional arguments after passing a keyword argument.
Once we pass a keyword argument, all the remaining arguments need to be passed as keyword arguments. Otherwise, the program will run into an error, as you can observe in the following example.
def printDetails(name, identity_number, age, weight):
print("Name is:", name)
print("Identity Number is:", identity_number)
print("Age is:", age)
print("Weight is:", weight)
printDetails("Aditya", identity_number="TM2017001", 23, weight=65)
Output:
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 8
printDetails("Aditya", identity_number="TM2017001", 23, weight=65)
^
SyntaxError: positional argument follows keyword argument
So, you can choose positional arguments, or keyword arguments, or both in the same function call according to your convenience.
Conclusion
In this article, we studied positional arguments and keyword arguments in Python. We also discussed positional argument vs. keyword argument and concluded that both approaches are equal in terms of execution efficiency. We can even use them together in a single function call. Just keep in mind that we cannot use a positional argument after a keyword argument.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHubRelated Article - Python Function
- How to Exit a Function in Python
- Optional Arguments in Python
- How to Fit a Step Function in Python
- Built-In Identity Function in Python
- Arguments in the main() Function in Python
- Python Functools Partial Function