How to Multiply Two Lists in Python
-
Multiply Two Lists in Python Using the
zip()
Method -
Multiply Two Lists in Python Using the
numpy.multiply()
Method -
Multiply Two Lists in Python Using the
map()
Function - Multiply Two Lists in Python Using a Loop
-
Multiply Two Lists in Python Using
operator.mul()
- Multiply Two Lists in Python Using a Recursive Method
- Conclusion
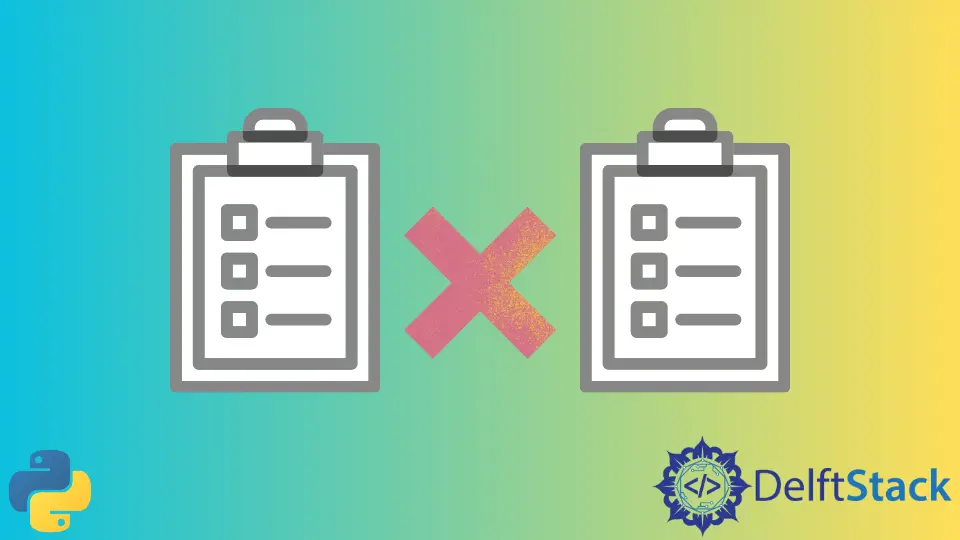
Multiplying two lists element-wise is a fundamental operation in Python, often encountered in various data manipulation and mathematical computations.
This article explores diverse methods for achieving element-wise list multiplication, providing a detailed overview of each approach.
Multiply Two Lists in Python Using the zip()
Method
The built-in [zip()
method]({{relref “/HowTo/Python/zip python 3.en.md”}}) in Python takes one or more iterables and aggregates them into tuples. For instance, applying zip()
to the lists [1, 2, 3]
and [4, 5, 6]
results in [(1, 4), (2, 5), (3, 6)]
.
When combined with other methods like map()
and list comprehension, zip()
becomes a handy tool for element-wise operations on lists.
Syntax of the zip()
method:
zip(iterable1, iterable2, ...)
Here, iterable1
, iterable2
, and ...
represent the iterables you want to combine. The zip()
method returns an iterator of tuples, where the i-th tuple contains the i-th element from each of the input iterables.
Let’s consider a scenario where we have two 1D lists, list1
and list2
, and we want to multiply their elements element-wise using the zip()
method.
list1 = [2, 4, 5, 3, 5, 4]
list2 = [4, 1, 2, 9, 7, 5]
product = [x * y for x, y in zip(list1, list2)]
print(product)
In this example, we declare two lists, list1
and list2
. The zip(list1, list2)
combines corresponding elements into tuples.
Here, the list comprehension [x * y for x, y in zip(list1, list2)]
iterates through these tuples, multiplies the elements, and forms a new list, product
, with the results.
Output:
[8, 4, 10, 27, 35, 20]
Each element in the new list corresponds to the product of elements at the same position in the original lists.
Let’s have another example. Consider two 2D lists, list1
and list2
, and perform element-wise multiplication using the zip()
method.
list1 = [[2, 4, 5], [3, 5, 4]]
list2 = [[4, 1, 2], [9, 7, 5]]
product = [[0] * 3] * 2
for x in range(len(list1)):
product[x] = [a * b for a, b in zip(list1[x], list2[x])]
print(product)
Using a for
loop, we iterate over the rows of the lists, and for each row, we employ the zip()
method to combine corresponding elements into tuples. The list comprehension [a * b for a, b in zip(list1[x], list2[x])]
then multiplies these elements, forming a new row in the product
list.
It’s worth noting that initializing the product
list using [[0] * 3] * 2
creates references to the same inner list, which might lead to unexpected behavior. A safer approach is to use a nested loop or list comprehension for initialization.
Output:
[[8, 4, 10], [27, 35, 20]]
Multiply Two Lists in Python Using the numpy.multiply()
Method
In Python, the numpy
library provides efficient tools for numerical operations. One such method is numpy.multiply()
, which enables element-wise multiplication of two arrays or lists.
This method simplifies the process of multiplying corresponding elements in arrays or lists, eliminating the need for explicit loops.
Syntax of the numpy.multiply()
method:
import numpy
numpy.multiply(x1, x2, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True[, signature, extobj])
Here, x1
and x2
are the input arrays or lists to be multiplied.
The /
indicates positional-only arguments, while the *
signifies keyword-only arguments. The method returns the element-wise product of the input arrays.
Before using the numpy.multiply()
method, make sure you have NumPy installed. If you don’t have it installed, you can use the following command in your terminal or command prompt to install it:
pip install numpy
Now, let’s dive into an example:
import numpy as np
list1 = [12, 3, 1, 2, 3, 1]
list2 = [13, 2, 3, 5, 3, 4]
product = np.multiply(list1, list2)
print(product)
As you can see, we begin by importing the numpy
library as np
. We then declare two lists, list1
and list2
.
The line np.multiply(list1, list2)
efficiently multiplies the corresponding elements of the two lists, producing a new array, product
, with the results.
Output:
[156 6 3 10 9 4]
Let’s see another example where we have two 2D lists, list1
and list2
, and perform element-wise multiplication using the numpy.multiply()
method.
import numpy as np
list1 = [[12, 3, 1], [2, 3, 1]]
list2 = [[13, 2, 3], [5, 3, 4]]
product = np.multiply(list1, list2)
print(product)
In this example, the line np.multiply(list1, list2)
efficiently multiplies corresponding elements of the two 2D lists, producing a new 2D array, product
, with the results.
It’s worth noting that, unlike the zip()
method, numpy.multiply()
handles 2D multiplication seamlessly without the need for explicit loops.
Output:
[[156 6 3]
[ 10 9 4]]
The numpy.multiply()
method simplifies the process of element-wise multiplication and enhances efficiency, especially in numerical computations.
Multiply Two Lists in Python Using the map()
Function
The map()
function offers a concise way to apply a specified function to each item of one or more iterable(s) and returns an iterable with the results. When used in conjunction with lambda functions, it becomes a powerful tool for performing operations on elements of lists, simplifying the syntax and promoting readability.
Syntax of the map()
function:
map(function, iterable, ...)
Here, function
is the function to apply to each item of the iterable(s), and iterable
is one or more iterables whose elements will be processed by the specified function.
Let’s see an example code for element-wise multiplication using the map()
function.
list1 = [2, 4, 5, 3, 5, 4]
list2 = [4, 1, 2, 9, 7, 5]
product = list(map(lambda x, y: x * y, list1, list2))
print(product)
In this example, the line list(map(lambda x, y: x * y, list1, list2))
efficiently applies a lambda function to each corresponding pair of elements from list1
and list2
.
Here, the lambda function, defined as lambda x, y: x * y
, multiplies the elements at the same position. The resulting list, product
, contains the products of the corresponding elements from the original lists.
Output:
[8, 4, 10, 27, 35, 20]
This result demonstrates the element-wise multiplication of list1
and list2
using the map()
function. Each element in the new list corresponds to the product of elements at the same position in the original lists.
Now, let’s do element-wise multiplication on two 2D lists using the map()
function.
list1 = [[2, 4, 5], [3, 5, 4]]
list2 = [[4, 1, 2], [9, 7, 5]]
product = list(
map(lambda row1, row2: list(map(lambda x, y: x * y, row1, row2)), list1, list2)
)
print(product)
As you can see in this code, the line list(map(lambda row1, row2: list(map(lambda x, y: x * y, row1, row2)), list1, list2))
employs nested map()
functions.
The outer map()
iterates over corresponding rows of list1
and list2
, and for each pair of rows, the inner map()
multiplies corresponding elements using the lambda function lambda x, y: x * y
.
The resulting product
list contains the multiplied values in a 2D structure.
Output:
[[8, 4, 10], [27, 35, 20]]
Multiply Two Lists in Python Using a Loop
In certain scenarios, a simple and traditional for
loop can be a preferred approach. This method, while straightforward, provides a clear understanding of the underlying logic.
When we use a loop, we iterate through each element in the lists, multiply the corresponding elements, and accumulate the results in a new list. This method is accessible and easy to understand, making it suitable for scenarios where simplicity is valued over conciseness.
Consider the following two lists, and let’s perform element-wise multiplication using a loop.
list1 = [2, 4, 5, 3, 5, 4]
list2 = [4, 1, 2, 9, 7, 5]
result = []
for i in range(len(list1)):
result.append(list1[i] * list2[i])
print(result)
In this code, the for
loop iterates through the indices of the lists using range(len(list1))
. At each iteration, it multiplies the elements at the same index from list1
and list2
and appends the result to the result
list.
After the loop completes, we print the result
list, which now contains the products of corresponding elements from the original lists.
Output:
[8, 4, 10, 27, 35, 20]
Now, let’s see how we can use a loop to multiply corresponding elements of two-dimensional lists.
list1 = [[2, 4, 5], [3, 5, 4]]
list2 = [[4, 1, 2], [9, 7, 5]]
result = []
for i in range(len(list1)):
row_result = []
for j in range(len(list1[i])):
row_result.append(list1[i][j] * list2[i][j])
result.append(row_result)
print(result)
In this 2D example, the outer for
loop iterates through the rows of the lists. Inside this loop, we initialize an empty list called row_result
.
The inner for
loop iterates through the elements of each row, multiply the corresponding elements, and appends the result to row_result
. After completing the inner loop for a row, we append row_result
to the result
list.
The final result is a new 2D list containing the products of corresponding elements from the original 2D lists.
Output:
[[8, 4, 10], [27, 35, 20]]
Multiply Two Lists in Python Using operator.mul()
The operator
module provides a set of efficient functions corresponding to the intrinsic operators of the language. One such function is operator.mul()
, which performs multiplication on two operands.
The operator.mul(a, b)
function is equivalent to the expression a * b
. It takes two arguments and returns their product.
By using this function, we can neatly express element-wise multiplication without the need for explicit loops or lambda functions.
Syntax of operator.mul()
:
operator.mul(a, b)
Here, a
and b
are the operands for multiplication. The function returns the product of a
and b
.
Consider two lists, list1
and list2
, and perform element-wise multiplication using operator.mul()
.
import operator
list1 = [2, 4, 5, 3, 5, 4]
list2 = [4, 1, 2, 9, 7, 5]
result = list(map(operator.mul, list1, list2))
print(result)
In this example, we start by importing the operator
module. We then declare two lists, list1
and list2
.
The line list(map(operator.mul, list1, list2))
utilizes the map()
function to apply the operator.mul()
function element-wise to corresponding pairs of elements from list1
and list2
.
As a result, we get a new list containing the products of corresponding elements from the original lists.
Output:
[8, 4, 10, 27, 35, 20]
Now, let’s explore how to apply this operator function for element-wise multiplication of corresponding elements in two-dimensional lists.
import operator
list1 = [[2, 4, 5], [3, 5, 4]]
list2 = [[4, 1, 2], [9, 7, 5]]
result = [list(map(operator.mul, row1, row2)) for row1, row2 in zip(list1, list2)]
print(result)
In this example, the line [list(map(operator.mul, row1, row2)) for row1, row2 in zip(list1, list2)]
uses a list comprehension to iterate through corresponding pairs of rows from list1
and list2
.
Within the list comprehension, the map()
function applies the operator.mul()
element-wise to each pair of elements from the rows. The result is a new 2D list, result
, containing the products of corresponding elements from the original 2D lists.
Output:
[[8, 4, 10], [27, 35, 20]]
Multiply Two Lists in Python Using a Recursive Method
In Python, the recursive approach offers an alternative method for performing element-wise multiplication of two lists.
The recursive method involves breaking down a problem into smaller sub-problems, solving them, and combining their solutions to derive the final result. For element-wise list multiplication, this means multiplying the first elements of the lists and recursively applying the same process to the remaining elements.
Let’s see an example:
def recursive_multiply(list1, list2, index=0, result=None):
# Base case
if index == len(list1):
return result
# Recursive case
current_product = list1[index] * list2[index]
result.append(current_product)
return recursive_multiply(list1, list2, index + 1, result)
list1 = [2, 4, 5, 3, 5, 4]
list2 = [4, 1, 2, 9, 7, 5]
result = recursive_multiply(list1, list2, result=[])
print(result)
Here, we define the recursive_multiply
function to handle the recursive multiplication of two lists. The base case checks if the current index is equal to the length of the lists, in which case the accumulated result is returned.
In the recursive case, the function calculates the product of the current elements, appends it to the result, and calls itself with the next index.
Output:
[8, 4, 10, 27, 35, 20]
We can also develop a recursive solution that multiplies the corresponding elements of two 2D lists. Let’s see how to implement this recursive method for element-wise multiplication.
def recursive_multiply_2d(list1, list2, row=0, col=0, result=None):
# Base case: reached the end of the rows
if row == len(list1):
return result
# Base case: reached the end of the columns in the current row
if col == len(list1[row]):
return recursive_multiply_2d(list1, list2, row + 1, 0, result)
# Recursive case
current_product = list1[row][col] * list2[row][col]
result[row].append(current_product)
return recursive_multiply_2d(list1, list2, row, col + 1, result)
list1 = [[2, 4, 5], [3, 5, 4]]
list2 = [[4, 1, 2], [9, 7, 5]]
result = recursive_multiply_2d(list1, list2, result=[[] for _ in range(len(list1))])
print(result)
In this example, the base cases check if we have reached the end of the rows or columns, in which case the function either returns the accumulated result (for the end of rows) or moves to the next row (for the end of columns).
In the recursive case, the function calculates the product of the current elements, appends it to the result, and calls itself with the next column.
Output:
[[8, 4, 10], [27, 35, 20]]
Conclusion
In Python, the ability to multiply lists in different ways underscores the language’s flexibility and richness. Each method discussed in this article offers a unique perspective on element-wise list multiplication, catering to various coding styles and scenarios.
Whether you opt for the clarity of loops, the conciseness of operator functions, the recursive elegance, or the functional programming approach, the goal remains the same – efficiently obtaining a list that captures the products of corresponding elements.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python