Matrix Multiplication in OpenCV
- Matrix Multiplication Using Asterisk Operator in OpenCV
-
Use the
multiply()
Function to Multiply Two Matrices in OpenCV -
Use the
cv2.gemm()
Function to Multiply Two Matrices in OpenCV - Conclusion
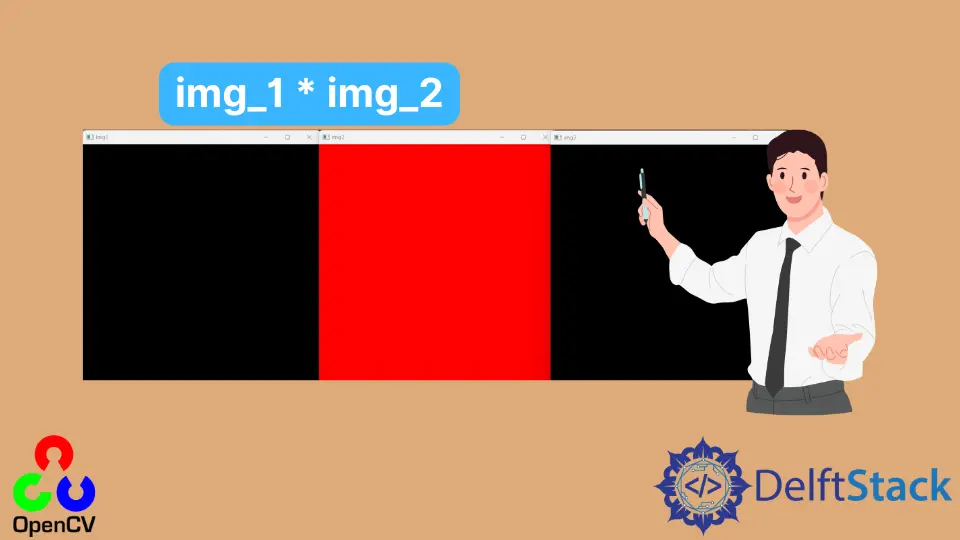
OpenCV, a widely-used library in Python, offers a range of functionalities for image manipulation and matrix operations. In this guide, we will explore different methods of multiplying matrices, each serving unique purposes in various image processing contexts.
This tutorial is designed to be informative for beginners yet detailed enough for experienced users, providing insights into the intricacies of matrix multiplication in OpenCV.
Matrix Multiplication Using Asterisk Operator in OpenCV
In OpenCV, we can multiply two images using the asterisk operator. Images are stored in a matrix in OpenCV, so we can use the asterisk operator to multiply two matrices.
In this case, the multiplication will be direct, the matrix X
of size (i x j)
will be multiplied with a matrix Y
of size (k x l)
, and it will produce a third matrix Z
of size (i x l)
.
For example, let’s use NumPy
to create two images, one with black color and one with red color, and then multiply them using the asterisk operator and show them using the imshow()
function of OpenCV.
import cv2
import numpy as np
img_1 = np.zeros((512, 512, 3), dtype=np.float32)
img_2 = np.zeros((512, 512, 3), dtype=np.float32)
img_2[:, :] = (0, 0, 255)
img_3 = img_1 * img_2
cv2.imshow("Img1", img_1)
cv2.imshow("img2", img_2)
cv2.imshow("img3", img_3)
cv2.waitKey(0)
In this example, we start by importing two essential libraries: cv2
for image processing operations and numpy
for array manipulations. We then create two images, img_1
and img_2
, using numpy.zeros
.
This function initializes arrays with the specified shape of 512x512 pixels, each pixel comprising 3 color channels (RGB). We choose float32
arrays for precision, a common practice in image processing.
Next, we assign a color to img_2
. By setting img_2[:, :] = (0, 0, 255)
, we fill the entire array with blue, following OpenCV’s BGR color representation. Then comes the element-wise multiplication: img_3 = img_1 * img_2
.
Since img_1
consists entirely of zeros, multiplying it with img_2
results in img_3
being an array of zeros as well.
Finally, we display these images using OpenCV’s cv2.imshow
function. img_1
appears as black, img_2
as red, and img_3
, the product of the multiplication, also as black. We use cv2.waitKey(0)
to keep the displayed windows open until a key press, allowing us to view the images.
Output:
Use the multiply()
Function to Multiply Two Matrices in OpenCV
OpenCV, a popular library in Python for these purposes, provides various methods for matrix manipulation. One such method is cv2.multiply
, which facilitates element-wise multiplication of matrices.
Element-wise multiplication, as performed by cv2.multiply
, is used extensively in operations like image blending, masking, and filtering. Unlike typical matrix multiplication, element-wise multiplication multiplies corresponding elements in the matrices.
import cv2
import numpy as np
# Create matrices A and B
A = np.array([[1, 2], [3, 4]], dtype=np.float64)
B = np.array([[5, 6], [7, 8]], dtype=np.float64)
# Element-wise multiplication using cv2.multiply
C = cv2.multiply(A, B)
# Display matrices and result
print("Matrix A:\n", A)
print("\nMatrix B:\n", B)
print("\nMatrix C (Result of element-wise multiplication of A and B):\n", C)
In this example, we demonstrate the use of cv2.multiply
for element-wise multiplication. We first define two matrices, A
and B
, using NumPy arrays.
These matrices are then multiplied element-wise utilizing the cv2.multiply
method. The resulting matrix, C
, stores the outcome of this operation.
Finally, we employ print
statements to display the contents of matrices A
, B
, and C
.
Output:
Use the cv2.gemm()
Function to Multiply Two Matrices in OpenCV
The cv::gemm
method (General Matrix Multiplication) is used for high-performance matrix multiplications and transformations. It’s especially useful in scenarios where you need to scale and add matrices in a single operation, like in affine or perspective transformations, or when working with large datasets in machine learning.
This method is designed for performing more general matrix multiplication, adhering to the formula: C = alpha * A * B + beta * C
.
import cv2
import numpy as np
# Create matrices
A = np.array([[1, 2], [3, 4]], dtype=np.float32)
B = np.array([[5, 6], [7, 8]], dtype=np.float32)
C = np.array([[9, 8], [7, 6]], dtype=np.float32)
# Perform general matrix multiplication
alpha, beta = 1, 1
result = cv2.gemm(A, B, alpha, C, beta)
# Display the result
print("Result of cv::gemm operation:\n", result)
In our code snippet, we delve into the process of general matrix multiplication using cv2.gemm
. Initially, we create three matrices, namely A
, B
, and C
, utilizing NumPy for this purpose.
Following this, we engage the cv2.gemm
function, where we input these matrices along with the scaling factors alpha
and beta
.
For the sake of simplicity, we set both of these factors to 1. This approach simplifies our calculation to result = A * B + C
, allowing us to directly observe the interaction and combined effect of these matrices through the cv::gemm
operation.
To visualize our results and verify the operation, we use the print
function at the end. This function outputs the final matrix, result
, to the console.
Output:
Conclusion
Throughout this tutorial, we have journeyed through various methods of matrix multiplication in OpenCV, gaining insights into their applications and nuances. Starting with the asterisk operator, we saw how simple multiplication can have significant effects in image processing, such as altering color intensities.
Then, with the cv2.multiply
method, we explored the realm of element-wise multiplication, a technique pivotal in many image manipulation tasks. Finally, the cv2.gemm()
method opened doors to more sophisticated operations, highlighting its importance in complex image transformations and machine learning applications.
Each method, unique in its functionality, plays a critical role in the vast world of computer vision and image processing.