How to Pass Kwargs to Another Function in Python
- Keyword Arguments in Python
-
Call Function With
**kwargs
in Python -
Pass
kwargs
to Another Function With Python - Conclusion
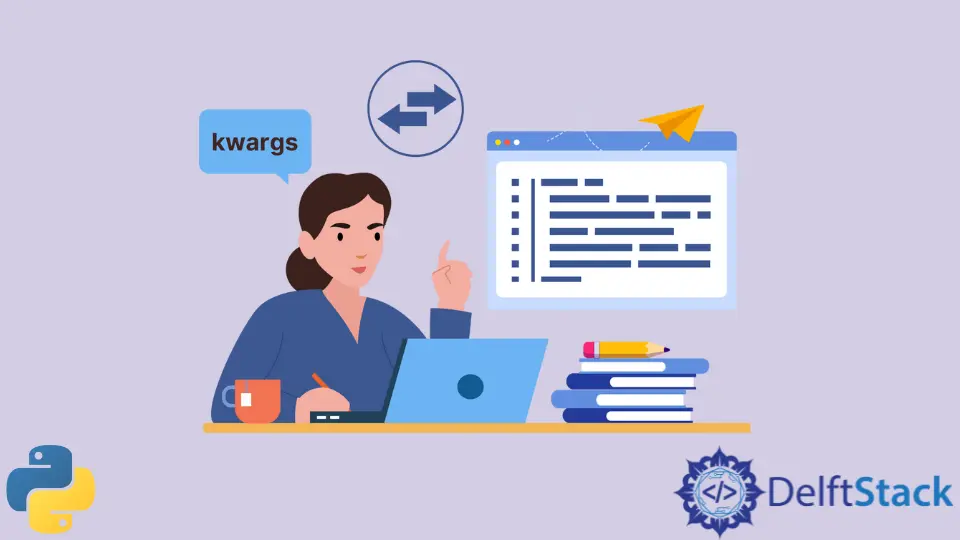
Python lists the two types of arguments that can be passed to functions in the program. The Non-keyword Arguments ( **args
) and Keyword Argument (**kwargs
) .
Usually, python functions have to call with the correct number of arguments. If the function expects two arguments, we should pass only two.
In this article, we’re going to discuss how to use keyword arguments and how to pass keyword arguments to another function.
Keyword Arguments in Python
Generally, arguments do pass information into functions. Keyword Argument is one method that can be used to pass arguments to the function.
Especially using Keyword Arguments, we can send arguments with key = value
syntax. Let’s look at the following example.
def keyword_function(fname, lname, age):
print("first name is " + fname)
keyword_function(fname="Anne", lname="Steaven", age=27)
This example defined a function called keyword_function
. It passes three arguments named fname
, lname
, and age
.
So, these arguments are sent as keyword arguments because when the function calls in the program, it takes key and value pairs for each argument.
Output:
In the above output, we print a statement passing the fname
argument.
Call Function With**kwargs
in Python
Mainly Keyword Arguments can be used when several arguments are unknown. In that case, add two asterisks(**
) before the parameter name in the function definition.
If the function has a **kwargs
parameter, it accepts a variable number of keyword arguments as a dictionary.
Give thought to the following example.
def animals(**kwargs):
print(kwargs)
animals(name1="Rabbit", name2="Dog", name3="Monkey")
There is a function called animals
in the above code. It has a parameter called **kwargs
, the function call with three arguments as keyword arguments.
Output:
We can see the result as a dictionary with two pairs of values and keys. The kwargs
argument is convention.
So, as a parameter name, we can use any meaningful name. As the same, this is how they call a function with the **kwargs
parameter.
Pass kwargs
to Another Function With Python
Code:
def info(**kwargs):
x = dict(name="shen", age=27, degree="SE")
pass_func(**x)
def pass_func(**kwargs):
for i in kwargs:
print(i, kwargs[i])
info()
Using the above code, we print information about the person, such as name
, age
, and degree
. This program passes kwargs
to another function which includes variable x
declaring the dict
method.
Example defined function info
without any parameter. The function info
declared a variable x
which defined three key-value pairs, and usually, the key-value pair define in dictionary type in Python.
Consequently, the dict
method specifies pair of keys and values. Since kwargs
unpack arguments are declared as a dictionary type, we can use the dict
method for this example.
Then we have another function called pass_func
, which passes kwargs
arguments. This function includes a for
loop that prints kwargs
arguments, and after that, we can pass the kwargs
function to the info
function to print x
.
So, we call pass_func
with the kwargs
argument to the x
variable. Finally, we call the info
function in the pass_func
function.
Output:
Conclusion
Throughout the article, we focused on how to pass kwargs
to another function. The kwargs
arguments can get several variables without a defined parameter for each argument.
When passing the kwargs
argument to the function, It must use double asterisks with the parameter name **kwargs
. When passing kwargs
to another function, first, create a parameter with two asterisks, and then we can pass that function to another function as our purpose.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.