Nested Class in Python
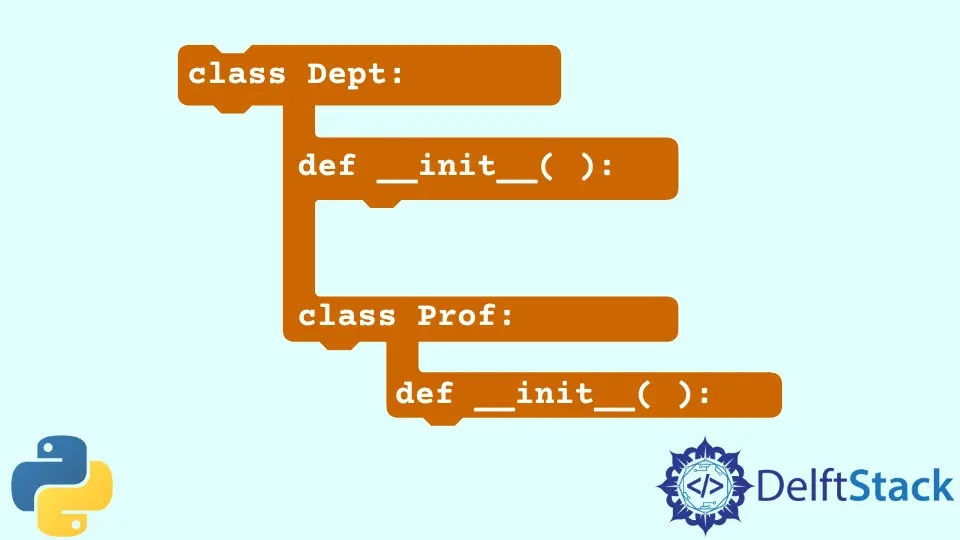
Classes contain different data members and functions and allow us to create objects to access these members.
Python, as an object-oriented programming language, has many such objects of different classes. In Python we have an important constructor called __init__
, which is called every time an instance of the class is created, and we also have the self
keyword to refer to the current instance of the class.
A nested class (also called an inner class) is defined within another class. It is very commonly used in all object-oriented programming languages and can have a lot of benefits. It doesn’t improve the execution time but can aid in program readability and maintenance by grouping related classes together and also hide the nested class from the outside world.
The following code shows a very simple structure of a nested class:
class Dept:
def __init__(self, dname):
self.dname = dname
class Prof:
def __init__(self, pname):
self.pname = pname
math = Dept("Mathematics")
mathprof = Dept.Prof("Mark")
print(math.dname)
print(mathprof.pname)
Output:
Mathematics
Mark
Note that we cannot access the inner class directly. We create its object using the outer.inner
format.
We can access the nested class in the outer class but not the other way around. To access the nested class in the outer class, we can either use the outer.inner
format or the self
keyword.
In the code below, we make some alterations to the above classes and access a function of the nested class using the parent class:
class Dept:
def __init__(self, dname):
self.dname = dname
self.inner = self.Prof()
def outer_disp(self):
self.inner.inner_disp(self.dname)
class Prof:
def inner_disp(self, details):
print(details, "From Inner Class")
math = Dept("Mathematics")
math.outer_disp()
Output:
Mathematics From Inner Class
We can also have multiple nested classes in Python. Such situations are called Multiple Nested Classes with one or more inner classes.
There can also be some cases where we have a nested class within a nested class, and this is called a multilevel nested class.
The following code shows a very simple example of a multilevel nested class.
class Dept:
def __init__(self, dname):
self.dname = dname
class Prof:
def __init__(self, pname):
self.pname = pname
class Country:
def __init__(self, cname):
self.cname = cname
math = Dept("Mathematics")
mathprof = Dept.Prof("Mark")
country = Dept.Prof.Country("UK")
print(math.dname, mathprof.pname, country.cname)
Output:
Mathematics Mark UK
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn