Python のネストされたクラス
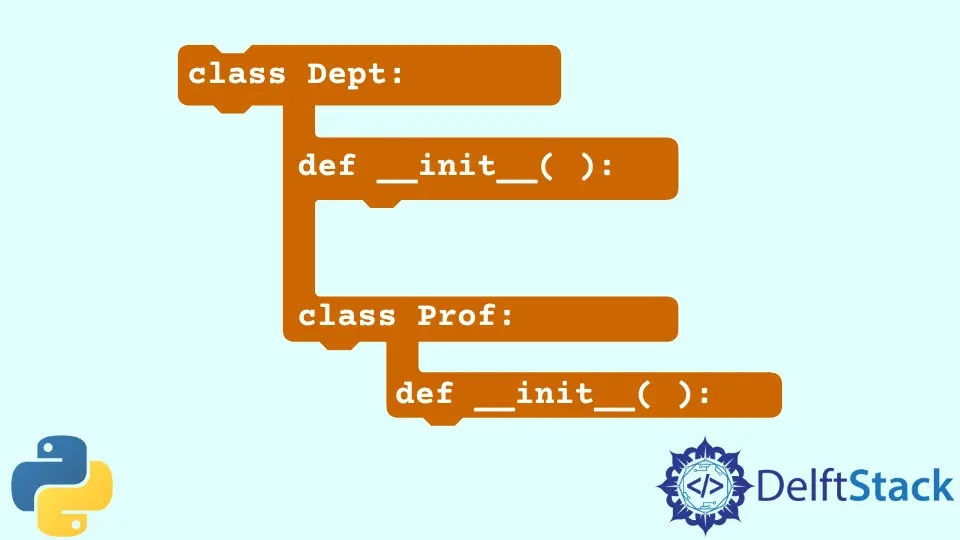
クラスには異なるデータのメンバや関数が含まれており、これらのメンバにアクセスするためのオブジェクトを作成することができます。
オブジェクト指向プログラミング言語である Python には、このような異なるクラスのオブジェクトがたくさんあります。Python では __init__
という重要なコンストラクタがあり、これはクラスのインスタンスが作成されるたびに呼び出され、また、クラスの現在のインスタンスを参照するための self
キーワードも持っています。
入れ子になったクラス (内部クラスとも呼ばれます) は、別のクラスの中で定義されます。これはすべてのオブジェクト指向プログラミング言語で非常に一般的に使われており、多くの利点があります。実行時間を改善するわけではありませんが、関連するクラスをまとめてグループ化することで、プログラムの可読性やメンテナンスを助けたり、入れ子になったクラスを外部から隠したりすることができます。
次のコードは、入れ子になったクラスの非常にシンプルな構造を示しています。
class Dept:
def __init__(self, dname):
self.dname = dname
class Prof:
def __init__(self, pname):
self.pname = pname
math = Dept("Mathematics")
mathprof = Dept.Prof("Mark")
print(math.dname)
print(mathprof.pname)
出力:
Mathematics
Mark
内部クラスに直接アクセスできないことに注意してください。オブジェクトは outer.inner
形式で作成します。
外部クラスの入れ子のクラスにはアクセスできますが、その逆はできません。外部クラスの入れ子になったクラスにアクセスするには、outer.inner
形式か self
キーワードを利用します。
以下のコードでは、上記のクラスに少し手を加えて、親クラスを使って入れ子クラスの関数にアクセスしています。
class Dept:
def __init__(self, dname):
self.dname = dname
self.inner = self.Prof()
def outer_disp(self):
self.inner.inner_disp(self.dname)
class Prof:
def inner_disp(self, details):
print(details, "From Inner Class")
math = Dept("Mathematics")
math.outer_disp()
出力:
Mathematics From Inner Class
Python では複数の入れ子クラスを持つこともできます。このような状況は、1つ以上の内部クラスを持つ複数のネストされたクラスと呼ばれています。
また、ネストされたクラスの中にネストされたクラスを持つ場合もあり、これをマルチレベルネストクラスと呼びます。
次のコードは、マルチレベルネストクラスの非常に簡単な例を示しています。
class Dept:
def __init__(self, dname):
self.dname = dname
class Prof:
def __init__(self, pname):
self.pname = pname
class Country:
def __init__(self, cname):
self.cname = cname
math = Dept("Mathematics")
mathprof = Dept.Prof("Mark")
country = Dept.Prof.Country("UK")
print(math.dname, mathprof.pname, country.cname)
出力:
Mathematics Mark UK
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn