Nested Dictionary Comprehension in Python
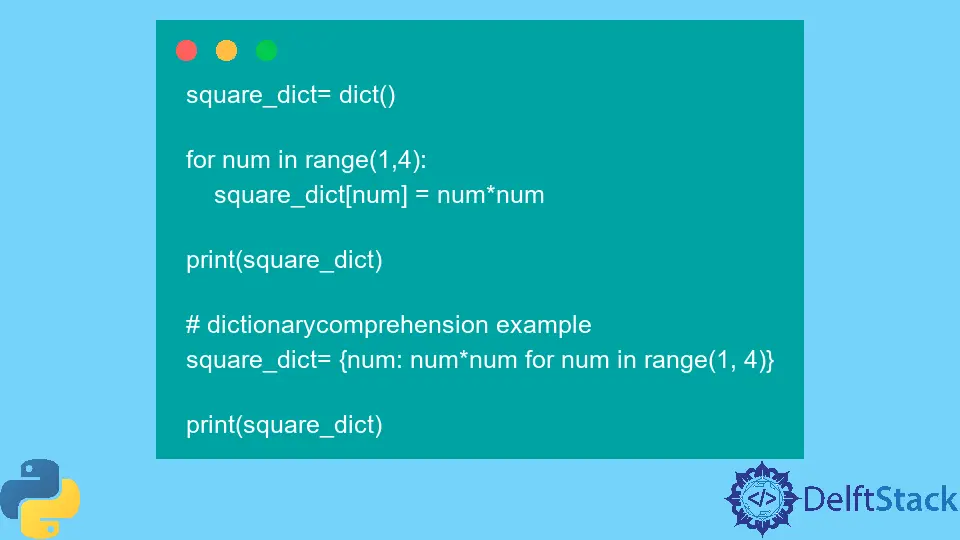
Python dictionary comprehension is a powerful tool that allows you to create new dictionaries from existing ones. It is similar to a list comprehension, but instead of creating a list, it creates a dictionary.
Dictionary comprehension is a very concise way to create a dictionary and is useful when you want to make a dictionary from a couple of tuples, where one tuple contains the key and value.
In this tutorial, we will explore Python nested dictionary comprehension, its use and the advantages of using it in Python.
Python Dictionary Comprehension
Dictionary comprehension is a powerful tool that we can use to perform various operations on dictionaries, such as filtering, transforming, or creating new dictionaries. It normally involves the one line for
loop.
Dictionary comprehensions allow you to create dictionaries from other dictionaries or other iterable
datatypes. They also allow you to specify key-value pairs instead of just values.
Dictionary comprehensions are most commonly used to create new dictionaries from existing ones. For example, you could create a new dictionary containing only the keys from an existing dictionary or the values.
You could also create a new dictionary that contains the keys and values from two existing dictionaries. We can also use dictionary comprehensions to manipulate existing dictionaries.
For example, you could add or remove items from a dictionary or change the values of existing items.
Code Example:
square_dict = dict()
for num in range(1, 4):
square_dict[num] = num * num
print(square_dict)
# dictionarycomprehension example
square_dict = {num: num * num for num in range(1, 4)}
print(square_dict)
Output:
{1: 1, 2: 4, 3: 9}
{1: 1, 2: 4, 3: 9}
Syntax of Dictionary Comprehension
Dictionary comprehension is a handy tool for creating dictionaries from other data structures in Python. The syntax is straightforward to read {key: value for (key, value) in iterable}
.
Code Example:
# The price of the item in dollars
old_price = {"price of milk": 2, "price of coffee": 4, "price of bread": 3.5}
dollar_to_pound = 0.76
new_price = {item: value * dollar_to_pound for (item, value) in old_price.items()}
print(new_price)
Output:
{'price of milk': 1.52, 'price of coffee': 3.04, 'price of bread': 2.66}
Python Nested Dictionary Comprehension
Nested dictionary comprehension works by iterating over a sequence of key-value pairs and then constructing a new dictionary from those pairs. The new dictionary can be built using any mapping function, such as dict.update
or dict.fromkeys
.
For example, consider the following data structure:
data = {"a": 1, "b": 2, "c": 3, "d": 4}
To synthesize a new dictionary that maintains only the keys from this data structure, you could use the following nested dictionary comprehension:
new_dict = {k: data[k] for k in data}
print(new_dict)
It would produce a new dictionary that looks like this:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
You could use the following nested dictionary comprehension to synthesize a new dictionary that maintains only the values from this data structure.
new_dict = {k: v for k, v in data.items()}
It would produce a new dictionary that looks like this:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
As you can see, nested dictionary comprehension is a powerful tool that can quickly and efficiently process and manipulate complex data structures. It is also an easy way to create ad-hoc data structures.
Syntax of Nested Dictionary Comprehension in Python
To create a nested dictionary comprehension, put another dictionary comprehension within the brackets of the first dictionary comprehension.
The following example creates a dictionary of dictionaries, with each inner dictionary containing information about a different fruit
:
{key:value for key, value in outer_dict.items() if key =='fruit'}
{key:{'name':fruit['name'], 'colour':fruit['colour']} for key,fruit in outer_dict.items() if key == 'fruit'}
The first dictionary comprehension above creates a dictionary with keys that are the names of fruits
and values that are the colours
of those fruits
.
The second dictionary comprehension above does the same thing but adds an extra level of nesting, creating a dictionary of dictionaries.
Code Example:
dictionary = {k1: {k2: k1 * k2 for k2 in range(1, 3)} for k1 in range(2, 5)}
print(dictionary)
Output:
{2: {1: 2, 2: 4}, 3: {1: 3, 2: 6}, 4: {1: 4, 2: 8}}
Advantages of Nested Dictionary Comprehension
There are a few advantages to using nested dictionary comprehensions in Python.
- We can use them to create complex nested dictionaries easily.
- We can use them to process large amounts of data efficiently.
- Finally, we can use them to create new dictionaries based on existing ones quickly.
So, understanding how nested dictionary comprehension works, you can quickly and efficiently work with complex data in Python.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn