How to Use Erode in OpenCV
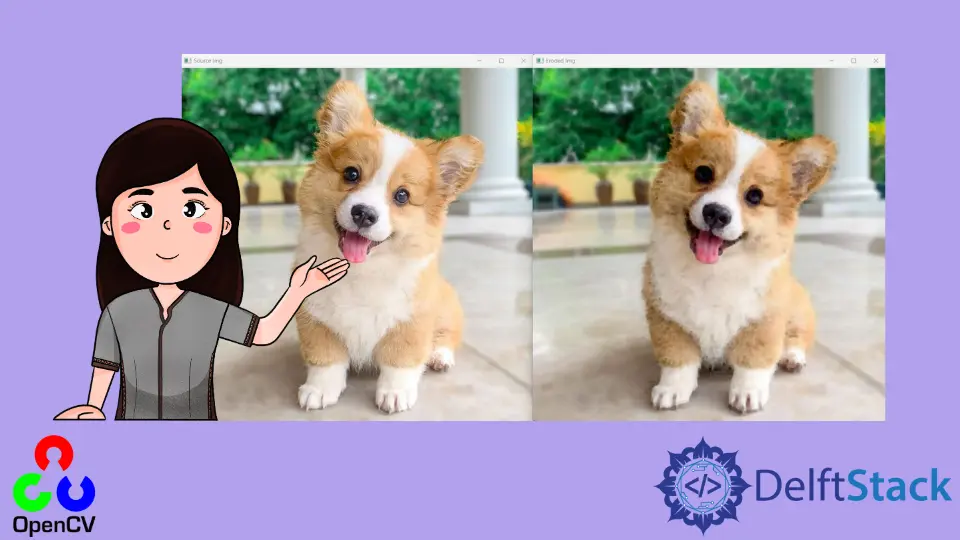
In OpenCV, erosion increases the dark areas present in an image using a specific structuring element. We can use the erode()
function of OpenCV to find the erosion of an image.
Use the erode()
Function to Find the Erosion of an Image in OpenCV
The erode()
function finds the local minimum over the area of a given structuring element.
We need a structuring element to find the erosion of an image. We can use the getStructuringElement()
function to create a kernel of a specific shape like a rectangle.
After that, we need to pass the given image along with the kernel inside the erode()
function, and it will find the erosion of the given image.
For example, let’s read an image of a cat using the imread()
function and increase the dark areas present in it using the erode()
function.
Then show it along with the original image using the imshow()
function of OpenCV.
import cv2
src_img = cv2.imread("cat.jpg")
kernel_img = cv2.getStructuringElement(cv2.MORPH_RECT, (5, 5))
image_erode = cv2.erode(src_img, kernel_img)
cv2.imshow("Source Img", src_img)
cv2.imshow("Eroded Img", image_erode)
cv2.waitKey()
cv2.destroyAllWindows()
We used a 5-by-5 structuring
element for erosion, but we can use any size for the structuring element. The greater the size of the structuring element, the greater the increase in the dark areas.
Output:
As we can see in the right side image, the dark areas have been increased depending on the size of the structuring element.
The Three optional arguments that we can set in the getStructuringElement()
function are:
- The first argument is the shape. We used the rectangular shape in the above code, but we can use other shapes like cross shape using the
cv2.MORPH_CROSS
argument and ellipse shape using thecv2.MORPH_ELLIPSE
argument. - The second argument is the size of the structuring element.
- The last argument is the location of the anchor point. By default, the anchor point’s location is set to
(-1, -1)
, which corresponds to the center, but we can change it to any location within the element.
There are four optional arguments that we can set in the erode()
function, which are:
- The first optional argument that we can change is the location of the anchor, which by default is set to
(-1, 1)
. - The second optional argument is the number of iterations, which is set to 1, but we can set it to any number.
- The third optional argument is the border type, which is set to the constant border by default, but we can change it to other types like reflect border using the
cv2.BORDER_REFLECT
argument. Check this link for more details about the border types. - The last optional argument is the value of the border in case of a constant border.
If we want to increase the bright areas present in an image instead of increasing the dark areas, we can use the dilate()
function of OpenCV.