OpenCV Convolution
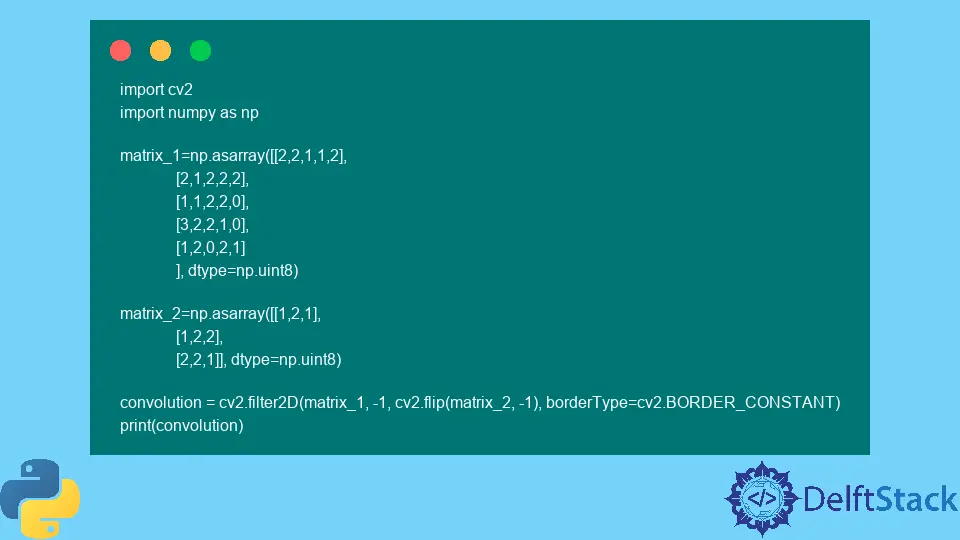
This tutorial will discuss finding the convolution of two matrices or images using the filter2D()
function of OpenCV in Python.
Use the filter2D()
Function of OpenCV to Find Convolution of Matrices or Images in Python
We can use the filter2D()
function of OpenCV to find the convolution of two matrices or an image with a kernel. The filter2D()
function finds the correlation between two matrices, but we can also use it to find the convolution.
To find the convolution between two matrices, we have to flip the second matrix or kernel using the flip()
function and then pass them inside the filter2D()
function, and it will return the convolution of the given matrices.
We also have to set the border type to the constant border using the borderType
argument.
For example, let’s create two matrices and find their convolution using the filter2D
function. See the code below.
import cv2
import numpy as np
matrix_1 = np.asarray(
[
[2, 2, 1, 1, 2],
[2, 1, 2, 2, 2],
[1, 1, 2, 2, 0],
[3, 2, 2, 1, 0],
[1, 2, 0, 2, 1],
],
dtype=np.uint8,
)
matrix_2 = np.asarray([[1, 2, 1], [1, 2, 2], [2, 2, 1]], dtype=np.uint8)
convolution = cv2.filter2D(
matrix_1, -1, cv2.flip(matrix_2, -1), borderType=cv2.BORDER_CONSTANT
)
print(convolution)
Output:
[[11 15 14 14 12]
[16 21 21 23 15]
[17 23 24 22 11]
[16 24 22 17 8]
[14 17 14 9 7]]
The first argument of the filter2D()
function is the input matrix or image we want to convolve with a kernel or matrix. The second argument is the depth of the output image, and if it is set to -1, the output image will have the same depth as the input image.
Check this link for more details about the depth combinations. The third argument is the kernel or the matrix to which we want to convolve the input matrix or image.
The fourth argument is optional and is used to set the anchor point, which by default is set to (-1, -1) or to the center of the kernel. The fifth argument is also optional and is used to set the delta value added to the filtered pixels, and by default, it is set to 0.
The sixth argument is also optional and is used to set the border type or pixel extrapolation method, and by default, it is set to default border. Check this link for more details about the different border types.